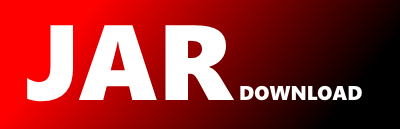
com.pulumi.googlenative.dialogflow.v3beta1.kotlin.inputs.GoogleCloudDialogflowCxV3beta1WebhookGenericWebServiceArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dialogflow.v3beta1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.dialogflow.v3beta1.inputs.GoogleCloudDialogflowCxV3beta1WebhookGenericWebServiceArgs.builder
import com.pulumi.googlenative.dialogflow.v3beta1.kotlin.enums.GoogleCloudDialogflowCxV3beta1WebhookGenericWebServiceHttpMethod
import com.pulumi.googlenative.dialogflow.v3beta1.kotlin.enums.GoogleCloudDialogflowCxV3beta1WebhookGenericWebServiceWebhookType
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Represents configuration for a generic web service.
* @property allowedCaCerts Optional. Specifies a list of allowed custom CA certificates (in DER format) for HTTPS verification. This overrides the default SSL trust store. If this is empty or unspecified, Dialogflow will use Google's default trust store to verify certificates. N.B. Make sure the HTTPS server certificates are signed with "subject alt name". For instance a certificate can be self-signed using the following command, ``` openssl x509 -req -days 200 -in example.com.csr \ -signkey example.com.key \ -out example.com.crt \ -extfile <(printf "\nsubjectAltName='DNS:www.example.com'") ```
* @property httpMethod Optional. HTTP method for the flexible webhook calls. Standard webhook always uses POST.
* @property parameterMapping Optional. Maps the values extracted from specific fields of the flexible webhook response into session parameters. - Key: session parameter name - Value: field path in the webhook response
* @property password The password for HTTP Basic authentication.
* @property requestBody Optional. Defines a custom JSON object as request body to send to flexible webhook.
* @property requestHeaders The HTTP request headers to send together with webhook requests.
* @property uri The webhook URI for receiving POST requests. It must use https protocol.
* @property username The user name for HTTP Basic authentication.
* @property webhookType Optional. Type of the webhook.
*/
public data class GoogleCloudDialogflowCxV3beta1WebhookGenericWebServiceArgs(
public val allowedCaCerts: Output>? = null,
public val httpMethod: Output? =
null,
public val parameterMapping: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy