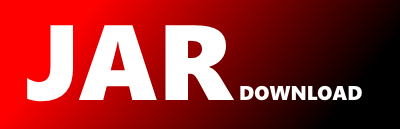
com.pulumi.googlenative.dataform.v1beta1.kotlin.ReleaseConfig.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dataform.v1beta1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.dataform.v1beta1.kotlin.outputs.CodeCompilationConfigResponse
import com.pulumi.googlenative.dataform.v1beta1.kotlin.outputs.ScheduledReleaseRecordResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.googlenative.dataform.v1beta1.kotlin.outputs.CodeCompilationConfigResponse.Companion.toKotlin as codeCompilationConfigResponseToKotlin
import com.pulumi.googlenative.dataform.v1beta1.kotlin.outputs.ScheduledReleaseRecordResponse.Companion.toKotlin as scheduledReleaseRecordResponseToKotlin
/**
* Builder for [ReleaseConfig].
*/
@PulumiTagMarker
public class ReleaseConfigResourceBuilder internal constructor() {
public var name: String? = null
public var args: ReleaseConfigArgs = ReleaseConfigArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ReleaseConfigArgsBuilder.() -> Unit) {
val builder = ReleaseConfigArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ReleaseConfig {
val builtJavaResource =
com.pulumi.googlenative.dataform.v1beta1.ReleaseConfig(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ReleaseConfig(builtJavaResource)
}
}
/**
* Creates a new ReleaseConfig in a given Repository.
* Auto-naming is currently not supported for this resource.
*/
public class ReleaseConfig internal constructor(
override val javaResource: com.pulumi.googlenative.dataform.v1beta1.ReleaseConfig,
) : KotlinCustomResource(javaResource, ReleaseConfigMapper) {
/**
* Optional. If set, fields of `code_compilation_config` override the default compilation settings that are specified in dataform.json.
*/
public val codeCompilationConfig: Output
get() = javaResource.codeCompilationConfig().applyValue({ args0 ->
args0.let({ args0 ->
codeCompilationConfigResponseToKotlin(args0)
})
})
/**
* Optional. Optional schedule (in cron format) for automatic creation of compilation results.
*/
public val cronSchedule: Output
get() = javaResource.cronSchedule().applyValue({ args0 -> args0 })
/**
* Git commit/tag/branch name at which the repository should be compiled. Must exist in the remote repository. Examples: - a commit SHA: `12ade345` - a tag: `tag1` - a branch name: `branch1`
*/
public val gitCommitish: Output
get() = javaResource.gitCommitish().applyValue({ args0 -> args0 })
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* The release config's name.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* Records of the 10 most recent scheduled release attempts, ordered in in descending order of `release_time`. Updated whenever automatic creation of a compilation result is triggered by cron_schedule.
*/
public val recentScheduledReleaseRecords: Output>
get() = javaResource.recentScheduledReleaseRecords().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> scheduledReleaseRecordResponseToKotlin(args0) })
})
})
/**
* Optional. The name of the currently released compilation result for this release config. This value is updated when a compilation result is created from this release config, or when this resource is updated by API call (perhaps to roll back to an earlier release). The compilation result must have been created using this release config. Must be in the format `projects/*/locations/*/repositories/*/compilationResults/*`.
* */*/*/*/
*/
public val releaseCompilationResult: Output
get() = javaResource.releaseCompilationResult().applyValue({ args0 -> args0 })
/**
* Required. The ID to use for the release config, which will become the final component of the release config's resource name.
*/
public val releaseConfigId: Output
get() = javaResource.releaseConfigId().applyValue({ args0 -> args0 })
public val repositoryId: Output
get() = javaResource.repositoryId().applyValue({ args0 -> args0 })
/**
* Optional. Specifies the time zone to be used when interpreting cron_schedule. Must be a time zone name from the time zone database (https://en.wikipedia.org/wiki/List_of_tz_database_time_zones). If left unspecified, the default is UTC.
*/
public val timeZone: Output
get() = javaResource.timeZone().applyValue({ args0 -> args0 })
}
public object ReleaseConfigMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.googlenative.dataform.v1beta1.ReleaseConfig::class == javaResource::class
override fun map(javaResource: Resource): ReleaseConfig = ReleaseConfig(
javaResource as
com.pulumi.googlenative.dataform.v1beta1.ReleaseConfig,
)
}
/**
* @see [ReleaseConfig].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [ReleaseConfig].
*/
public suspend fun releaseConfig(
name: String,
block: suspend ReleaseConfigResourceBuilder.() -> Unit,
): ReleaseConfig {
val builder = ReleaseConfigResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [ReleaseConfig].
* @param name The _unique_ name of the resulting resource.
*/
public fun releaseConfig(name: String): ReleaseConfig {
val builder = ReleaseConfigResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy