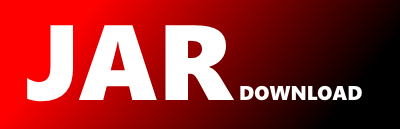
com.pulumi.googlenative.healthcare.v1beta1.kotlin.inputs.ValidationConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.healthcare.v1beta1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.healthcare.v1beta1.inputs.ValidationConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Contains the configuration for FHIR profiles and validation.
* @property disableFhirpathValidation Whether to disable FHIRPath validation for incoming resources. Set this to true to disable checking incoming resources for conformance against FHIRPath requirement defined in the FHIR specification. This property only affects resource types that do not have profiles configured for them, any rules in enabled implementation guides will still be enforced.
* @property disableProfileValidation Whether to disable profile validation for this FHIR store. Set this to true to disable checking incoming resources for conformance against StructureDefinitions in this FHIR store.
* @property disableReferenceTypeValidation Whether to disable reference type validation for incoming resources. Set this to true to disable checking incoming resources for conformance against reference type requirement defined in the FHIR specification. This property only affects resource types that do not have profiles configured for them, any rules in enabled implementation guides will still be enforced.
* @property disableRequiredFieldValidation Whether to disable required fields validation for incoming resources. Set this to true to disable checking incoming resources for conformance against required fields requirement defined in the FHIR specification. This property only affects resource types that do not have profiles configured for them, any rules in enabled implementation guides will still be enforced.
* @property enabledImplementationGuides A list of ImplementationGuide URLs in this FHIR store that are used to configure the profiles to use for validation. For example, to use the US Core profiles for validation, set `enabled_implementation_guides` to `["http://hl7.org/fhir/us/core/ImplementationGuide/ig"]`. If `enabled_implementation_guides` is empty or omitted, then incoming resources are only required to conform to the base FHIR profiles. Otherwise, a resource must conform to at least one profile listed in the `global` property of one of the enabled ImplementationGuides. The Cloud Healthcare API does not currently enforce all of the rules in a StructureDefinition. The following rules are supported: - min/max - minValue/maxValue - maxLength - type - fixed[x] - pattern[x] on simple types - slicing, when using "value" as the discriminator type When a URL cannot be resolved (for example, in a type assertion), the server does not return an error.
*/
public data class ValidationConfigArgs(
public val disableFhirpathValidation: Output? = null,
public val disableProfileValidation: Output? = null,
public val disableReferenceTypeValidation: Output? = null,
public val disableRequiredFieldValidation: Output? = null,
public val enabledImplementationGuides: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.healthcare.v1beta1.inputs.ValidationConfigArgs =
com.pulumi.googlenative.healthcare.v1beta1.inputs.ValidationConfigArgs.builder()
.disableFhirpathValidation(disableFhirpathValidation?.applyValue({ args0 -> args0 }))
.disableProfileValidation(disableProfileValidation?.applyValue({ args0 -> args0 }))
.disableReferenceTypeValidation(disableReferenceTypeValidation?.applyValue({ args0 -> args0 }))
.disableRequiredFieldValidation(disableRequiredFieldValidation?.applyValue({ args0 -> args0 }))
.enabledImplementationGuides(
enabledImplementationGuides?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
).build()
}
/**
* Builder for [ValidationConfigArgs].
*/
@PulumiTagMarker
public class ValidationConfigArgsBuilder internal constructor() {
private var disableFhirpathValidation: Output? = null
private var disableProfileValidation: Output? = null
private var disableReferenceTypeValidation: Output? = null
private var disableRequiredFieldValidation: Output? = null
private var enabledImplementationGuides: Output>? = null
/**
* @param value Whether to disable FHIRPath validation for incoming resources. Set this to true to disable checking incoming resources for conformance against FHIRPath requirement defined in the FHIR specification. This property only affects resource types that do not have profiles configured for them, any rules in enabled implementation guides will still be enforced.
*/
@JvmName("qevhnurqeckvppby")
public suspend fun disableFhirpathValidation(`value`: Output) {
this.disableFhirpathValidation = value
}
/**
* @param value Whether to disable profile validation for this FHIR store. Set this to true to disable checking incoming resources for conformance against StructureDefinitions in this FHIR store.
*/
@JvmName("jsisjgayuakslpth")
public suspend fun disableProfileValidation(`value`: Output) {
this.disableProfileValidation = value
}
/**
* @param value Whether to disable reference type validation for incoming resources. Set this to true to disable checking incoming resources for conformance against reference type requirement defined in the FHIR specification. This property only affects resource types that do not have profiles configured for them, any rules in enabled implementation guides will still be enforced.
*/
@JvmName("itpgwgvwcjqgcyxd")
public suspend fun disableReferenceTypeValidation(`value`: Output) {
this.disableReferenceTypeValidation = value
}
/**
* @param value Whether to disable required fields validation for incoming resources. Set this to true to disable checking incoming resources for conformance against required fields requirement defined in the FHIR specification. This property only affects resource types that do not have profiles configured for them, any rules in enabled implementation guides will still be enforced.
*/
@JvmName("ljdvjqlkmsjjptil")
public suspend fun disableRequiredFieldValidation(`value`: Output) {
this.disableRequiredFieldValidation = value
}
/**
* @param value A list of ImplementationGuide URLs in this FHIR store that are used to configure the profiles to use for validation. For example, to use the US Core profiles for validation, set `enabled_implementation_guides` to `["http://hl7.org/fhir/us/core/ImplementationGuide/ig"]`. If `enabled_implementation_guides` is empty or omitted, then incoming resources are only required to conform to the base FHIR profiles. Otherwise, a resource must conform to at least one profile listed in the `global` property of one of the enabled ImplementationGuides. The Cloud Healthcare API does not currently enforce all of the rules in a StructureDefinition. The following rules are supported: - min/max - minValue/maxValue - maxLength - type - fixed[x] - pattern[x] on simple types - slicing, when using "value" as the discriminator type When a URL cannot be resolved (for example, in a type assertion), the server does not return an error.
*/
@JvmName("ydugexvwxqplbeeh")
public suspend fun enabledImplementationGuides(`value`: Output>) {
this.enabledImplementationGuides = value
}
@JvmName("dskdtdwdkdgeicvi")
public suspend fun enabledImplementationGuides(vararg values: Output) {
this.enabledImplementationGuides = Output.all(values.asList())
}
/**
* @param values A list of ImplementationGuide URLs in this FHIR store that are used to configure the profiles to use for validation. For example, to use the US Core profiles for validation, set `enabled_implementation_guides` to `["http://hl7.org/fhir/us/core/ImplementationGuide/ig"]`. If `enabled_implementation_guides` is empty or omitted, then incoming resources are only required to conform to the base FHIR profiles. Otherwise, a resource must conform to at least one profile listed in the `global` property of one of the enabled ImplementationGuides. The Cloud Healthcare API does not currently enforce all of the rules in a StructureDefinition. The following rules are supported: - min/max - minValue/maxValue - maxLength - type - fixed[x] - pattern[x] on simple types - slicing, when using "value" as the discriminator type When a URL cannot be resolved (for example, in a type assertion), the server does not return an error.
*/
@JvmName("vhfbfhmmxegyllgj")
public suspend fun enabledImplementationGuides(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy