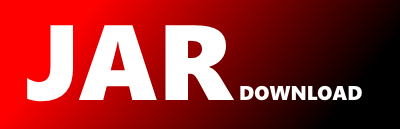
com.pulumi.googlenative.file.v1.kotlin.Instance.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.`file`.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.`file`.v1.kotlin.outputs.FileShareConfigResponse
import com.pulumi.googlenative.`file`.v1.kotlin.outputs.NetworkConfigResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.googlenative.`file`.v1.kotlin.outputs.FileShareConfigResponse.Companion.toKotlin as fileShareConfigResponseToKotlin
import com.pulumi.googlenative.`file`.v1.kotlin.outputs.NetworkConfigResponse.Companion.toKotlin as networkConfigResponseToKotlin
/**
* Builder for [Instance].
*/
@PulumiTagMarker
public class InstanceResourceBuilder internal constructor() {
public var name: String? = null
public var args: InstanceArgs = InstanceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend InstanceArgsBuilder.() -> Unit) {
val builder = InstanceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Instance {
val builtJavaResource = com.pulumi.googlenative.`file`.v1.Instance(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Instance(builtJavaResource)
}
}
/**
* Creates an instance. When creating from a backup, the capacity of the new instance needs to be equal to or larger than the capacity of the backup (and also equal to or larger than the minimum capacity of the tier).
* Auto-naming is currently not supported for this resource.
*/
public class Instance internal constructor(
override val javaResource: com.pulumi.googlenative.`file`.v1.Instance,
) : KotlinCustomResource(javaResource, InstanceMapper) {
/**
* The time when the instance was created.
*/
public val createTime: Output
get() = javaResource.createTime().applyValue({ args0 -> args0 })
/**
* The description of the instance (2048 characters or less).
*/
public val description: Output
get() = javaResource.description().applyValue({ args0 -> args0 })
/**
* Server-specified ETag for the instance resource to prevent simultaneous updates from overwriting each other.
*/
public val etag: Output
get() = javaResource.etag().applyValue({ args0 -> args0 })
/**
* File system shares on the instance. For this version, only a single file share is supported.
*/
public val fileShares: Output>
get() = javaResource.fileShares().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
fileShareConfigResponseToKotlin(args0)
})
})
})
/**
* Required. The name of the instance to create. The name must be unique for the specified project and location.
*/
public val instanceId: Output
get() = javaResource.instanceId().applyValue({ args0 -> args0 })
/**
* KMS key name used for data encryption.
*/
public val kmsKeyName: Output
get() = javaResource.kmsKeyName().applyValue({ args0 -> args0 })
/**
* Resource labels to represent user provided metadata.
*/
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy