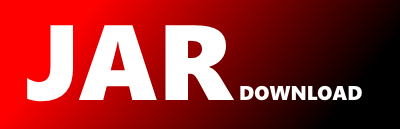
com.pulumi.googlenative.memcache.v1.kotlin.Instance.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.memcache.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.memcache.v1.kotlin.outputs.GoogleCloudMemcacheV1MaintenancePolicyResponse
import com.pulumi.googlenative.memcache.v1.kotlin.outputs.InstanceMessageResponse
import com.pulumi.googlenative.memcache.v1.kotlin.outputs.MaintenanceScheduleResponse
import com.pulumi.googlenative.memcache.v1.kotlin.outputs.MemcacheParametersResponse
import com.pulumi.googlenative.memcache.v1.kotlin.outputs.NodeConfigResponse
import com.pulumi.googlenative.memcache.v1.kotlin.outputs.NodeResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.googlenative.memcache.v1.kotlin.outputs.GoogleCloudMemcacheV1MaintenancePolicyResponse.Companion.toKotlin as googleCloudMemcacheV1MaintenancePolicyResponseToKotlin
import com.pulumi.googlenative.memcache.v1.kotlin.outputs.InstanceMessageResponse.Companion.toKotlin as instanceMessageResponseToKotlin
import com.pulumi.googlenative.memcache.v1.kotlin.outputs.MaintenanceScheduleResponse.Companion.toKotlin as maintenanceScheduleResponseToKotlin
import com.pulumi.googlenative.memcache.v1.kotlin.outputs.MemcacheParametersResponse.Companion.toKotlin as memcacheParametersResponseToKotlin
import com.pulumi.googlenative.memcache.v1.kotlin.outputs.NodeConfigResponse.Companion.toKotlin as nodeConfigResponseToKotlin
import com.pulumi.googlenative.memcache.v1.kotlin.outputs.NodeResponse.Companion.toKotlin as nodeResponseToKotlin
/**
* Builder for [Instance].
*/
@PulumiTagMarker
public class InstanceResourceBuilder internal constructor() {
public var name: String? = null
public var args: InstanceArgs = InstanceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend InstanceArgsBuilder.() -> Unit) {
val builder = InstanceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Instance {
val builtJavaResource = com.pulumi.googlenative.memcache.v1.Instance(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Instance(builtJavaResource)
}
}
/**
* Creates a new Instance in a given location.
*/
public class Instance internal constructor(
override val javaResource: com.pulumi.googlenative.memcache.v1.Instance,
) : KotlinCustomResource(javaResource, InstanceMapper) {
/**
* The full name of the Google Compute Engine [network](/compute/docs/networks-and-firewalls#networks) to which the instance is connected. If left unspecified, the `default` network will be used.
*/
public val authorizedNetwork: Output
get() = javaResource.authorizedNetwork().applyValue({ args0 -> args0 })
/**
* The time the instance was created.
*/
public val createTime: Output
get() = javaResource.createTime().applyValue({ args0 -> args0 })
/**
* Endpoint for the Discovery API.
*/
public val discoveryEndpoint: Output
get() = javaResource.discoveryEndpoint().applyValue({ args0 -> args0 })
/**
* User provided name for the instance, which is only used for display purposes. Cannot be more than 80 characters.
*/
public val displayName: Output
get() = javaResource.displayName().applyValue({ args0 -> args0 })
/**
* Required. The logical name of the Memcached instance in the user project with the following restrictions: * Must contain only lowercase letters, numbers, and hyphens. * Must start with a letter. * Must be between 1-40 characters. * Must end with a number or a letter. * Must be unique within the user project / location. If any of the above are not met, the API raises an invalid argument error.
*/
public val instanceId: Output
get() = javaResource.instanceId().applyValue({ args0 -> args0 })
/**
* List of messages that describe the current state of the Memcached instance.
*/
public val instanceMessages: Output>
get() = javaResource.instanceMessages().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> instanceMessageResponseToKotlin(args0) })
})
})
/**
* Resource labels to represent user-provided metadata. Refer to cloud documentation on labels for more details. https://cloud.google.com/compute/docs/labeling-resources
*/
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy