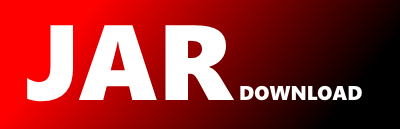
com.pulumi.googlenative.datalineage.v1.kotlin.Datalineage_v1Functions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.datalineage.v1.kotlin
import com.pulumi.googlenative.datalineage.v1.Datalineage_v1Functions.getLineageEventPlain
import com.pulumi.googlenative.datalineage.v1.Datalineage_v1Functions.getProcessPlain
import com.pulumi.googlenative.datalineage.v1.Datalineage_v1Functions.getRunPlain
import com.pulumi.googlenative.datalineage.v1.kotlin.inputs.GetLineageEventPlainArgs
import com.pulumi.googlenative.datalineage.v1.kotlin.inputs.GetLineageEventPlainArgsBuilder
import com.pulumi.googlenative.datalineage.v1.kotlin.inputs.GetProcessPlainArgs
import com.pulumi.googlenative.datalineage.v1.kotlin.inputs.GetProcessPlainArgsBuilder
import com.pulumi.googlenative.datalineage.v1.kotlin.inputs.GetRunPlainArgs
import com.pulumi.googlenative.datalineage.v1.kotlin.inputs.GetRunPlainArgsBuilder
import com.pulumi.googlenative.datalineage.v1.kotlin.outputs.GetLineageEventResult
import com.pulumi.googlenative.datalineage.v1.kotlin.outputs.GetProcessResult
import com.pulumi.googlenative.datalineage.v1.kotlin.outputs.GetRunResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.googlenative.datalineage.v1.kotlin.outputs.GetLineageEventResult.Companion.toKotlin as getLineageEventResultToKotlin
import com.pulumi.googlenative.datalineage.v1.kotlin.outputs.GetProcessResult.Companion.toKotlin as getProcessResultToKotlin
import com.pulumi.googlenative.datalineage.v1.kotlin.outputs.GetRunResult.Companion.toKotlin as getRunResultToKotlin
public object Datalineage_v1Functions {
/**
* Gets details of a specified lineage event.
* @param argument null
* @return null
*/
public suspend fun getLineageEvent(argument: GetLineageEventPlainArgs): GetLineageEventResult =
getLineageEventResultToKotlin(getLineageEventPlain(argument.toJava()).await())
/**
* @see [getLineageEvent].
* @param lineageEventId
* @param location
* @param processId
* @param project
* @param runId
* @return null
*/
public suspend fun getLineageEvent(
lineageEventId: String,
location: String,
processId: String,
project: String? = null,
runId: String,
): GetLineageEventResult {
val argument = GetLineageEventPlainArgs(
lineageEventId = lineageEventId,
location = location,
processId = processId,
project = project,
runId = runId,
)
return getLineageEventResultToKotlin(getLineageEventPlain(argument.toJava()).await())
}
/**
* @see [getLineageEvent].
* @param argument Builder for [com.pulumi.googlenative.datalineage.v1.kotlin.inputs.GetLineageEventPlainArgs].
* @return null
*/
public suspend fun getLineageEvent(argument: suspend GetLineageEventPlainArgsBuilder.() -> Unit): GetLineageEventResult {
val builder = GetLineageEventPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getLineageEventResultToKotlin(getLineageEventPlain(builtArgument.toJava()).await())
}
/**
* Gets the details of the specified process.
* @param argument null
* @return null
*/
public suspend fun getProcess(argument: GetProcessPlainArgs): GetProcessResult =
getProcessResultToKotlin(getProcessPlain(argument.toJava()).await())
/**
* @see [getProcess].
* @param location
* @param processId
* @param project
* @return null
*/
public suspend fun getProcess(
location: String,
processId: String,
project: String? = null,
): GetProcessResult {
val argument = GetProcessPlainArgs(
location = location,
processId = processId,
project = project,
)
return getProcessResultToKotlin(getProcessPlain(argument.toJava()).await())
}
/**
* @see [getProcess].
* @param argument Builder for [com.pulumi.googlenative.datalineage.v1.kotlin.inputs.GetProcessPlainArgs].
* @return null
*/
public suspend fun getProcess(argument: suspend GetProcessPlainArgsBuilder.() -> Unit): GetProcessResult {
val builder = GetProcessPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getProcessResultToKotlin(getProcessPlain(builtArgument.toJava()).await())
}
/**
* Gets the details of the specified run.
* @param argument null
* @return null
*/
public suspend fun getRun(argument: GetRunPlainArgs): GetRunResult =
getRunResultToKotlin(getRunPlain(argument.toJava()).await())
/**
* @see [getRun].
* @param location
* @param processId
* @param project
* @param runId
* @return null
*/
public suspend fun getRun(
location: String,
processId: String,
project: String? = null,
runId: String,
): GetRunResult {
val argument = GetRunPlainArgs(
location = location,
processId = processId,
project = project,
runId = runId,
)
return getRunResultToKotlin(getRunPlain(argument.toJava()).await())
}
/**
* @see [getRun].
* @param argument Builder for [com.pulumi.googlenative.datalineage.v1.kotlin.inputs.GetRunPlainArgs].
* @return null
*/
public suspend fun getRun(argument: suspend GetRunPlainArgsBuilder.() -> Unit): GetRunResult {
val builder = GetRunPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getRunResultToKotlin(getRunPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy