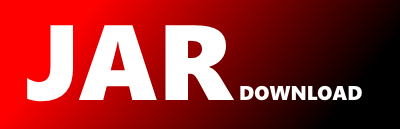
com.pulumi.googlenative.dialogflow.v2beta1.kotlin.inputs.GoogleCloudDialogflowV2beta1IntentTrainingPhrasePartArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dialogflow.v2beta1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GoogleCloudDialogflowV2beta1IntentTrainingPhrasePartArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Represents a part of a training phrase.
* @property alias Optional. The parameter name for the value extracted from the annotated part of the example. This field is required for annotated parts of the training phrase.
* @property entityType Optional. The entity type name prefixed with `@`. This field is required for annotated parts of the training phrase.
* @property text The text for this part.
* @property userDefined Optional. Indicates whether the text was manually annotated. This field is set to true when the Dialogflow Console is used to manually annotate the part. When creating an annotated part with the API, you must set this to true.
*/
public data class GoogleCloudDialogflowV2beta1IntentTrainingPhrasePartArgs(
public val alias: Output? = null,
public val entityType: Output? = null,
public val text: Output,
public val userDefined: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.dialogflow.v2beta1.inputs.GoogleCloudDialogflowV2beta1IntentTrainingPhrasePartArgs =
com.pulumi.googlenative.dialogflow.v2beta1.inputs.GoogleCloudDialogflowV2beta1IntentTrainingPhrasePartArgs.builder()
.alias(alias?.applyValue({ args0 -> args0 }))
.entityType(entityType?.applyValue({ args0 -> args0 }))
.text(text.applyValue({ args0 -> args0 }))
.userDefined(userDefined?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [GoogleCloudDialogflowV2beta1IntentTrainingPhrasePartArgs].
*/
@PulumiTagMarker
public class GoogleCloudDialogflowV2beta1IntentTrainingPhrasePartArgsBuilder internal constructor() {
private var alias: Output? = null
private var entityType: Output? = null
private var text: Output? = null
private var userDefined: Output? = null
/**
* @param value Optional. The parameter name for the value extracted from the annotated part of the example. This field is required for annotated parts of the training phrase.
*/
@JvmName("xiedidhjxmohejru")
public suspend fun alias(`value`: Output) {
this.alias = value
}
/**
* @param value Optional. The entity type name prefixed with `@`. This field is required for annotated parts of the training phrase.
*/
@JvmName("cewnwiqrnpresmyd")
public suspend fun entityType(`value`: Output) {
this.entityType = value
}
/**
* @param value The text for this part.
*/
@JvmName("ahpqamnmekswhdrq")
public suspend fun text(`value`: Output) {
this.text = value
}
/**
* @param value Optional. Indicates whether the text was manually annotated. This field is set to true when the Dialogflow Console is used to manually annotate the part. When creating an annotated part with the API, you must set this to true.
*/
@JvmName("wwmnbyxyxcdhfbvk")
public suspend fun userDefined(`value`: Output) {
this.userDefined = value
}
/**
* @param value Optional. The parameter name for the value extracted from the annotated part of the example. This field is required for annotated parts of the training phrase.
*/
@JvmName("ettwbcvwqousgrsb")
public suspend fun alias(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alias = mapped
}
/**
* @param value Optional. The entity type name prefixed with `@`. This field is required for annotated parts of the training phrase.
*/
@JvmName("ogpklbihfixjwpsr")
public suspend fun entityType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.entityType = mapped
}
/**
* @param value The text for this part.
*/
@JvmName("mbbxdgclrqlvlaho")
public suspend fun text(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.text = mapped
}
/**
* @param value Optional. Indicates whether the text was manually annotated. This field is set to true when the Dialogflow Console is used to manually annotate the part. When creating an annotated part with the API, you must set this to true.
*/
@JvmName("yrrtfdxpgedonwdb")
public suspend fun userDefined(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.userDefined = mapped
}
internal fun build(): GoogleCloudDialogflowV2beta1IntentTrainingPhrasePartArgs =
GoogleCloudDialogflowV2beta1IntentTrainingPhrasePartArgs(
alias = alias,
entityType = entityType,
text = text ?: throw PulumiNullFieldException("text"),
userDefined = userDefined,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy