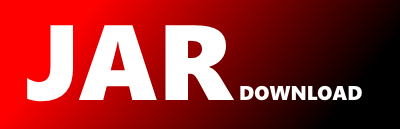
com.pulumi.googlenative.datamigration.v1.kotlin.inputs.OracleConnectionProfileArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.datamigration.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.datamigration.v1.inputs.OracleConnectionProfileArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Specifies connection parameters required specifically for Oracle databases.
* @property databaseService Database service for the Oracle connection.
* @property forwardSshConnectivity Forward SSH tunnel connectivity.
* @property host The IP or hostname of the source Oracle database.
* @property password Input only. The password for the user that Database Migration Service will be using to connect to the database. This field is not returned on request, and the value is encrypted when stored in Database Migration Service.
* @property port The network port of the source Oracle database.
* @property privateConnectivity Private connectivity.
* @property staticServiceIpConnectivity Static Service IP connectivity.
* @property username The username that Database Migration Service will use to connect to the database. The value is encrypted when stored in Database Migration Service.
*/
public data class OracleConnectionProfileArgs(
public val databaseService: Output,
public val forwardSshConnectivity: Output? = null,
public val host: Output,
public val password: Output,
public val port: Output,
public val privateConnectivity: Output? = null,
public val staticServiceIpConnectivity: Output? = null,
public val username: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.datamigration.v1.inputs.OracleConnectionProfileArgs = com.pulumi.googlenative.datamigration.v1.inputs.OracleConnectionProfileArgs.builder()
.databaseService(databaseService.applyValue({ args0 -> args0 }))
.forwardSshConnectivity(
forwardSshConnectivity?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.host(host.applyValue({ args0 -> args0 }))
.password(password.applyValue({ args0 -> args0 }))
.port(port.applyValue({ args0 -> args0 }))
.privateConnectivity(
privateConnectivity?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.staticServiceIpConnectivity(
staticServiceIpConnectivity?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.username(username.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [OracleConnectionProfileArgs].
*/
@PulumiTagMarker
public class OracleConnectionProfileArgsBuilder internal constructor() {
private var databaseService: Output? = null
private var forwardSshConnectivity: Output? = null
private var host: Output? = null
private var password: Output? = null
private var port: Output? = null
private var privateConnectivity: Output? = null
private var staticServiceIpConnectivity: Output? = null
private var username: Output? = null
/**
* @param value Database service for the Oracle connection.
*/
@JvmName("twoqjebyxhfnfgxm")
public suspend fun databaseService(`value`: Output) {
this.databaseService = value
}
/**
* @param value Forward SSH tunnel connectivity.
*/
@JvmName("iokkqtycpnpwpvpm")
public suspend fun forwardSshConnectivity(`value`: Output) {
this.forwardSshConnectivity = value
}
/**
* @param value The IP or hostname of the source Oracle database.
*/
@JvmName("qcpyhvkqvwvcufxd")
public suspend fun host(`value`: Output) {
this.host = value
}
/**
* @param value Input only. The password for the user that Database Migration Service will be using to connect to the database. This field is not returned on request, and the value is encrypted when stored in Database Migration Service.
*/
@JvmName("pgcfuyrfdseffdyw")
public suspend fun password(`value`: Output) {
this.password = value
}
/**
* @param value The network port of the source Oracle database.
*/
@JvmName("taeyqeqedhyhndkw")
public suspend fun port(`value`: Output) {
this.port = value
}
/**
* @param value Private connectivity.
*/
@JvmName("sbtayibstmpbdvqm")
public suspend fun privateConnectivity(`value`: Output) {
this.privateConnectivity = value
}
/**
* @param value Static Service IP connectivity.
*/
@JvmName("ifpflaynhmfcuosh")
public suspend fun staticServiceIpConnectivity(`value`: Output) {
this.staticServiceIpConnectivity = value
}
/**
* @param value The username that Database Migration Service will use to connect to the database. The value is encrypted when stored in Database Migration Service.
*/
@JvmName("sjagguyfddeduell")
public suspend fun username(`value`: Output) {
this.username = value
}
/**
* @param value Database service for the Oracle connection.
*/
@JvmName("risthrdaytrxxsip")
public suspend fun databaseService(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.databaseService = mapped
}
/**
* @param value Forward SSH tunnel connectivity.
*/
@JvmName("fxhkhaaevfreiyql")
public suspend fun forwardSshConnectivity(`value`: ForwardSshTunnelConnectivityArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.forwardSshConnectivity = mapped
}
/**
* @param argument Forward SSH tunnel connectivity.
*/
@JvmName("yukktamkoddqvote")
public suspend fun forwardSshConnectivity(argument: suspend ForwardSshTunnelConnectivityArgsBuilder.() -> Unit) {
val toBeMapped = ForwardSshTunnelConnectivityArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.forwardSshConnectivity = mapped
}
/**
* @param value The IP or hostname of the source Oracle database.
*/
@JvmName("xxywbseolmcdykrp")
public suspend fun host(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.host = mapped
}
/**
* @param value Input only. The password for the user that Database Migration Service will be using to connect to the database. This field is not returned on request, and the value is encrypted when stored in Database Migration Service.
*/
@JvmName("ioyppknlyhksncdm")
public suspend fun password(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.password = mapped
}
/**
* @param value The network port of the source Oracle database.
*/
@JvmName("oqnhbbhcqrghgjgg")
public suspend fun port(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.port = mapped
}
/**
* @param value Private connectivity.
*/
@JvmName("hqqaoxbsqcisyebq")
public suspend fun privateConnectivity(`value`: PrivateConnectivityArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateConnectivity = mapped
}
/**
* @param argument Private connectivity.
*/
@JvmName("ntdsihsmqxvxsyho")
public suspend fun privateConnectivity(argument: suspend PrivateConnectivityArgsBuilder.() -> Unit) {
val toBeMapped = PrivateConnectivityArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.privateConnectivity = mapped
}
/**
* @param value Static Service IP connectivity.
*/
@JvmName("fpdpttwntclekhwh")
public suspend fun staticServiceIpConnectivity(`value`: StaticServiceIpConnectivityArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.staticServiceIpConnectivity = mapped
}
/**
* @param argument Static Service IP connectivity.
*/
@JvmName("knsmwqylrhvgibyo")
public suspend fun staticServiceIpConnectivity(argument: suspend StaticServiceIpConnectivityArgsBuilder.() -> Unit) {
val toBeMapped = StaticServiceIpConnectivityArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.staticServiceIpConnectivity = mapped
}
/**
* @param value The username that Database Migration Service will use to connect to the database. The value is encrypted when stored in Database Migration Service.
*/
@JvmName("iowtkcqxomerqlta")
public suspend fun username(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.username = mapped
}
internal fun build(): OracleConnectionProfileArgs = OracleConnectionProfileArgs(
databaseService = databaseService ?: throw PulumiNullFieldException("databaseService"),
forwardSshConnectivity = forwardSshConnectivity,
host = host ?: throw PulumiNullFieldException("host"),
password = password ?: throw PulumiNullFieldException("password"),
port = port ?: throw PulumiNullFieldException("port"),
privateConnectivity = privateConnectivity,
staticServiceIpConnectivity = staticServiceIpConnectivity,
username = username ?: throw PulumiNullFieldException("username"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy