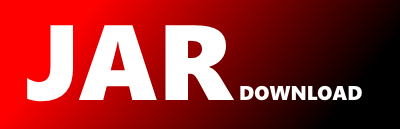
com.pulumi.googlenative.healthcare.v1beta1.kotlin.AttributeDefinition.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.healthcare.v1beta1.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [AttributeDefinition].
*/
@PulumiTagMarker
public class AttributeDefinitionResourceBuilder internal constructor() {
public var name: String? = null
public var args: AttributeDefinitionArgs = AttributeDefinitionArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AttributeDefinitionArgsBuilder.() -> Unit) {
val builder = AttributeDefinitionArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): AttributeDefinition {
val builtJavaResource =
com.pulumi.googlenative.healthcare.v1beta1.AttributeDefinition(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return AttributeDefinition(builtJavaResource)
}
}
/**
* Creates a new Attribute definition in the parent consent store.
*/
public class AttributeDefinition internal constructor(
override val javaResource: com.pulumi.googlenative.healthcare.v1beta1.AttributeDefinition,
) : KotlinCustomResource(javaResource, AttributeDefinitionMapper) {
/**
* Possible values for the attribute. The number of allowed values must not exceed 500. An empty list is invalid. The list can only be expanded after creation.
*/
public val allowedValues: Output>
get() = javaResource.allowedValues().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* Required. The ID of the Attribute definition to create. The string must match the following regex: `_a-zA-Z{0,255}` and must not be a reserved keyword within the Common Expression Language as listed on https://github.com/google/cel-spec/blob/master/doc/langdef.md.
*/
public val attributeDefinitionId: Output
get() = javaResource.attributeDefinitionId().applyValue({ args0 -> args0 })
/**
* The category of the attribute. The value of this field cannot be changed after creation.
*/
public val category: Output
get() = javaResource.category().applyValue({ args0 -> args0 })
/**
* Optional. Default values of the attribute in Consents. If no default values are specified, it defaults to an empty value.
*/
public val consentDefaultValues: Output>
get() = javaResource.consentDefaultValues().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
public val consentStoreId: Output
get() = javaResource.consentStoreId().applyValue({ args0 -> args0 })
/**
* Optional. Default value of the attribute in User data mappings. If no default value is specified, it defaults to an empty value. This field is only applicable to attributes of the category `RESOURCE`.
*/
public val dataMappingDefaultValue: Output
get() = javaResource.dataMappingDefaultValue().applyValue({ args0 -> args0 })
public val datasetId: Output
get() = javaResource.datasetId().applyValue({ args0 -> args0 })
/**
* Optional. A description of the attribute.
*/
public val description: Output
get() = javaResource.description().applyValue({ args0 -> args0 })
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* Resource name of the Attribute definition, of the form `projects/{project_id}/locations/{location_id}/datasets/{dataset_id}/consentStores/{consent_store_id}/attributeDefinitions/{attribute_definition_id}`. Cannot be changed after creation.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
}
public object AttributeDefinitionMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.googlenative.healthcare.v1beta1.AttributeDefinition::class == javaResource::class
override fun map(javaResource: Resource): AttributeDefinition = AttributeDefinition(
javaResource
as com.pulumi.googlenative.healthcare.v1beta1.AttributeDefinition,
)
}
/**
* @see [AttributeDefinition].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [AttributeDefinition].
*/
public suspend fun attributeDefinition(
name: String,
block: suspend AttributeDefinitionResourceBuilder.() -> Unit,
): AttributeDefinition {
val builder = AttributeDefinitionResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [AttributeDefinition].
* @param name The _unique_ name of the resulting resource.
*/
public fun attributeDefinition(name: String): AttributeDefinition {
val builder = AttributeDefinitionResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy