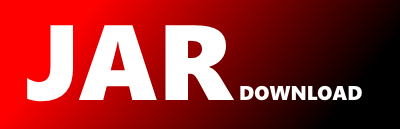
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.GetBareMetalAdminClusterResult.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
/**
*
* @property annotations Annotations on the bare metal admin cluster. This field has the same restrictions as Kubernetes annotations. The total size of all keys and values combined is limited to 256k. Key can have 2 segments: prefix (optional) and name (required), separated by a slash (/). Prefix must be a DNS subdomain. Name must be 63 characters or less, begin and end with alphanumerics, with dashes (-), underscores (_), dots (.), and alphanumerics between.
* @property bareMetalVersion The Anthos clusters on bare metal version for the bare metal admin cluster.
* @property clusterOperations Cluster operations configuration.
* @property controlPlane Control plane configuration.
* @property createTime The time at which this bare metal admin cluster was created.
* @property deleteTime The time at which this bare metal admin cluster was deleted. If the resource is not deleted, this must be empty
* @property description A human readable description of this bare metal admin cluster.
* @property endpoint The IP address name of bare metal admin cluster's API server.
* @property etag This checksum is computed by the server based on the value of other fields, and may be sent on update and delete requests to ensure the client has an up-to-date value before proceeding. Allows clients to perform consistent read-modify-writes through optimistic concurrency control.
* @property fleet Fleet configuration for the cluster.
* @property loadBalancer Load balancer configuration.
* @property localName The object name of the bare metal cluster custom resource. This field is used to support conflicting names when enrolling existing clusters to the API. When used as a part of cluster enrollment, this field will differ from the ID in the resource name. For new clusters, this field will match the user provided cluster name and be visible in the last component of the resource name. It is not modifiable. All users should use this name to access their cluster using gkectl or kubectl and should expect to see the local name when viewing admin cluster controller logs.
* @property maintenanceConfig Maintenance configuration.
* @property maintenanceStatus MaintenanceStatus representing state of maintenance.
* @property name Immutable. The bare metal admin cluster resource name.
* @property networkConfig Network configuration.
* @property nodeAccessConfig Node access related configurations.
* @property nodeConfig Workload node configuration.
* @property osEnvironmentConfig OS environment related configurations.
* @property proxy Proxy configuration.
* @property reconciling If set, there are currently changes in flight to the bare metal Admin Cluster.
* @property securityConfig Security related configuration.
* @property state The current state of the bare metal admin cluster.
* @property status ResourceStatus representing detailed cluster status.
* @property storage Storage configuration.
* @property uid The unique identifier of the bare metal admin cluster.
* @property updateTime The time at which this bare metal admin cluster was last updated.
* @property validationCheck ValidationCheck representing the result of the preflight check.
*/
public data class GetBareMetalAdminClusterResult(
public val annotations: Map,
public val bareMetalVersion: String,
public val clusterOperations: BareMetalAdminClusterOperationsConfigResponse,
public val controlPlane: BareMetalAdminControlPlaneConfigResponse,
public val createTime: String,
public val deleteTime: String,
public val description: String,
public val endpoint: String,
public val etag: String,
public val fleet: FleetResponse,
public val loadBalancer: BareMetalAdminLoadBalancerConfigResponse,
public val localName: String,
public val maintenanceConfig: BareMetalAdminMaintenanceConfigResponse,
public val maintenanceStatus: BareMetalAdminMaintenanceStatusResponse,
public val name: String,
public val networkConfig: BareMetalAdminNetworkConfigResponse,
public val nodeAccessConfig: BareMetalAdminNodeAccessConfigResponse,
public val nodeConfig: BareMetalAdminWorkloadNodeConfigResponse,
public val osEnvironmentConfig: BareMetalAdminOsEnvironmentConfigResponse,
public val proxy: BareMetalAdminProxyConfigResponse,
public val reconciling: Boolean,
public val securityConfig: BareMetalAdminSecurityConfigResponse,
public val state: String,
public val status: ResourceStatusResponse,
public val storage: BareMetalAdminStorageConfigResponse,
public val uid: String,
public val updateTime: String,
public val validationCheck: ValidationCheckResponse,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.googlenative.gkeonprem.v1.outputs.GetBareMetalAdminClusterResult): GetBareMetalAdminClusterResult = GetBareMetalAdminClusterResult(
annotations = javaType.annotations().map({ args0 -> args0.key.to(args0.value) }).toMap(),
bareMetalVersion = javaType.bareMetalVersion(),
clusterOperations = javaType.clusterOperations().let({ args0 ->
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.BareMetalAdminClusterOperationsConfigResponse.Companion.toKotlin(args0)
}),
controlPlane = javaType.controlPlane().let({ args0 ->
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.BareMetalAdminControlPlaneConfigResponse.Companion.toKotlin(args0)
}),
createTime = javaType.createTime(),
deleteTime = javaType.deleteTime(),
description = javaType.description(),
endpoint = javaType.endpoint(),
etag = javaType.etag(),
fleet = javaType.fleet().let({ args0 ->
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.FleetResponse.Companion.toKotlin(args0)
}),
loadBalancer = javaType.loadBalancer().let({ args0 ->
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.BareMetalAdminLoadBalancerConfigResponse.Companion.toKotlin(args0)
}),
localName = javaType.localName(),
maintenanceConfig = javaType.maintenanceConfig().let({ args0 ->
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.BareMetalAdminMaintenanceConfigResponse.Companion.toKotlin(args0)
}),
maintenanceStatus = javaType.maintenanceStatus().let({ args0 ->
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.BareMetalAdminMaintenanceStatusResponse.Companion.toKotlin(args0)
}),
name = javaType.name(),
networkConfig = javaType.networkConfig().let({ args0 ->
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.BareMetalAdminNetworkConfigResponse.Companion.toKotlin(args0)
}),
nodeAccessConfig = javaType.nodeAccessConfig().let({ args0 ->
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.BareMetalAdminNodeAccessConfigResponse.Companion.toKotlin(args0)
}),
nodeConfig = javaType.nodeConfig().let({ args0 ->
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.BareMetalAdminWorkloadNodeConfigResponse.Companion.toKotlin(args0)
}),
osEnvironmentConfig = javaType.osEnvironmentConfig().let({ args0 ->
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.BareMetalAdminOsEnvironmentConfigResponse.Companion.toKotlin(args0)
}),
proxy = javaType.proxy().let({ args0 ->
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.BareMetalAdminProxyConfigResponse.Companion.toKotlin(args0)
}),
reconciling = javaType.reconciling(),
securityConfig = javaType.securityConfig().let({ args0 ->
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.BareMetalAdminSecurityConfigResponse.Companion.toKotlin(args0)
}),
state = javaType.state(),
status = javaType.status().let({ args0 ->
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.ResourceStatusResponse.Companion.toKotlin(args0)
}),
storage = javaType.storage().let({ args0 ->
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.BareMetalAdminStorageConfigResponse.Companion.toKotlin(args0)
}),
uid = javaType.uid(),
updateTime = javaType.updateTime(),
validationCheck = javaType.validationCheck().let({ args0 ->
com.pulumi.googlenative.gkeonprem.v1.kotlin.outputs.ValidationCheckResponse.Companion.toKotlin(args0)
}),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy