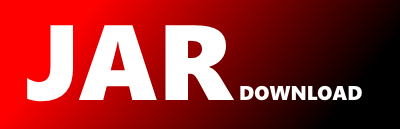
com.pulumi.googlenative.gkebackup.v1.kotlin.RestorePlanArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.gkebackup.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.gkebackup.v1.RestorePlanArgs.builder
import com.pulumi.googlenative.gkebackup.v1.kotlin.inputs.RestoreConfigArgs
import com.pulumi.googlenative.gkebackup.v1.kotlin.inputs.RestoreConfigArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Creates a new RestorePlan in a given location.
* Auto-naming is currently not supported for this resource.
* @property backupPlan Immutable. A reference to the BackupPlan from which Backups may be used as the source for Restores created via this RestorePlan. Format: `projects/*/locations/*/backupPlans/*`.
* @property cluster Immutable. The target cluster into which Restores created via this RestorePlan will restore data. NOTE: the cluster's region must be the same as the RestorePlan. Valid formats: - `projects/*/locations/*/clusters/*` - `projects/*/zones/*/clusters/*`
* @property description User specified descriptive string for this RestorePlan.
* @property labels A set of custom labels supplied by user.
* @property location
* @property project
* @property restoreConfig Configuration of Restores created via this RestorePlan.
* @property restorePlanId Required. The client-provided short name for the RestorePlan resource. This name must: - be between 1 and 63 characters long (inclusive) - consist of only lower-case ASCII letters, numbers, and dashes - start with a lower-case letter - end with a lower-case letter or number - be unique within the set of RestorePlans in this location
* */*/*/*/*/*/*/*/*/
*/
public data class RestorePlanArgs(
public val backupPlan: Output? = null,
public val cluster: Output? = null,
public val description: Output? = null,
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy