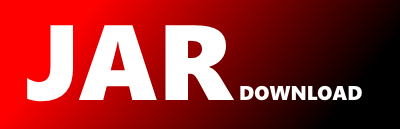
com.pulumi.googlenative.dataplex.v1.kotlin.ZoneArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dataplex.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.dataplex.v1.ZoneArgs.builder
import com.pulumi.googlenative.dataplex.v1.kotlin.enums.ZoneType
import com.pulumi.googlenative.dataplex.v1.kotlin.inputs.GoogleCloudDataplexV1ZoneDiscoverySpecArgs
import com.pulumi.googlenative.dataplex.v1.kotlin.inputs.GoogleCloudDataplexV1ZoneDiscoverySpecArgsBuilder
import com.pulumi.googlenative.dataplex.v1.kotlin.inputs.GoogleCloudDataplexV1ZoneResourceSpecArgs
import com.pulumi.googlenative.dataplex.v1.kotlin.inputs.GoogleCloudDataplexV1ZoneResourceSpecArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Creates a zone resource within a lake.
* Auto-naming is currently not supported for this resource.
* @property description Optional. Description of the zone.
* @property discoverySpec Optional. Specification of the discovery feature applied to data in this zone.
* @property displayName Optional. User friendly display name.
* @property labels Optional. User defined labels for the zone.
* @property lakeId
* @property location
* @property project
* @property resourceSpec Specification of the resources that are referenced by the assets within this zone.
* @property type Immutable. The type of the zone.
* @property zoneId Required. Zone identifier. This ID will be used to generate names such as database and dataset names when publishing metadata to Hive Metastore and BigQuery. * Must contain only lowercase letters, numbers and hyphens. * Must start with a letter. * Must end with a number or a letter. * Must be between 1-63 characters. * Must be unique across all lakes from all locations in a project. * Must not be one of the reserved IDs (i.e. "default", "global-temp")
*/
public data class ZoneArgs(
public val description: Output? = null,
public val discoverySpec: Output? = null,
public val displayName: Output? = null,
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy