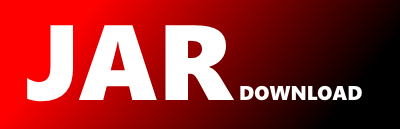
com.pulumi.googlenative.firebase.v1beta1.kotlin.WebAppArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.firebase.v1beta1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.firebase.v1beta1.WebAppArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Requests the creation of a new WebApp in the specified FirebaseProject. The result of this call is an `Operation` which can be used to track the provisioning process. The `Operation` is automatically deleted after completion, so there is no need to call `DeleteOperation`.
* Note - this resource's API doesn't support deletion. When deleted, the resource will persist
* on Google Cloud even though it will be deleted from Pulumi state.
* @property apiKeyId The globally unique, Google-assigned identifier (UID) for the Firebase API key associated with the `WebApp`. Be aware that this value is the UID of the API key, _not_ the [`keyString`](https://cloud.google.com/api-keys/docs/reference/rest/v2/projects.locations.keys#Key.FIELDS.key_string) of the API key. The `keyString` is the value that can be found in the App's [configuration artifact](../../rest/v1beta1/projects.webApps/getConfig). If `api_key_id` is not set in requests to [`webApps.Create`](../../rest/v1beta1/projects.webApps/create), then Firebase automatically associates an `api_key_id` with the `WebApp`. This auto-associated key may be an existing valid key or, if no valid key exists, a new one will be provisioned. In patch requests, `api_key_id` cannot be set to an empty value, and the new UID must have no restrictions or only have restrictions that are valid for the associated `WebApp`. We recommend using the [Google Cloud Console](https://console.cloud.google.com/apis/credentials) to manage API keys.
* @property appUrls The URLs where the `WebApp` is hosted.
* @property displayName The user-assigned display name for the `WebApp`.
* @property etag This checksum is computed by the server based on the value of other fields, and it may be sent with update requests to ensure the client has an up-to-date value before proceeding. Learn more about `etag` in Google's [AIP-154 standard](https://google.aip.dev/154#declarative-friendly-resources). This etag is strongly validated.
* @property name The resource name of the WebApp, in the format: projects/PROJECT_IDENTIFIER /webApps/APP_ID * PROJECT_IDENTIFIER: the parent Project's [`ProjectNumber`](../projects#FirebaseProject.FIELDS.project_number) ***(recommended)*** or its [`ProjectId`](../projects#FirebaseProject.FIELDS.project_id). Learn more about using project identifiers in Google's [AIP 2510 standard](https://google.aip.dev/cloud/2510). Note that the value for PROJECT_IDENTIFIER in any response body will be the `ProjectId`. * APP_ID: the globally unique, Firebase-assigned identifier for the App (see [`appId`](../projects.webApps#WebApp.FIELDS.app_id)).
* @property project
*/
public data class WebAppArgs(
public val apiKeyId: Output? = null,
public val appUrls: Output>? = null,
public val displayName: Output? = null,
public val etag: Output? = null,
public val name: Output? = null,
public val project: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.firebase.v1beta1.WebAppArgs =
com.pulumi.googlenative.firebase.v1beta1.WebAppArgs.builder()
.apiKeyId(apiKeyId?.applyValue({ args0 -> args0 }))
.appUrls(appUrls?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.etag(etag?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [WebAppArgs].
*/
@PulumiTagMarker
public class WebAppArgsBuilder internal constructor() {
private var apiKeyId: Output? = null
private var appUrls: Output>? = null
private var displayName: Output? = null
private var etag: Output? = null
private var name: Output? = null
private var project: Output? = null
/**
* @param value The globally unique, Google-assigned identifier (UID) for the Firebase API key associated with the `WebApp`. Be aware that this value is the UID of the API key, _not_ the [`keyString`](https://cloud.google.com/api-keys/docs/reference/rest/v2/projects.locations.keys#Key.FIELDS.key_string) of the API key. The `keyString` is the value that can be found in the App's [configuration artifact](../../rest/v1beta1/projects.webApps/getConfig). If `api_key_id` is not set in requests to [`webApps.Create`](../../rest/v1beta1/projects.webApps/create), then Firebase automatically associates an `api_key_id` with the `WebApp`. This auto-associated key may be an existing valid key or, if no valid key exists, a new one will be provisioned. In patch requests, `api_key_id` cannot be set to an empty value, and the new UID must have no restrictions or only have restrictions that are valid for the associated `WebApp`. We recommend using the [Google Cloud Console](https://console.cloud.google.com/apis/credentials) to manage API keys.
*/
@JvmName("cokcfpdtgwxycaot")
public suspend fun apiKeyId(`value`: Output) {
this.apiKeyId = value
}
/**
* @param value The URLs where the `WebApp` is hosted.
*/
@JvmName("utgkikqnduhpjlqj")
public suspend fun appUrls(`value`: Output>) {
this.appUrls = value
}
@JvmName("akgagkjokxatbnqk")
public suspend fun appUrls(vararg values: Output) {
this.appUrls = Output.all(values.asList())
}
/**
* @param values The URLs where the `WebApp` is hosted.
*/
@JvmName("aptqmsinwlcomykx")
public suspend fun appUrls(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy