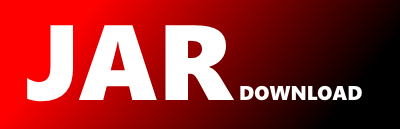
com.pulumi.googlenative.dialogflow.v3.kotlin.Flow.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dialogflow.v3.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.dialogflow.v3.kotlin.outputs.GoogleCloudDialogflowCxV3EventHandlerResponse
import com.pulumi.googlenative.dialogflow.v3.kotlin.outputs.GoogleCloudDialogflowCxV3NluSettingsResponse
import com.pulumi.googlenative.dialogflow.v3.kotlin.outputs.GoogleCloudDialogflowCxV3TransitionRouteResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.googlenative.dialogflow.v3.kotlin.outputs.GoogleCloudDialogflowCxV3EventHandlerResponse.Companion.toKotlin as googleCloudDialogflowCxV3EventHandlerResponseToKotlin
import com.pulumi.googlenative.dialogflow.v3.kotlin.outputs.GoogleCloudDialogflowCxV3NluSettingsResponse.Companion.toKotlin as googleCloudDialogflowCxV3NluSettingsResponseToKotlin
import com.pulumi.googlenative.dialogflow.v3.kotlin.outputs.GoogleCloudDialogflowCxV3TransitionRouteResponse.Companion.toKotlin as googleCloudDialogflowCxV3TransitionRouteResponseToKotlin
/**
* Builder for [Flow].
*/
@PulumiTagMarker
public class FlowResourceBuilder internal constructor() {
public var name: String? = null
public var args: FlowArgs = FlowArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend FlowArgsBuilder.() -> Unit) {
val builder = FlowArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Flow {
val builtJavaResource = com.pulumi.googlenative.dialogflow.v3.Flow(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Flow(builtJavaResource)
}
}
/**
* Creates a flow in the specified agent. Note: You should always train a flow prior to sending it queries. See the [training documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*/
public class Flow internal constructor(
override val javaResource: com.pulumi.googlenative.dialogflow.v3.Flow,
) : KotlinCustomResource(javaResource, FlowMapper) {
public val agentId: Output
get() = javaResource.agentId().applyValue({ args0 -> args0 })
/**
* The description of the flow. The maximum length is 500 characters. If exceeded, the request is rejected.
*/
public val description: Output
get() = javaResource.description().applyValue({ args0 -> args0 })
/**
* The human-readable name of the flow.
*/
public val displayName: Output
get() = javaResource.displayName().applyValue({ args0 -> args0 })
/**
* A flow's event handlers serve two purposes: * They are responsible for handling events (e.g. no match, webhook errors) in the flow. * They are inherited by every page's event handlers, which can be used to handle common events regardless of the current page. Event handlers defined in the page have higher priority than those defined in the flow. Unlike transition_routes, these handlers are evaluated on a first-match basis. The first one that matches the event get executed, with the rest being ignored.
*/
public val eventHandlers: Output>
get() = javaResource.eventHandlers().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> googleCloudDialogflowCxV3EventHandlerResponseToKotlin(args0) })
})
})
/**
* The language of the following fields in `flow`: * `Flow.event_handlers.trigger_fulfillment.messages` * `Flow.event_handlers.trigger_fulfillment.conditional_cases` * `Flow.transition_routes.trigger_fulfillment.messages` * `Flow.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the agent's default language is used. [Many languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note: languages must be enabled in the agent before they can be used.
*/
public val languageCode: Output?
get() = javaResource.languageCode().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* The unique identifier of the flow. Format: `projects//locations//agents//flows/`.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* NLU related settings of the flow.
*/
public val nluSettings: Output
get() = javaResource.nluSettings().applyValue({ args0 ->
args0.let({ args0 ->
googleCloudDialogflowCxV3NluSettingsResponseToKotlin(args0)
})
})
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* A flow's transition route group serve two purposes: * They are responsible for matching the user's first utterances in the flow. * They are inherited by every page's transition route groups. Transition route groups defined in the page have higher priority than those defined in the flow. Format:`projects//locations//agents//flows//transitionRouteGroups/`.
*/
public val transitionRouteGroups: Output>
get() = javaResource.transitionRouteGroups().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* A flow's transition routes serve two purposes: * They are responsible for matching the user's first utterances in the flow. * They are inherited by every page's transition routes and can support use cases such as the user saying "help" or "can I talk to a human?", which can be handled in a common way regardless of the current page. Transition routes defined in the page have higher priority than those defined in the flow. TransitionRoutes are evalauted in the following order: * TransitionRoutes with intent specified. * TransitionRoutes with only condition specified. TransitionRoutes with intent specified are inherited by pages in the flow.
*/
public val transitionRoutes: Output>
get() = javaResource.transitionRoutes().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> googleCloudDialogflowCxV3TransitionRouteResponseToKotlin(args0) })
})
})
}
public object FlowMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.googlenative.dialogflow.v3.Flow::class == javaResource::class
override fun map(javaResource: Resource): Flow = Flow(
javaResource as
com.pulumi.googlenative.dialogflow.v3.Flow,
)
}
/**
* @see [Flow].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Flow].
*/
public suspend fun flow(name: String, block: suspend FlowResourceBuilder.() -> Unit): Flow {
val builder = FlowResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Flow].
* @param name The _unique_ name of the resulting resource.
*/
public fun flow(name: String): Flow {
val builder = FlowResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy