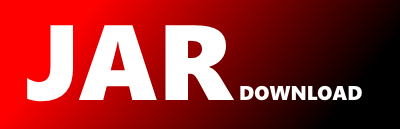
com.pulumi.googlenative.gkeonprem.v1.kotlin.VmwareNodePool.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.gkeonprem.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.ResourceStatusResponse
import com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.VmwareNodeConfigResponse
import com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.VmwareNodePoolAutoscalingConfigResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.ResourceStatusResponse.Companion.toKotlin as resourceStatusResponseToKotlin
import com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.VmwareNodeConfigResponse.Companion.toKotlin as vmwareNodeConfigResponseToKotlin
import com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.VmwareNodePoolAutoscalingConfigResponse.Companion.toKotlin as vmwareNodePoolAutoscalingConfigResponseToKotlin
/**
* Builder for [VmwareNodePool].
*/
@PulumiTagMarker
public class VmwareNodePoolResourceBuilder internal constructor() {
public var name: String? = null
public var args: VmwareNodePoolArgs = VmwareNodePoolArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend VmwareNodePoolArgsBuilder.() -> Unit) {
val builder = VmwareNodePoolArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): VmwareNodePool {
val builtJavaResource =
com.pulumi.googlenative.gkeonprem.v1.VmwareNodePool(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return VmwareNodePool(builtJavaResource)
}
}
/**
* Creates a new VMware node pool in a given project, location and VMWare cluster.
*/
public class VmwareNodePool internal constructor(
override val javaResource: com.pulumi.googlenative.gkeonprem.v1.VmwareNodePool,
) : KotlinCustomResource(javaResource, VmwareNodePoolMapper) {
/**
* Annotations on the node pool. This field has the same restrictions as Kubernetes annotations. The total size of all keys and values combined is limited to 256k. Key can have 2 segments: prefix (optional) and name (required), separated by a slash (/). Prefix must be a DNS subdomain. Name must be 63 characters or less, begin and end with alphanumerics, with dashes (-), underscores (_), dots (.), and alphanumerics between.
*/
public val annotations: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy