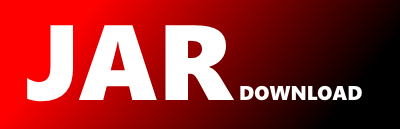
com.pulumi.googlenative.dns.v1beta2.kotlin.Dns_v1beta2Functions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dns.v1beta2.kotlin
import com.pulumi.googlenative.dns.v1beta2.Dns_v1beta2Functions.getChangePlain
import com.pulumi.googlenative.dns.v1beta2.Dns_v1beta2Functions.getManagedZoneIamPolicyPlain
import com.pulumi.googlenative.dns.v1beta2.Dns_v1beta2Functions.getManagedZonePlain
import com.pulumi.googlenative.dns.v1beta2.Dns_v1beta2Functions.getPolicyPlain
import com.pulumi.googlenative.dns.v1beta2.Dns_v1beta2Functions.getResourceRecordSetPlain
import com.pulumi.googlenative.dns.v1beta2.Dns_v1beta2Functions.getResponsePolicyPlain
import com.pulumi.googlenative.dns.v1beta2.Dns_v1beta2Functions.getResponsePolicyRulePlain
import com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetChangePlainArgs
import com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetChangePlainArgsBuilder
import com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetManagedZoneIamPolicyPlainArgs
import com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetManagedZoneIamPolicyPlainArgsBuilder
import com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetManagedZonePlainArgs
import com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetManagedZonePlainArgsBuilder
import com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetPolicyPlainArgs
import com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetPolicyPlainArgsBuilder
import com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetResourceRecordSetPlainArgs
import com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetResourceRecordSetPlainArgsBuilder
import com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetResponsePolicyPlainArgs
import com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetResponsePolicyPlainArgsBuilder
import com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetResponsePolicyRulePlainArgs
import com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetResponsePolicyRulePlainArgsBuilder
import com.pulumi.googlenative.dns.v1beta2.kotlin.outputs.GetChangeResult
import com.pulumi.googlenative.dns.v1beta2.kotlin.outputs.GetManagedZoneIamPolicyResult
import com.pulumi.googlenative.dns.v1beta2.kotlin.outputs.GetManagedZoneResult
import com.pulumi.googlenative.dns.v1beta2.kotlin.outputs.GetPolicyResult
import com.pulumi.googlenative.dns.v1beta2.kotlin.outputs.GetResourceRecordSetResult
import com.pulumi.googlenative.dns.v1beta2.kotlin.outputs.GetResponsePolicyResult
import com.pulumi.googlenative.dns.v1beta2.kotlin.outputs.GetResponsePolicyRuleResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.googlenative.dns.v1beta2.kotlin.outputs.GetChangeResult.Companion.toKotlin as getChangeResultToKotlin
import com.pulumi.googlenative.dns.v1beta2.kotlin.outputs.GetManagedZoneIamPolicyResult.Companion.toKotlin as getManagedZoneIamPolicyResultToKotlin
import com.pulumi.googlenative.dns.v1beta2.kotlin.outputs.GetManagedZoneResult.Companion.toKotlin as getManagedZoneResultToKotlin
import com.pulumi.googlenative.dns.v1beta2.kotlin.outputs.GetPolicyResult.Companion.toKotlin as getPolicyResultToKotlin
import com.pulumi.googlenative.dns.v1beta2.kotlin.outputs.GetResourceRecordSetResult.Companion.toKotlin as getResourceRecordSetResultToKotlin
import com.pulumi.googlenative.dns.v1beta2.kotlin.outputs.GetResponsePolicyResult.Companion.toKotlin as getResponsePolicyResultToKotlin
import com.pulumi.googlenative.dns.v1beta2.kotlin.outputs.GetResponsePolicyRuleResult.Companion.toKotlin as getResponsePolicyRuleResultToKotlin
public object Dns_v1beta2Functions {
/**
* Fetches the representation of an existing Change.
* @param argument null
* @return null
*/
public suspend fun getChange(argument: GetChangePlainArgs): GetChangeResult =
getChangeResultToKotlin(getChangePlain(argument.toJava()).await())
/**
* @see [getChange].
* @param changeId
* @param clientOperationId
* @param managedZone
* @param project
* @return null
*/
public suspend fun getChange(
changeId: String,
clientOperationId: String? = null,
managedZone: String,
project: String? = null,
): GetChangeResult {
val argument = GetChangePlainArgs(
changeId = changeId,
clientOperationId = clientOperationId,
managedZone = managedZone,
project = project,
)
return getChangeResultToKotlin(getChangePlain(argument.toJava()).await())
}
/**
* @see [getChange].
* @param argument Builder for [com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetChangePlainArgs].
* @return null
*/
public suspend fun getChange(argument: suspend GetChangePlainArgsBuilder.() -> Unit): GetChangeResult {
val builder = GetChangePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getChangeResultToKotlin(getChangePlain(builtArgument.toJava()).await())
}
/**
* Fetches the representation of an existing ManagedZone.
* @param argument null
* @return null
*/
public suspend fun getManagedZone(argument: GetManagedZonePlainArgs): GetManagedZoneResult =
getManagedZoneResultToKotlin(getManagedZonePlain(argument.toJava()).await())
/**
* @see [getManagedZone].
* @param clientOperationId
* @param managedZone
* @param project
* @return null
*/
public suspend fun getManagedZone(
clientOperationId: String? = null,
managedZone: String,
project: String? = null,
): GetManagedZoneResult {
val argument = GetManagedZonePlainArgs(
clientOperationId = clientOperationId,
managedZone = managedZone,
project = project,
)
return getManagedZoneResultToKotlin(getManagedZonePlain(argument.toJava()).await())
}
/**
* @see [getManagedZone].
* @param argument Builder for [com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetManagedZonePlainArgs].
* @return null
*/
public suspend fun getManagedZone(argument: suspend GetManagedZonePlainArgsBuilder.() -> Unit): GetManagedZoneResult {
val builder = GetManagedZonePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getManagedZoneResultToKotlin(getManagedZonePlain(builtArgument.toJava()).await())
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
* @param argument null
* @return null
*/
public suspend fun getManagedZoneIamPolicy(argument: GetManagedZoneIamPolicyPlainArgs): GetManagedZoneIamPolicyResult =
getManagedZoneIamPolicyResultToKotlin(getManagedZoneIamPolicyPlain(argument.toJava()).await())
/**
* @see [getManagedZoneIamPolicy].
* @param managedZone
* @param project
* @return null
*/
public suspend fun getManagedZoneIamPolicy(managedZone: String, project: String? = null): GetManagedZoneIamPolicyResult {
val argument = GetManagedZoneIamPolicyPlainArgs(
managedZone = managedZone,
project = project,
)
return getManagedZoneIamPolicyResultToKotlin(getManagedZoneIamPolicyPlain(argument.toJava()).await())
}
/**
* @see [getManagedZoneIamPolicy].
* @param argument Builder for [com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetManagedZoneIamPolicyPlainArgs].
* @return null
*/
public suspend fun getManagedZoneIamPolicy(argument: suspend GetManagedZoneIamPolicyPlainArgsBuilder.() -> Unit): GetManagedZoneIamPolicyResult {
val builder = GetManagedZoneIamPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getManagedZoneIamPolicyResultToKotlin(getManagedZoneIamPolicyPlain(builtArgument.toJava()).await())
}
/**
* Fetches the representation of an existing Policy.
* @param argument null
* @return null
*/
public suspend fun getPolicy(argument: GetPolicyPlainArgs): GetPolicyResult =
getPolicyResultToKotlin(getPolicyPlain(argument.toJava()).await())
/**
* @see [getPolicy].
* @param clientOperationId
* @param policy
* @param project
* @return null
*/
public suspend fun getPolicy(
clientOperationId: String? = null,
policy: String,
project: String? = null,
): GetPolicyResult {
val argument = GetPolicyPlainArgs(
clientOperationId = clientOperationId,
policy = policy,
project = project,
)
return getPolicyResultToKotlin(getPolicyPlain(argument.toJava()).await())
}
/**
* @see [getPolicy].
* @param argument Builder for [com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetPolicyPlainArgs].
* @return null
*/
public suspend fun getPolicy(argument: suspend GetPolicyPlainArgsBuilder.() -> Unit): GetPolicyResult {
val builder = GetPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getPolicyResultToKotlin(getPolicyPlain(builtArgument.toJava()).await())
}
/**
* Fetches the representation of an existing ResourceRecordSet.
* @param argument null
* @return null
*/
public suspend fun getResourceRecordSet(argument: GetResourceRecordSetPlainArgs): GetResourceRecordSetResult =
getResourceRecordSetResultToKotlin(getResourceRecordSetPlain(argument.toJava()).await())
/**
* @see [getResourceRecordSet].
* @param clientOperationId
* @param managedZone
* @param name
* @param project
* @param type
* @return null
*/
public suspend fun getResourceRecordSet(
clientOperationId: String? = null,
managedZone: String,
name: String,
project: String? = null,
type: String,
): GetResourceRecordSetResult {
val argument = GetResourceRecordSetPlainArgs(
clientOperationId = clientOperationId,
managedZone = managedZone,
name = name,
project = project,
type = type,
)
return getResourceRecordSetResultToKotlin(getResourceRecordSetPlain(argument.toJava()).await())
}
/**
* @see [getResourceRecordSet].
* @param argument Builder for [com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetResourceRecordSetPlainArgs].
* @return null
*/
public suspend fun getResourceRecordSet(argument: suspend GetResourceRecordSetPlainArgsBuilder.() -> Unit): GetResourceRecordSetResult {
val builder = GetResourceRecordSetPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getResourceRecordSetResultToKotlin(getResourceRecordSetPlain(builtArgument.toJava()).await())
}
/**
* Fetches the representation of an existing Response Policy.
* @param argument null
* @return null
*/
public suspend fun getResponsePolicy(argument: GetResponsePolicyPlainArgs): GetResponsePolicyResult =
getResponsePolicyResultToKotlin(getResponsePolicyPlain(argument.toJava()).await())
/**
* @see [getResponsePolicy].
* @param clientOperationId
* @param project
* @param responsePolicy
* @return null
*/
public suspend fun getResponsePolicy(
clientOperationId: String? = null,
project: String? = null,
responsePolicy: String,
): GetResponsePolicyResult {
val argument = GetResponsePolicyPlainArgs(
clientOperationId = clientOperationId,
project = project,
responsePolicy = responsePolicy,
)
return getResponsePolicyResultToKotlin(getResponsePolicyPlain(argument.toJava()).await())
}
/**
* @see [getResponsePolicy].
* @param argument Builder for [com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetResponsePolicyPlainArgs].
* @return null
*/
public suspend fun getResponsePolicy(argument: suspend GetResponsePolicyPlainArgsBuilder.() -> Unit): GetResponsePolicyResult {
val builder = GetResponsePolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getResponsePolicyResultToKotlin(getResponsePolicyPlain(builtArgument.toJava()).await())
}
/**
* Fetches the representation of an existing Response Policy Rule.
* @param argument null
* @return null
*/
public suspend fun getResponsePolicyRule(argument: GetResponsePolicyRulePlainArgs): GetResponsePolicyRuleResult =
getResponsePolicyRuleResultToKotlin(getResponsePolicyRulePlain(argument.toJava()).await())
/**
* @see [getResponsePolicyRule].
* @param clientOperationId
* @param project
* @param responsePolicy
* @param responsePolicyRule
* @return null
*/
public suspend fun getResponsePolicyRule(
clientOperationId: String? = null,
project: String? = null,
responsePolicy: String,
responsePolicyRule: String,
): GetResponsePolicyRuleResult {
val argument = GetResponsePolicyRulePlainArgs(
clientOperationId = clientOperationId,
project = project,
responsePolicy = responsePolicy,
responsePolicyRule = responsePolicyRule,
)
return getResponsePolicyRuleResultToKotlin(getResponsePolicyRulePlain(argument.toJava()).await())
}
/**
* @see [getResponsePolicyRule].
* @param argument Builder for [com.pulumi.googlenative.dns.v1beta2.kotlin.inputs.GetResponsePolicyRulePlainArgs].
* @return null
*/
public suspend fun getResponsePolicyRule(argument: suspend GetResponsePolicyRulePlainArgsBuilder.() -> Unit): GetResponsePolicyRuleResult {
val builder = GetResponsePolicyRulePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getResponsePolicyRuleResultToKotlin(getResponsePolicyRulePlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy