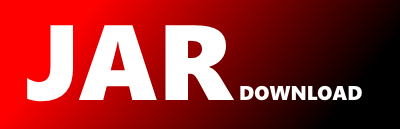
com.pulumi.googlenative.dataproc.v1.kotlin.outputs.GetBatchResult.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dataproc.v1.kotlin.outputs
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
*
* @property createTime The time when the batch was created.
* @property creator The email address of the user who created the batch.
* @property environmentConfig Optional. Environment configuration for the batch execution.
* @property labels Optional. The labels to associate with this batch. Label keys must contain 1 to 63 characters, and must conform to RFC 1035 (https://www.ietf.org/rfc/rfc1035.txt). Label values may be empty, but, if present, must contain 1 to 63 characters, and must conform to RFC 1035 (https://www.ietf.org/rfc/rfc1035.txt). No more than 32 labels can be associated with a batch.
* @property name The resource name of the batch.
* @property operation The resource name of the operation associated with this batch.
* @property pysparkBatch Optional. PySpark batch config.
* @property runtimeConfig Optional. Runtime configuration for the batch execution.
* @property runtimeInfo Runtime information about batch execution.
* @property sparkBatch Optional. Spark batch config.
* @property sparkRBatch Optional. SparkR batch config.
* @property sparkSqlBatch Optional. SparkSql batch config.
* @property state The state of the batch.
* @property stateHistory Historical state information for the batch.
* @property stateMessage Batch state details, such as a failure description if the state is FAILED.
* @property stateTime The time when the batch entered a current state.
* @property uuid A batch UUID (Unique Universal Identifier). The service generates this value when it creates the batch.
*/
public data class GetBatchResult(
public val createTime: String,
public val creator: String,
public val environmentConfig: EnvironmentConfigResponse,
public val labels: Map,
public val name: String,
public val operation: String,
public val pysparkBatch: PySparkBatchResponse,
public val runtimeConfig: RuntimeConfigResponse,
public val runtimeInfo: RuntimeInfoResponse,
public val sparkBatch: SparkBatchResponse,
public val sparkRBatch: SparkRBatchResponse,
public val sparkSqlBatch: SparkSqlBatchResponse,
public val state: String,
public val stateHistory: List,
public val stateMessage: String,
public val stateTime: String,
public val uuid: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.googlenative.dataproc.v1.outputs.GetBatchResult): GetBatchResult = GetBatchResult(
createTime = javaType.createTime(),
creator = javaType.creator(),
environmentConfig = javaType.environmentConfig().let({ args0 ->
com.pulumi.googlenative.dataproc.v1.kotlin.outputs.EnvironmentConfigResponse.Companion.toKotlin(args0)
}),
labels = javaType.labels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
name = javaType.name(),
operation = javaType.operation(),
pysparkBatch = javaType.pysparkBatch().let({ args0 ->
com.pulumi.googlenative.dataproc.v1.kotlin.outputs.PySparkBatchResponse.Companion.toKotlin(args0)
}),
runtimeConfig = javaType.runtimeConfig().let({ args0 ->
com.pulumi.googlenative.dataproc.v1.kotlin.outputs.RuntimeConfigResponse.Companion.toKotlin(args0)
}),
runtimeInfo = javaType.runtimeInfo().let({ args0 ->
com.pulumi.googlenative.dataproc.v1.kotlin.outputs.RuntimeInfoResponse.Companion.toKotlin(args0)
}),
sparkBatch = javaType.sparkBatch().let({ args0 ->
com.pulumi.googlenative.dataproc.v1.kotlin.outputs.SparkBatchResponse.Companion.toKotlin(args0)
}),
sparkRBatch = javaType.sparkRBatch().let({ args0 ->
com.pulumi.googlenative.dataproc.v1.kotlin.outputs.SparkRBatchResponse.Companion.toKotlin(args0)
}),
sparkSqlBatch = javaType.sparkSqlBatch().let({ args0 ->
com.pulumi.googlenative.dataproc.v1.kotlin.outputs.SparkSqlBatchResponse.Companion.toKotlin(args0)
}),
state = javaType.state(),
stateHistory = javaType.stateHistory().map({ args0 ->
args0.let({ args0 ->
com.pulumi.googlenative.dataproc.v1.kotlin.outputs.StateHistoryResponse.Companion.toKotlin(args0)
})
}),
stateMessage = javaType.stateMessage(),
stateTime = javaType.stateTime(),
uuid = javaType.uuid(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy