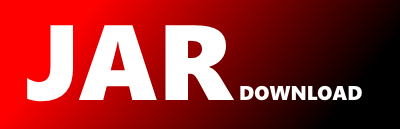
com.pulumi.googlenative.firestore.v1.kotlin.BackupScheduleArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.firestore.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.firestore.v1.BackupScheduleArgs.builder
import com.pulumi.googlenative.firestore.v1.kotlin.inputs.GoogleFirestoreAdminV1DailyRecurrenceArgs
import com.pulumi.googlenative.firestore.v1.kotlin.inputs.GoogleFirestoreAdminV1DailyRecurrenceArgsBuilder
import com.pulumi.googlenative.firestore.v1.kotlin.inputs.GoogleFirestoreAdminV1WeeklyRecurrenceArgs
import com.pulumi.googlenative.firestore.v1.kotlin.inputs.GoogleFirestoreAdminV1WeeklyRecurrenceArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Creates a backup schedule on a database. At most two backup schedules can be configured on a database, one daily backup schedule with retention up to 7 days and one weekly backup schedule with retention up to 14 weeks.
* Auto-naming is currently not supported for this resource.
* @property dailyRecurrence For a schedule that runs daily at a specified time.
* @property databaseId
* @property project
* @property retention At what relative time in the future, compared to the creation time of the backup should the backup be deleted, i.e. keep backups for 7 days.
* @property weeklyRecurrence For a schedule that runs weekly on a specific day and time.
*/
public data class BackupScheduleArgs(
public val dailyRecurrence: Output? = null,
public val databaseId: Output? = null,
public val project: Output? = null,
public val retention: Output? = null,
public val weeklyRecurrence: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.firestore.v1.BackupScheduleArgs =
com.pulumi.googlenative.firestore.v1.BackupScheduleArgs.builder()
.dailyRecurrence(dailyRecurrence?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.databaseId(databaseId?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.retention(retention?.applyValue({ args0 -> args0 }))
.weeklyRecurrence(
weeklyRecurrence?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [BackupScheduleArgs].
*/
@PulumiTagMarker
public class BackupScheduleArgsBuilder internal constructor() {
private var dailyRecurrence: Output? = null
private var databaseId: Output? = null
private var project: Output? = null
private var retention: Output? = null
private var weeklyRecurrence: Output? = null
/**
* @param value For a schedule that runs daily at a specified time.
*/
@JvmName("xbhybkaorqwuohng")
public suspend fun dailyRecurrence(`value`: Output) {
this.dailyRecurrence = value
}
/**
* @param value
*/
@JvmName("cvfffsrowxwgpxeb")
public suspend fun databaseId(`value`: Output) {
this.databaseId = value
}
/**
* @param value
*/
@JvmName("ovtngpfifimecrgi")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value At what relative time in the future, compared to the creation time of the backup should the backup be deleted, i.e. keep backups for 7 days.
*/
@JvmName("fiptaffhromafvke")
public suspend fun retention(`value`: Output) {
this.retention = value
}
/**
* @param value For a schedule that runs weekly on a specific day and time.
*/
@JvmName("xkwnuiuiblmcllki")
public suspend fun weeklyRecurrence(`value`: Output) {
this.weeklyRecurrence = value
}
/**
* @param value For a schedule that runs daily at a specified time.
*/
@JvmName("wqmrcscihujaanmb")
public suspend fun dailyRecurrence(`value`: GoogleFirestoreAdminV1DailyRecurrenceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dailyRecurrence = mapped
}
/**
* @param argument For a schedule that runs daily at a specified time.
*/
@JvmName("nxsjoljxvtgtbdpj")
public suspend fun dailyRecurrence(argument: suspend GoogleFirestoreAdminV1DailyRecurrenceArgsBuilder.() -> Unit) {
val toBeMapped = GoogleFirestoreAdminV1DailyRecurrenceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.dailyRecurrence = mapped
}
/**
* @param value
*/
@JvmName("ynwxlcotawraslyr")
public suspend fun databaseId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.databaseId = mapped
}
/**
* @param value
*/
@JvmName("aagrnelscjuqopim")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value At what relative time in the future, compared to the creation time of the backup should the backup be deleted, i.e. keep backups for 7 days.
*/
@JvmName("ctklfqijpquupdwc")
public suspend fun retention(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retention = mapped
}
/**
* @param value For a schedule that runs weekly on a specific day and time.
*/
@JvmName("fbrxdselhbmktkpu")
public suspend fun weeklyRecurrence(`value`: GoogleFirestoreAdminV1WeeklyRecurrenceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.weeklyRecurrence = mapped
}
/**
* @param argument For a schedule that runs weekly on a specific day and time.
*/
@JvmName("hqvqmptnqrlfhivd")
public suspend fun weeklyRecurrence(argument: suspend GoogleFirestoreAdminV1WeeklyRecurrenceArgsBuilder.() -> Unit) {
val toBeMapped = GoogleFirestoreAdminV1WeeklyRecurrenceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.weeklyRecurrence = mapped
}
internal fun build(): BackupScheduleArgs = BackupScheduleArgs(
dailyRecurrence = dailyRecurrence,
databaseId = databaseId,
project = project,
retention = retention,
weeklyRecurrence = weeklyRecurrence,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy