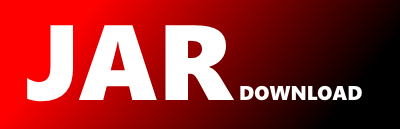
com.pulumi.googlenative.dialogflow.v2.kotlin.ConversationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dialogflow.v2.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.dialogflow.v2.ConversationArgs.builder
import com.pulumi.googlenative.dialogflow.v2.kotlin.enums.ConversationConversationStage
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Creates a new conversation. Conversations are auto-completed after 24 hours. Conversation Lifecycle: There are two stages during a conversation: Automated Agent Stage and Assist Stage. For Automated Agent Stage, there will be a dialogflow agent responding to user queries. For Assist Stage, there's no dialogflow agent responding to user queries. But we will provide suggestions which are generated from conversation. If Conversation.conversation_profile is configured for a dialogflow agent, conversation will start from `Automated Agent Stage`, otherwise, it will start from `Assist Stage`. And during `Automated Agent Stage`, once an Intent with Intent.live_agent_handoff is triggered, conversation will transfer to Assist Stage.
* Auto-naming is currently not supported for this resource.
* Note - this resource's API doesn't support deletion. When deleted, the resource will persist
* on Google Cloud even though it will be deleted from Pulumi state.
* @property conversationId Optional. Identifier of the conversation. Generally it's auto generated by Google. Only set it if you cannot wait for the response to return a auto-generated one to you. The conversation ID must be compliant with the regression fomula "a-zA-Z*" with the characters length in range of [3,64]. If the field is provided, the caller is resposible for 1. the uniqueness of the ID, otherwise the request will be rejected. 2. the consistency for whether to use custom ID or not under a project to better ensure uniqueness.
* @property conversationProfile The Conversation Profile to be used to configure this Conversation. This field cannot be updated. Format: `projects//locations//conversationProfiles/`.
* @property conversationStage The stage of a conversation. It indicates whether the virtual agent or a human agent is handling the conversation. If the conversation is created with the conversation profile that has Dialogflow config set, defaults to ConversationStage.VIRTUAL_AGENT_STAGE; Otherwise, defaults to ConversationStage.HUMAN_ASSIST_STAGE. If the conversation is created with the conversation profile that has Dialogflow config set but explicitly sets conversation_stage to ConversationStage.HUMAN_ASSIST_STAGE, it skips ConversationStage.VIRTUAL_AGENT_STAGE stage and directly goes to ConversationStage.HUMAN_ASSIST_STAGE.
* @property location
* @property project
*/
public data class ConversationArgs(
public val conversationId: Output? = null,
public val conversationProfile: Output? = null,
public val conversationStage: Output? = null,
public val location: Output? = null,
public val project: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.dialogflow.v2.ConversationArgs =
com.pulumi.googlenative.dialogflow.v2.ConversationArgs.builder()
.conversationId(conversationId?.applyValue({ args0 -> args0 }))
.conversationProfile(conversationProfile?.applyValue({ args0 -> args0 }))
.conversationStage(conversationStage?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.location(location?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ConversationArgs].
*/
@PulumiTagMarker
public class ConversationArgsBuilder internal constructor() {
private var conversationId: Output? = null
private var conversationProfile: Output? = null
private var conversationStage: Output? = null
private var location: Output? = null
private var project: Output? = null
/**
* @param value Optional. Identifier of the conversation. Generally it's auto generated by Google. Only set it if you cannot wait for the response to return a auto-generated one to you. The conversation ID must be compliant with the regression fomula "a-zA-Z*" with the characters length in range of [3,64]. If the field is provided, the caller is resposible for 1. the uniqueness of the ID, otherwise the request will be rejected. 2. the consistency for whether to use custom ID or not under a project to better ensure uniqueness.
*/
@JvmName("nnpjsoejvulgmsla")
public suspend fun conversationId(`value`: Output) {
this.conversationId = value
}
/**
* @param value The Conversation Profile to be used to configure this Conversation. This field cannot be updated. Format: `projects//locations//conversationProfiles/`.
*/
@JvmName("ceqwhorxqagvlrty")
public suspend fun conversationProfile(`value`: Output) {
this.conversationProfile = value
}
/**
* @param value The stage of a conversation. It indicates whether the virtual agent or a human agent is handling the conversation. If the conversation is created with the conversation profile that has Dialogflow config set, defaults to ConversationStage.VIRTUAL_AGENT_STAGE; Otherwise, defaults to ConversationStage.HUMAN_ASSIST_STAGE. If the conversation is created with the conversation profile that has Dialogflow config set but explicitly sets conversation_stage to ConversationStage.HUMAN_ASSIST_STAGE, it skips ConversationStage.VIRTUAL_AGENT_STAGE stage and directly goes to ConversationStage.HUMAN_ASSIST_STAGE.
*/
@JvmName("miowrxfwwrfrihlj")
public suspend fun conversationStage(`value`: Output) {
this.conversationStage = value
}
/**
* @param value
*/
@JvmName("mocsnqpsubblijpx")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value
*/
@JvmName("jevfhfdeoviummya")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value Optional. Identifier of the conversation. Generally it's auto generated by Google. Only set it if you cannot wait for the response to return a auto-generated one to you. The conversation ID must be compliant with the regression fomula "a-zA-Z*" with the characters length in range of [3,64]. If the field is provided, the caller is resposible for 1. the uniqueness of the ID, otherwise the request will be rejected. 2. the consistency for whether to use custom ID or not under a project to better ensure uniqueness.
*/
@JvmName("fnjvxbvxjkrqkrlr")
public suspend fun conversationId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.conversationId = mapped
}
/**
* @param value The Conversation Profile to be used to configure this Conversation. This field cannot be updated. Format: `projects//locations//conversationProfiles/`.
*/
@JvmName("fmdivrehhuqyyyrm")
public suspend fun conversationProfile(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.conversationProfile = mapped
}
/**
* @param value The stage of a conversation. It indicates whether the virtual agent or a human agent is handling the conversation. If the conversation is created with the conversation profile that has Dialogflow config set, defaults to ConversationStage.VIRTUAL_AGENT_STAGE; Otherwise, defaults to ConversationStage.HUMAN_ASSIST_STAGE. If the conversation is created with the conversation profile that has Dialogflow config set but explicitly sets conversation_stage to ConversationStage.HUMAN_ASSIST_STAGE, it skips ConversationStage.VIRTUAL_AGENT_STAGE stage and directly goes to ConversationStage.HUMAN_ASSIST_STAGE.
*/
@JvmName("penjkjvjsbrbctmn")
public suspend fun conversationStage(`value`: ConversationConversationStage?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.conversationStage = mapped
}
/**
* @param value
*/
@JvmName("dpplsfpxrhsytvnk")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value
*/
@JvmName("aalgtyoggcdenwuu")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
internal fun build(): ConversationArgs = ConversationArgs(
conversationId = conversationId,
conversationProfile = conversationProfile,
conversationStage = conversationStage,
location = location,
project = project,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy