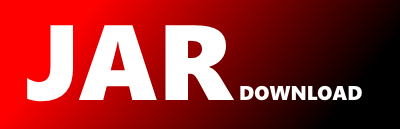
com.pulumi.googlenative.jobs.v4.kotlin.inputs.CompensationEntryArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.jobs.v4.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.jobs.v4.inputs.CompensationEntryArgs.builder
import com.pulumi.googlenative.jobs.v4.kotlin.enums.CompensationEntryType
import com.pulumi.googlenative.jobs.v4.kotlin.enums.CompensationEntryUnit
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* A compensation entry that represents one component of compensation, such as base pay, bonus, or other compensation type. Annualization: One compensation entry can be annualized if - it contains valid amount or range. - and its expected_units_per_year is set or can be derived. Its annualized range is determined as (amount or range) times expected_units_per_year.
* @property amount Compensation amount.
* @property description Compensation description. For example, could indicate equity terms or provide additional context to an estimated bonus.
* @property expectedUnitsPerYear Expected number of units paid each year. If not specified, when Job.employment_types is FULLTIME, a default value is inferred based on unit. Default values: - HOURLY: 2080 - DAILY: 260 - WEEKLY: 52 - MONTHLY: 12 - ANNUAL: 1
* @property range Compensation range.
* @property type Compensation type. Default is CompensationType.COMPENSATION_TYPE_UNSPECIFIED.
* @property unit Frequency of the specified amount. Default is CompensationUnit.COMPENSATION_UNIT_UNSPECIFIED.
*/
public data class CompensationEntryArgs(
public val amount: Output? = null,
public val description: Output? = null,
public val expectedUnitsPerYear: Output? = null,
public val range: Output? = null,
public val type: Output? = null,
public val unit: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.jobs.v4.inputs.CompensationEntryArgs =
com.pulumi.googlenative.jobs.v4.inputs.CompensationEntryArgs.builder()
.amount(amount?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.description(description?.applyValue({ args0 -> args0 }))
.expectedUnitsPerYear(expectedUnitsPerYear?.applyValue({ args0 -> args0 }))
.range(range?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.type(type?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.unit(unit?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [CompensationEntryArgs].
*/
@PulumiTagMarker
public class CompensationEntryArgsBuilder internal constructor() {
private var amount: Output? = null
private var description: Output? = null
private var expectedUnitsPerYear: Output? = null
private var range: Output? = null
private var type: Output? = null
private var unit: Output? = null
/**
* @param value Compensation amount.
*/
@JvmName("auqeejfxxpxjopjp")
public suspend fun amount(`value`: Output) {
this.amount = value
}
/**
* @param value Compensation description. For example, could indicate equity terms or provide additional context to an estimated bonus.
*/
@JvmName("glfifsxyfsgtemal")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Expected number of units paid each year. If not specified, when Job.employment_types is FULLTIME, a default value is inferred based on unit. Default values: - HOURLY: 2080 - DAILY: 260 - WEEKLY: 52 - MONTHLY: 12 - ANNUAL: 1
*/
@JvmName("hwwqpqwetqelocsn")
public suspend fun expectedUnitsPerYear(`value`: Output) {
this.expectedUnitsPerYear = value
}
/**
* @param value Compensation range.
*/
@JvmName("cstubfafrtbffqvs")
public suspend fun range(`value`: Output) {
this.range = value
}
/**
* @param value Compensation type. Default is CompensationType.COMPENSATION_TYPE_UNSPECIFIED.
*/
@JvmName("uqgchfyqjosojebl")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value Frequency of the specified amount. Default is CompensationUnit.COMPENSATION_UNIT_UNSPECIFIED.
*/
@JvmName("yvvgnwubmlgeixmx")
public suspend fun unit(`value`: Output) {
this.unit = value
}
/**
* @param value Compensation amount.
*/
@JvmName("ypvwoftjpkgsvloj")
public suspend fun amount(`value`: MoneyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.amount = mapped
}
/**
* @param argument Compensation amount.
*/
@JvmName("olymwvjrnkbiioih")
public suspend fun amount(argument: suspend MoneyArgsBuilder.() -> Unit) {
val toBeMapped = MoneyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.amount = mapped
}
/**
* @param value Compensation description. For example, could indicate equity terms or provide additional context to an estimated bonus.
*/
@JvmName("lqyhkjmaognyrnmf")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value Expected number of units paid each year. If not specified, when Job.employment_types is FULLTIME, a default value is inferred based on unit. Default values: - HOURLY: 2080 - DAILY: 260 - WEEKLY: 52 - MONTHLY: 12 - ANNUAL: 1
*/
@JvmName("dskajryojljavhro")
public suspend fun expectedUnitsPerYear(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.expectedUnitsPerYear = mapped
}
/**
* @param value Compensation range.
*/
@JvmName("anqnxxgrbdyberux")
public suspend fun range(`value`: CompensationRangeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.range = mapped
}
/**
* @param argument Compensation range.
*/
@JvmName("dcmarsrnvxujhuxi")
public suspend fun range(argument: suspend CompensationRangeArgsBuilder.() -> Unit) {
val toBeMapped = CompensationRangeArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.range = mapped
}
/**
* @param value Compensation type. Default is CompensationType.COMPENSATION_TYPE_UNSPECIFIED.
*/
@JvmName("cengnsxqfqwywiko")
public suspend fun type(`value`: CompensationEntryType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.type = mapped
}
/**
* @param value Frequency of the specified amount. Default is CompensationUnit.COMPENSATION_UNIT_UNSPECIFIED.
*/
@JvmName("kgbemiwffviuyygu")
public suspend fun unit(`value`: CompensationEntryUnit?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.unit = mapped
}
internal fun build(): CompensationEntryArgs = CompensationEntryArgs(
amount = amount,
description = description,
expectedUnitsPerYear = expectedUnitsPerYear,
range = range,
type = type,
unit = unit,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy