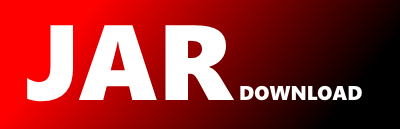
com.pulumi.googlenative.eventarc.v1.kotlin.Eventarc_v1Functions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.eventarc.v1.kotlin
import com.pulumi.googlenative.eventarc.v1.Eventarc_v1Functions.getChannelConnectionIamPolicyPlain
import com.pulumi.googlenative.eventarc.v1.Eventarc_v1Functions.getChannelConnectionPlain
import com.pulumi.googlenative.eventarc.v1.Eventarc_v1Functions.getChannelIamPolicyPlain
import com.pulumi.googlenative.eventarc.v1.Eventarc_v1Functions.getChannelPlain
import com.pulumi.googlenative.eventarc.v1.Eventarc_v1Functions.getTriggerIamPolicyPlain
import com.pulumi.googlenative.eventarc.v1.Eventarc_v1Functions.getTriggerPlain
import com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetChannelConnectionIamPolicyPlainArgs
import com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetChannelConnectionIamPolicyPlainArgsBuilder
import com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetChannelConnectionPlainArgs
import com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetChannelConnectionPlainArgsBuilder
import com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetChannelIamPolicyPlainArgs
import com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetChannelIamPolicyPlainArgsBuilder
import com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetChannelPlainArgs
import com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetChannelPlainArgsBuilder
import com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetTriggerIamPolicyPlainArgs
import com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetTriggerIamPolicyPlainArgsBuilder
import com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetTriggerPlainArgs
import com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetTriggerPlainArgsBuilder
import com.pulumi.googlenative.eventarc.v1.kotlin.outputs.GetChannelConnectionIamPolicyResult
import com.pulumi.googlenative.eventarc.v1.kotlin.outputs.GetChannelConnectionResult
import com.pulumi.googlenative.eventarc.v1.kotlin.outputs.GetChannelIamPolicyResult
import com.pulumi.googlenative.eventarc.v1.kotlin.outputs.GetChannelResult
import com.pulumi.googlenative.eventarc.v1.kotlin.outputs.GetTriggerIamPolicyResult
import com.pulumi.googlenative.eventarc.v1.kotlin.outputs.GetTriggerResult
import kotlinx.coroutines.future.await
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.googlenative.eventarc.v1.kotlin.outputs.GetChannelConnectionIamPolicyResult.Companion.toKotlin as getChannelConnectionIamPolicyResultToKotlin
import com.pulumi.googlenative.eventarc.v1.kotlin.outputs.GetChannelConnectionResult.Companion.toKotlin as getChannelConnectionResultToKotlin
import com.pulumi.googlenative.eventarc.v1.kotlin.outputs.GetChannelIamPolicyResult.Companion.toKotlin as getChannelIamPolicyResultToKotlin
import com.pulumi.googlenative.eventarc.v1.kotlin.outputs.GetChannelResult.Companion.toKotlin as getChannelResultToKotlin
import com.pulumi.googlenative.eventarc.v1.kotlin.outputs.GetTriggerIamPolicyResult.Companion.toKotlin as getTriggerIamPolicyResultToKotlin
import com.pulumi.googlenative.eventarc.v1.kotlin.outputs.GetTriggerResult.Companion.toKotlin as getTriggerResultToKotlin
public object Eventarc_v1Functions {
/**
* Get a single Channel.
* @param argument null
* @return null
*/
public suspend fun getChannel(argument: GetChannelPlainArgs): GetChannelResult =
getChannelResultToKotlin(getChannelPlain(argument.toJava()).await())
/**
* @see [getChannel].
* @param channelId
* @param location
* @param project
* @return null
*/
public suspend fun getChannel(
channelId: String,
location: String,
project: String? = null,
): GetChannelResult {
val argument = GetChannelPlainArgs(
channelId = channelId,
location = location,
project = project,
)
return getChannelResultToKotlin(getChannelPlain(argument.toJava()).await())
}
/**
* @see [getChannel].
* @param argument Builder for [com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetChannelPlainArgs].
* @return null
*/
public suspend fun getChannel(argument: suspend GetChannelPlainArgsBuilder.() -> Unit): GetChannelResult {
val builder = GetChannelPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getChannelResultToKotlin(getChannelPlain(builtArgument.toJava()).await())
}
/**
* Get a single ChannelConnection.
* @param argument null
* @return null
*/
public suspend fun getChannelConnection(argument: GetChannelConnectionPlainArgs): GetChannelConnectionResult =
getChannelConnectionResultToKotlin(getChannelConnectionPlain(argument.toJava()).await())
/**
* @see [getChannelConnection].
* @param channelConnectionId
* @param location
* @param project
* @return null
*/
public suspend fun getChannelConnection(
channelConnectionId: String,
location: String,
project: String? = null,
): GetChannelConnectionResult {
val argument = GetChannelConnectionPlainArgs(
channelConnectionId = channelConnectionId,
location = location,
project = project,
)
return getChannelConnectionResultToKotlin(getChannelConnectionPlain(argument.toJava()).await())
}
/**
* @see [getChannelConnection].
* @param argument Builder for [com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetChannelConnectionPlainArgs].
* @return null
*/
public suspend fun getChannelConnection(argument: suspend GetChannelConnectionPlainArgsBuilder.() -> Unit): GetChannelConnectionResult {
val builder = GetChannelConnectionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getChannelConnectionResultToKotlin(getChannelConnectionPlain(builtArgument.toJava()).await())
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
* @param argument null
* @return null
*/
public suspend fun getChannelConnectionIamPolicy(argument: GetChannelConnectionIamPolicyPlainArgs): GetChannelConnectionIamPolicyResult =
getChannelConnectionIamPolicyResultToKotlin(getChannelConnectionIamPolicyPlain(argument.toJava()).await())
/**
* @see [getChannelConnectionIamPolicy].
* @param channelConnectionId
* @param location
* @param optionsRequestedPolicyVersion
* @param project
* @return null
*/
public suspend fun getChannelConnectionIamPolicy(
channelConnectionId: String,
location: String,
optionsRequestedPolicyVersion: Int? = null,
project: String? = null,
): GetChannelConnectionIamPolicyResult {
val argument = GetChannelConnectionIamPolicyPlainArgs(
channelConnectionId = channelConnectionId,
location = location,
optionsRequestedPolicyVersion = optionsRequestedPolicyVersion,
project = project,
)
return getChannelConnectionIamPolicyResultToKotlin(getChannelConnectionIamPolicyPlain(argument.toJava()).await())
}
/**
* @see [getChannelConnectionIamPolicy].
* @param argument Builder for [com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetChannelConnectionIamPolicyPlainArgs].
* @return null
*/
public suspend fun getChannelConnectionIamPolicy(argument: suspend GetChannelConnectionIamPolicyPlainArgsBuilder.() -> Unit): GetChannelConnectionIamPolicyResult {
val builder = GetChannelConnectionIamPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getChannelConnectionIamPolicyResultToKotlin(getChannelConnectionIamPolicyPlain(builtArgument.toJava()).await())
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
* @param argument null
* @return null
*/
public suspend fun getChannelIamPolicy(argument: GetChannelIamPolicyPlainArgs): GetChannelIamPolicyResult =
getChannelIamPolicyResultToKotlin(getChannelIamPolicyPlain(argument.toJava()).await())
/**
* @see [getChannelIamPolicy].
* @param channelId
* @param location
* @param optionsRequestedPolicyVersion
* @param project
* @return null
*/
public suspend fun getChannelIamPolicy(
channelId: String,
location: String,
optionsRequestedPolicyVersion: Int? = null,
project: String? = null,
): GetChannelIamPolicyResult {
val argument = GetChannelIamPolicyPlainArgs(
channelId = channelId,
location = location,
optionsRequestedPolicyVersion = optionsRequestedPolicyVersion,
project = project,
)
return getChannelIamPolicyResultToKotlin(getChannelIamPolicyPlain(argument.toJava()).await())
}
/**
* @see [getChannelIamPolicy].
* @param argument Builder for [com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetChannelIamPolicyPlainArgs].
* @return null
*/
public suspend fun getChannelIamPolicy(argument: suspend GetChannelIamPolicyPlainArgsBuilder.() -> Unit): GetChannelIamPolicyResult {
val builder = GetChannelIamPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getChannelIamPolicyResultToKotlin(getChannelIamPolicyPlain(builtArgument.toJava()).await())
}
/**
* Get a single trigger.
* @param argument null
* @return null
*/
public suspend fun getTrigger(argument: GetTriggerPlainArgs): GetTriggerResult =
getTriggerResultToKotlin(getTriggerPlain(argument.toJava()).await())
/**
* @see [getTrigger].
* @param location
* @param project
* @param triggerId
* @return null
*/
public suspend fun getTrigger(
location: String,
project: String? = null,
triggerId: String,
): GetTriggerResult {
val argument = GetTriggerPlainArgs(
location = location,
project = project,
triggerId = triggerId,
)
return getTriggerResultToKotlin(getTriggerPlain(argument.toJava()).await())
}
/**
* @see [getTrigger].
* @param argument Builder for [com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetTriggerPlainArgs].
* @return null
*/
public suspend fun getTrigger(argument: suspend GetTriggerPlainArgsBuilder.() -> Unit): GetTriggerResult {
val builder = GetTriggerPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getTriggerResultToKotlin(getTriggerPlain(builtArgument.toJava()).await())
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
* @param argument null
* @return null
*/
public suspend fun getTriggerIamPolicy(argument: GetTriggerIamPolicyPlainArgs): GetTriggerIamPolicyResult =
getTriggerIamPolicyResultToKotlin(getTriggerIamPolicyPlain(argument.toJava()).await())
/**
* @see [getTriggerIamPolicy].
* @param location
* @param optionsRequestedPolicyVersion
* @param project
* @param triggerId
* @return null
*/
public suspend fun getTriggerIamPolicy(
location: String,
optionsRequestedPolicyVersion: Int? = null,
project: String? = null,
triggerId: String,
): GetTriggerIamPolicyResult {
val argument = GetTriggerIamPolicyPlainArgs(
location = location,
optionsRequestedPolicyVersion = optionsRequestedPolicyVersion,
project = project,
triggerId = triggerId,
)
return getTriggerIamPolicyResultToKotlin(getTriggerIamPolicyPlain(argument.toJava()).await())
}
/**
* @see [getTriggerIamPolicy].
* @param argument Builder for [com.pulumi.googlenative.eventarc.v1.kotlin.inputs.GetTriggerIamPolicyPlainArgs].
* @return null
*/
public suspend fun getTriggerIamPolicy(argument: suspend GetTriggerIamPolicyPlainArgsBuilder.() -> Unit): GetTriggerIamPolicyResult {
val builder = GetTriggerIamPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getTriggerIamPolicyResultToKotlin(getTriggerIamPolicyPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy