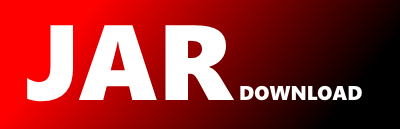
com.pulumi.googlenative.healthcare.v1.kotlin.inputs.GoogleCloudHealthcareV1ConsentPolicyArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.healthcare.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.healthcare.v1.inputs.GoogleCloudHealthcareV1ConsentPolicyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Represents a user's consent in terms of the resources that can be accessed and under what conditions.
* @property authorizationRule The request conditions to meet to grant access. In addition to any supported comparison operators, authorization rules may have `IN` operator as well as at most 10 logical operators that are limited to `AND` (`&&`), `OR` (`||`).
* @property resourceAttributes The resources that this policy applies to. A resource is a match if it matches all the attributes listed here. If empty, this policy applies to all User data mappings for the given user.
*/
public data class GoogleCloudHealthcareV1ConsentPolicyArgs(
public val authorizationRule: Output,
public val resourceAttributes: Output>? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.healthcare.v1.inputs.GoogleCloudHealthcareV1ConsentPolicyArgs =
com.pulumi.googlenative.healthcare.v1.inputs.GoogleCloudHealthcareV1ConsentPolicyArgs.builder()
.authorizationRule(authorizationRule.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.resourceAttributes(
resourceAttributes?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
).build()
}
/**
* Builder for [GoogleCloudHealthcareV1ConsentPolicyArgs].
*/
@PulumiTagMarker
public class GoogleCloudHealthcareV1ConsentPolicyArgsBuilder internal constructor() {
private var authorizationRule: Output? = null
private var resourceAttributes: Output>? = null
/**
* @param value The request conditions to meet to grant access. In addition to any supported comparison operators, authorization rules may have `IN` operator as well as at most 10 logical operators that are limited to `AND` (`&&`), `OR` (`||`).
*/
@JvmName("hwmwxuevsykcganu")
public suspend fun authorizationRule(`value`: Output) {
this.authorizationRule = value
}
/**
* @param value The resources that this policy applies to. A resource is a match if it matches all the attributes listed here. If empty, this policy applies to all User data mappings for the given user.
*/
@JvmName("yfhrklayhthskphp")
public suspend fun resourceAttributes(`value`: Output>) {
this.resourceAttributes = value
}
@JvmName("psfdqnfswxuwhqqn")
public suspend fun resourceAttributes(vararg values: Output) {
this.resourceAttributes = Output.all(values.asList())
}
/**
* @param values The resources that this policy applies to. A resource is a match if it matches all the attributes listed here. If empty, this policy applies to all User data mappings for the given user.
*/
@JvmName("adjlosjtegtajffw")
public suspend fun resourceAttributes(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy