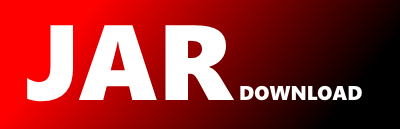
com.pulumi.googlenative.dataflow.v1b3.kotlin.inputs.TaskRunnerSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dataflow.v1b3.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.dataflow.v1b3.inputs.TaskRunnerSettingsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Taskrunner configuration settings.
* @property alsologtostderr Whether to also send taskrunner log info to stderr.
* @property baseTaskDir The location on the worker for task-specific subdirectories.
* @property baseUrl The base URL for the taskrunner to use when accessing Google Cloud APIs. When workers access Google Cloud APIs, they logically do so via relative URLs. If this field is specified, it supplies the base URL to use for resolving these relative URLs. The normative algorithm used is defined by RFC 1808, "Relative Uniform Resource Locators". If not specified, the default value is "http://www.googleapis.com/"
* @property commandlinesFileName The file to store preprocessing commands in.
* @property continueOnException Whether to continue taskrunner if an exception is hit.
* @property dataflowApiVersion The API version of endpoint, e.g. "v1b3"
* @property harnessCommand The command to launch the worker harness.
* @property languageHint The suggested backend language.
* @property logDir The directory on the VM to store logs.
* @property logToSerialconsole Whether to send taskrunner log info to Google Compute Engine VM serial console.
* @property logUploadLocation Indicates where to put logs. If this is not specified, the logs will not be uploaded. The supported resource type is: Google Cloud Storage: storage.googleapis.com/{bucket}/{object} bucket.storage.googleapis.com/{object}
* @property oauthScopes The OAuth2 scopes to be requested by the taskrunner in order to access the Cloud Dataflow API.
* @property parallelWorkerSettings The settings to pass to the parallel worker harness.
* @property streamingWorkerMainClass The streaming worker main class name.
* @property taskGroup The UNIX group ID on the worker VM to use for tasks launched by taskrunner; e.g. "wheel".
* @property taskUser The UNIX user ID on the worker VM to use for tasks launched by taskrunner; e.g. "root".
* @property tempStoragePrefix The prefix of the resources the taskrunner should use for temporary storage. The supported resource type is: Google Cloud Storage: storage.googleapis.com/{bucket}/{object} bucket.storage.googleapis.com/{object}
* @property vmId The ID string of the VM.
* @property workflowFileName The file to store the workflow in.
*/
public data class TaskRunnerSettingsArgs(
public val alsologtostderr: Output? = null,
public val baseTaskDir: Output? = null,
public val baseUrl: Output? = null,
public val commandlinesFileName: Output? = null,
public val continueOnException: Output? = null,
public val dataflowApiVersion: Output? = null,
public val harnessCommand: Output? = null,
public val languageHint: Output? = null,
public val logDir: Output? = null,
public val logToSerialconsole: Output? = null,
public val logUploadLocation: Output? = null,
public val oauthScopes: Output>? = null,
public val parallelWorkerSettings: Output? = null,
public val streamingWorkerMainClass: Output? = null,
public val taskGroup: Output? = null,
public val taskUser: Output? = null,
public val tempStoragePrefix: Output? = null,
public val vmId: Output? = null,
public val workflowFileName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.dataflow.v1b3.inputs.TaskRunnerSettingsArgs =
com.pulumi.googlenative.dataflow.v1b3.inputs.TaskRunnerSettingsArgs.builder()
.alsologtostderr(alsologtostderr?.applyValue({ args0 -> args0 }))
.baseTaskDir(baseTaskDir?.applyValue({ args0 -> args0 }))
.baseUrl(baseUrl?.applyValue({ args0 -> args0 }))
.commandlinesFileName(commandlinesFileName?.applyValue({ args0 -> args0 }))
.continueOnException(continueOnException?.applyValue({ args0 -> args0 }))
.dataflowApiVersion(dataflowApiVersion?.applyValue({ args0 -> args0 }))
.harnessCommand(harnessCommand?.applyValue({ args0 -> args0 }))
.languageHint(languageHint?.applyValue({ args0 -> args0 }))
.logDir(logDir?.applyValue({ args0 -> args0 }))
.logToSerialconsole(logToSerialconsole?.applyValue({ args0 -> args0 }))
.logUploadLocation(logUploadLocation?.applyValue({ args0 -> args0 }))
.oauthScopes(oauthScopes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.parallelWorkerSettings(
parallelWorkerSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.streamingWorkerMainClass(streamingWorkerMainClass?.applyValue({ args0 -> args0 }))
.taskGroup(taskGroup?.applyValue({ args0 -> args0 }))
.taskUser(taskUser?.applyValue({ args0 -> args0 }))
.tempStoragePrefix(tempStoragePrefix?.applyValue({ args0 -> args0 }))
.vmId(vmId?.applyValue({ args0 -> args0 }))
.workflowFileName(workflowFileName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TaskRunnerSettingsArgs].
*/
@PulumiTagMarker
public class TaskRunnerSettingsArgsBuilder internal constructor() {
private var alsologtostderr: Output? = null
private var baseTaskDir: Output? = null
private var baseUrl: Output? = null
private var commandlinesFileName: Output? = null
private var continueOnException: Output? = null
private var dataflowApiVersion: Output? = null
private var harnessCommand: Output? = null
private var languageHint: Output? = null
private var logDir: Output? = null
private var logToSerialconsole: Output? = null
private var logUploadLocation: Output? = null
private var oauthScopes: Output>? = null
private var parallelWorkerSettings: Output? = null
private var streamingWorkerMainClass: Output? = null
private var taskGroup: Output? = null
private var taskUser: Output? = null
private var tempStoragePrefix: Output? = null
private var vmId: Output? = null
private var workflowFileName: Output? = null
/**
* @param value Whether to also send taskrunner log info to stderr.
*/
@JvmName("iaumndpmetwvfolc")
public suspend fun alsologtostderr(`value`: Output) {
this.alsologtostderr = value
}
/**
* @param value The location on the worker for task-specific subdirectories.
*/
@JvmName("kdijiblmcnxbstra")
public suspend fun baseTaskDir(`value`: Output) {
this.baseTaskDir = value
}
/**
* @param value The base URL for the taskrunner to use when accessing Google Cloud APIs. When workers access Google Cloud APIs, they logically do so via relative URLs. If this field is specified, it supplies the base URL to use for resolving these relative URLs. The normative algorithm used is defined by RFC 1808, "Relative Uniform Resource Locators". If not specified, the default value is "http://www.googleapis.com/"
*/
@JvmName("yqfcxwijabkvslyk")
public suspend fun baseUrl(`value`: Output) {
this.baseUrl = value
}
/**
* @param value The file to store preprocessing commands in.
*/
@JvmName("pmbhtprvmjsgmjnk")
public suspend fun commandlinesFileName(`value`: Output) {
this.commandlinesFileName = value
}
/**
* @param value Whether to continue taskrunner if an exception is hit.
*/
@JvmName("mwxnpcogxeloosnv")
public suspend fun continueOnException(`value`: Output) {
this.continueOnException = value
}
/**
* @param value The API version of endpoint, e.g. "v1b3"
*/
@JvmName("pmqnrnryxavtdnmf")
public suspend fun dataflowApiVersion(`value`: Output) {
this.dataflowApiVersion = value
}
/**
* @param value The command to launch the worker harness.
*/
@JvmName("qehwgjqpjbokexst")
public suspend fun harnessCommand(`value`: Output) {
this.harnessCommand = value
}
/**
* @param value The suggested backend language.
*/
@JvmName("acawuhtsrrhpunmo")
public suspend fun languageHint(`value`: Output) {
this.languageHint = value
}
/**
* @param value The directory on the VM to store logs.
*/
@JvmName("vgbbdhnaytcajtpb")
public suspend fun logDir(`value`: Output) {
this.logDir = value
}
/**
* @param value Whether to send taskrunner log info to Google Compute Engine VM serial console.
*/
@JvmName("aevkprmqormjilho")
public suspend fun logToSerialconsole(`value`: Output) {
this.logToSerialconsole = value
}
/**
* @param value Indicates where to put logs. If this is not specified, the logs will not be uploaded. The supported resource type is: Google Cloud Storage: storage.googleapis.com/{bucket}/{object} bucket.storage.googleapis.com/{object}
*/
@JvmName("avetebuuwwbryyyp")
public suspend fun logUploadLocation(`value`: Output) {
this.logUploadLocation = value
}
/**
* @param value The OAuth2 scopes to be requested by the taskrunner in order to access the Cloud Dataflow API.
*/
@JvmName("kumbuvegagcgcyrj")
public suspend fun oauthScopes(`value`: Output>) {
this.oauthScopes = value
}
@JvmName("vsimhiioyglmtqpr")
public suspend fun oauthScopes(vararg values: Output) {
this.oauthScopes = Output.all(values.asList())
}
/**
* @param values The OAuth2 scopes to be requested by the taskrunner in order to access the Cloud Dataflow API.
*/
@JvmName("vsiwfrccetybqsgp")
public suspend fun oauthScopes(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy