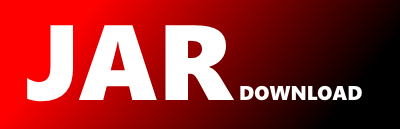
com.pulumi.googlenative.dlp.v2.kotlin.InspectTemplateArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dlp.v2.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.dlp.v2.InspectTemplateArgs.builder
import com.pulumi.googlenative.dlp.v2.kotlin.inputs.GooglePrivacyDlpV2InspectConfigArgs
import com.pulumi.googlenative.dlp.v2.kotlin.inputs.GooglePrivacyDlpV2InspectConfigArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Creates an InspectTemplate for reusing frequently used configuration for inspecting content, images, and storage. See https://cloud.google.com/dlp/docs/creating-templates to learn more.
* Auto-naming is currently not supported for this resource.
* @property description Short description (max 256 chars).
* @property displayName Display name (max 256 chars).
* @property inspectConfig The core content of the template. Configuration of the scanning process.
* @property location Deprecated. This field has no effect.
* @property project
* @property templateId The template id can contain uppercase and lowercase letters, numbers, and hyphens; that is, it must match the regular expression: `[a-zA-Z\d-_]+`. The maximum length is 100 characters. Can be empty to allow the system to generate one.
*/
public data class InspectTemplateArgs(
public val description: Output? = null,
public val displayName: Output? = null,
public val inspectConfig: Output? = null,
@Deprecated(
message = """
Deprecated. This field has no effect.
""",
)
public val location: Output? = null,
public val project: Output? = null,
public val templateId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.dlp.v2.InspectTemplateArgs =
com.pulumi.googlenative.dlp.v2.InspectTemplateArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.inspectConfig(inspectConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.location(location?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.templateId(templateId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [InspectTemplateArgs].
*/
@PulumiTagMarker
public class InspectTemplateArgsBuilder internal constructor() {
private var description: Output? = null
private var displayName: Output? = null
private var inspectConfig: Output? = null
private var location: Output? = null
private var project: Output? = null
private var templateId: Output? = null
/**
* @param value Short description (max 256 chars).
*/
@JvmName("gcddplexbpfhilrs")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Display name (max 256 chars).
*/
@JvmName("hederfwltatxnjyk")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value The core content of the template. Configuration of the scanning process.
*/
@JvmName("lsjbveflekmfiepa")
public suspend fun inspectConfig(`value`: Output) {
this.inspectConfig = value
}
/**
* @param value Deprecated. This field has no effect.
*/
@Deprecated(
message = """
Deprecated. This field has no effect.
""",
)
@JvmName("dxghscoormyrhmnx")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value
*/
@JvmName("hhooyrncqrdxapjo")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value The template id can contain uppercase and lowercase letters, numbers, and hyphens; that is, it must match the regular expression: `[a-zA-Z\d-_]+`. The maximum length is 100 characters. Can be empty to allow the system to generate one.
*/
@JvmName("ojshvfefuvneptpa")
public suspend fun templateId(`value`: Output) {
this.templateId = value
}
/**
* @param value Short description (max 256 chars).
*/
@JvmName("qxymfirntotdeqkd")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value Display name (max 256 chars).
*/
@JvmName("ctiwfwhdeorcuyha")
public suspend fun displayName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.displayName = mapped
}
/**
* @param value The core content of the template. Configuration of the scanning process.
*/
@JvmName("castgddvgmytfhbg")
public suspend fun inspectConfig(`value`: GooglePrivacyDlpV2InspectConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.inspectConfig = mapped
}
/**
* @param argument The core content of the template. Configuration of the scanning process.
*/
@JvmName("ljubyvraysywaweu")
public suspend fun inspectConfig(argument: suspend GooglePrivacyDlpV2InspectConfigArgsBuilder.() -> Unit) {
val toBeMapped = GooglePrivacyDlpV2InspectConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.inspectConfig = mapped
}
/**
* @param value Deprecated. This field has no effect.
*/
@Deprecated(
message = """
Deprecated. This field has no effect.
""",
)
@JvmName("xpgnfcoqbwegxtri")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value
*/
@JvmName("qnsuxntenpydevgt")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value The template id can contain uppercase and lowercase letters, numbers, and hyphens; that is, it must match the regular expression: `[a-zA-Z\d-_]+`. The maximum length is 100 characters. Can be empty to allow the system to generate one.
*/
@JvmName("gnwfprlbtggmdubh")
public suspend fun templateId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.templateId = mapped
}
internal fun build(): InspectTemplateArgs = InspectTemplateArgs(
description = description,
displayName = displayName,
inspectConfig = inspectConfig,
location = location,
project = project,
templateId = templateId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy