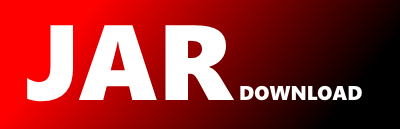
com.pulumi.googlenative.dataproc.v1beta2.kotlin.Cluster.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dataproc.v1beta2.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.dataproc.v1beta2.kotlin.outputs.ClusterConfigResponse
import com.pulumi.googlenative.dataproc.v1beta2.kotlin.outputs.ClusterMetricsResponse
import com.pulumi.googlenative.dataproc.v1beta2.kotlin.outputs.ClusterStatusResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.googlenative.dataproc.v1beta2.kotlin.outputs.ClusterConfigResponse.Companion.toKotlin as clusterConfigResponseToKotlin
import com.pulumi.googlenative.dataproc.v1beta2.kotlin.outputs.ClusterMetricsResponse.Companion.toKotlin as clusterMetricsResponseToKotlin
import com.pulumi.googlenative.dataproc.v1beta2.kotlin.outputs.ClusterStatusResponse.Companion.toKotlin as clusterStatusResponseToKotlin
/**
* Builder for [Cluster].
*/
@PulumiTagMarker
public class ClusterResourceBuilder internal constructor() {
public var name: String? = null
public var args: ClusterArgs = ClusterArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ClusterArgsBuilder.() -> Unit) {
val builder = ClusterArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Cluster {
val builtJavaResource =
com.pulumi.googlenative.dataproc.v1beta2.Cluster(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Cluster(builtJavaResource)
}
}
/**
* Creates a cluster in a project. The returned Operation.metadata will be ClusterOperationMetadata (https://cloud.google.com/dataproc/docs/reference/rpc/google.cloud.dataproc.v1beta2#clusteroperationmetadata).
* Auto-naming is currently not supported for this resource.
*/
public class Cluster internal constructor(
override val javaResource: com.pulumi.googlenative.dataproc.v1beta2.Cluster,
) : KotlinCustomResource(javaResource, ClusterMapper) {
/**
* The cluster name. Cluster names within a project must be unique. Names of deleted clusters can be reused.
*/
public val clusterName: Output
get() = javaResource.clusterName().applyValue({ args0 -> args0 })
/**
* A cluster UUID (Unique Universal Identifier). Dataproc generates this value when it creates the cluster.
*/
public val clusterUuid: Output
get() = javaResource.clusterUuid().applyValue({ args0 -> args0 })
/**
* The cluster config. Note that Dataproc may set default values, and values may change when clusters are updated.
*/
public val config: Output
get() = javaResource.config().applyValue({ args0 ->
args0.let({ args0 ->
clusterConfigResponseToKotlin(args0)
})
})
/**
* Optional. The labels to associate with this cluster. Label keys must contain 1 to 63 characters, and must conform to RFC 1035 (https://www.ietf.org/rfc/rfc1035.txt). Label values may be empty, but, if present, must contain 1 to 63 characters, and must conform to RFC 1035 (https://www.ietf.org/rfc/rfc1035.txt). No more than 32 labels can be associated with a cluster.
*/
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy