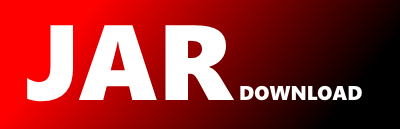
com.pulumi.googlenative.dialogflow.v2beta1.kotlin.inputs.GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dialogflow.v2beta1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Rich Business Messaging (RBM) suggested client-side action that the user can choose from the card.
* @property dial Suggested client side action: Dial a phone number
* @property openUrl Suggested client side action: Open a URI on device
* @property postbackData Opaque payload that the Dialogflow receives in a user event when the user taps the suggested action. This data will be also forwarded to webhook to allow performing custom business logic.
* @property shareLocation Suggested client side action: Share user location
* @property text Text to display alongside the action.
*/
public data class GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionArgs(
public val dial: Output? =
null,
public val openUrl: Output? =
null,
public val postbackData: Output? = null,
public val shareLocation: Output? =
null,
public val text: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.dialogflow.v2beta1.inputs.GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionArgs =
com.pulumi.googlenative.dialogflow.v2beta1.inputs.GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionArgs.builder()
.dial(dial?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.openUrl(openUrl?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.postbackData(postbackData?.applyValue({ args0 -> args0 }))
.shareLocation(shareLocation?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.text(text?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionArgs].
*/
@PulumiTagMarker
public class GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionArgsBuilder internal constructor() {
private var dial:
Output? =
null
private var openUrl:
Output? =
null
private var postbackData: Output? = null
private var shareLocation:
Output? =
null
private var text: Output? = null
/**
* @param value Suggested client side action: Dial a phone number
*/
@JvmName("ijwgdecssjtqatur")
public suspend fun dial(`value`: Output) {
this.dial = value
}
/**
* @param value Suggested client side action: Open a URI on device
*/
@JvmName("nucabdrxntwvlepu")
public suspend fun openUrl(`value`: Output) {
this.openUrl = value
}
/**
* @param value Opaque payload that the Dialogflow receives in a user event when the user taps the suggested action. This data will be also forwarded to webhook to allow performing custom business logic.
*/
@JvmName("ejlogijwioruayse")
public suspend fun postbackData(`value`: Output) {
this.postbackData = value
}
/**
* @param value Suggested client side action: Share user location
*/
@JvmName("kpgekvsusrnqckok")
public suspend fun shareLocation(`value`: Output) {
this.shareLocation = value
}
/**
* @param value Text to display alongside the action.
*/
@JvmName("ysommanklqwutgdw")
public suspend fun text(`value`: Output) {
this.text = value
}
/**
* @param value Suggested client side action: Dial a phone number
*/
@JvmName("dpddvtumfmiiypep")
public suspend fun dial(`value`: GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionRbmSuggestedActionDialArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dial = mapped
}
/**
* @param argument Suggested client side action: Dial a phone number
*/
@JvmName("owfrdxbgvtmyeyku")
public suspend fun dial(argument: suspend GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionRbmSuggestedActionDialArgsBuilder.() -> Unit) {
val toBeMapped =
GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionRbmSuggestedActionDialArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.dial = mapped
}
/**
* @param value Suggested client side action: Open a URI on device
*/
@JvmName("njmvadlyptqcdvir")
public suspend fun openUrl(`value`: GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionRbmSuggestedActionOpenUriArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.openUrl = mapped
}
/**
* @param argument Suggested client side action: Open a URI on device
*/
@JvmName("ypdsuihquvoemnfs")
public suspend fun openUrl(argument: suspend GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionRbmSuggestedActionOpenUriArgsBuilder.() -> Unit) {
val toBeMapped =
GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionRbmSuggestedActionOpenUriArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.openUrl = mapped
}
/**
* @param value Opaque payload that the Dialogflow receives in a user event when the user taps the suggested action. This data will be also forwarded to webhook to allow performing custom business logic.
*/
@JvmName("iwgameclwmjnpspe")
public suspend fun postbackData(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.postbackData = mapped
}
/**
* @param value Suggested client side action: Share user location
*/
@JvmName("hcvgesgteeclwftt")
public suspend fun shareLocation(`value`: GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionRbmSuggestedActionShareLocationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.shareLocation = mapped
}
/**
* @param argument Suggested client side action: Share user location
*/
@JvmName("cnqpyrocrmosljwf")
public suspend fun shareLocation(argument: suspend GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionRbmSuggestedActionShareLocationArgsBuilder.() -> Unit) {
val toBeMapped =
GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionRbmSuggestedActionShareLocationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.shareLocation = mapped
}
/**
* @param value Text to display alongside the action.
*/
@JvmName("tfioivssaptikhsj")
public suspend fun text(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.text = mapped
}
internal fun build(): GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionArgs =
GoogleCloudDialogflowV2beta1IntentMessageRbmSuggestedActionArgs(
dial = dial,
openUrl = openUrl,
postbackData = postbackData,
shareLocation = shareLocation,
text = text,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy