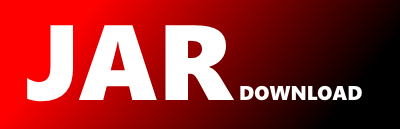
com.pulumi.googlenative.dns.v1.kotlin.ChangeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dns.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.dns.v1.ChangeArgs.builder
import com.pulumi.googlenative.dns.v1.kotlin.inputs.ResourceRecordSetArgs
import com.pulumi.googlenative.dns.v1.kotlin.inputs.ResourceRecordSetArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Atomically updates the ResourceRecordSet collection.
* Auto-naming is currently not supported for this resource.
* Note - this resource's API doesn't support deletion. When deleted, the resource will persist
* on Google Cloud even though it will be deleted from Pulumi state.
* @property additions Which ResourceRecordSets to add?
* @property clientOperationId For mutating operation requests only. An optional identifier specified by the client. Must be unique for operation resources in the Operations collection.
* @property deletions Which ResourceRecordSets to remove? Must match existing data exactly.
* @property isServing If the DNS queries for the zone will be served.
* @property kind
* @property managedZone
* @property project
*/
public data class ChangeArgs(
public val additions: Output>? = null,
public val clientOperationId: Output? = null,
public val deletions: Output>? = null,
public val isServing: Output? = null,
public val kind: Output? = null,
public val managedZone: Output? = null,
public val project: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.dns.v1.ChangeArgs =
com.pulumi.googlenative.dns.v1.ChangeArgs.builder()
.additions(
additions?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.clientOperationId(clientOperationId?.applyValue({ args0 -> args0 }))
.deletions(
deletions?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.isServing(isServing?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 }))
.managedZone(managedZone?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ChangeArgs].
*/
@PulumiTagMarker
public class ChangeArgsBuilder internal constructor() {
private var additions: Output>? = null
private var clientOperationId: Output? = null
private var deletions: Output>? = null
private var isServing: Output? = null
private var kind: Output? = null
private var managedZone: Output? = null
private var project: Output? = null
/**
* @param value Which ResourceRecordSets to add?
*/
@JvmName("khrbufgyuofpuwar")
public suspend fun additions(`value`: Output>) {
this.additions = value
}
@JvmName("yoywkpxqplrnoqdt")
public suspend fun additions(vararg values: Output) {
this.additions = Output.all(values.asList())
}
/**
* @param values Which ResourceRecordSets to add?
*/
@JvmName("mgennbarvujeaakk")
public suspend fun additions(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy