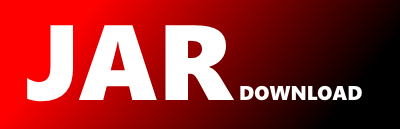
com.pulumi.googlenative.dataplex.v1.kotlin.DataTaxonomyArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dataplex.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.dataplex.v1.DataTaxonomyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Create a DataTaxonomy resource.
* Auto-naming is currently not supported for this resource.
* @property dataTaxonomyId Required. DataTaxonomy identifier. * Must contain only lowercase letters, numbers and hyphens. * Must start with a letter. * Must be between 1-63 characters. * Must end with a number or a letter. * Must be unique within the Project.
* @property description Optional. Description of the DataTaxonomy.
* @property displayName Optional. User friendly display name.
* @property etag This checksum is computed by the server based on the value of other fields, and may be sent on update and delete requests to ensure the client has an up-to-date value before proceeding.
* @property labels Optional. User-defined labels for the DataTaxonomy.
* @property location
* @property project
*/
public data class DataTaxonomyArgs(
public val dataTaxonomyId: Output? = null,
public val description: Output? = null,
public val displayName: Output? = null,
public val etag: Output? = null,
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy