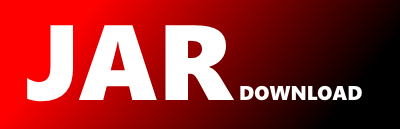
com.pulumi.googlenative.metastore.v1alpha.kotlin.Federation.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.metastore.v1alpha.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
/**
* Builder for [Federation].
*/
@PulumiTagMarker
public class FederationResourceBuilder internal constructor() {
public var name: String? = null
public var args: FederationArgs = FederationArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend FederationArgsBuilder.() -> Unit) {
val builder = FederationArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Federation {
val builtJavaResource =
com.pulumi.googlenative.metastore.v1alpha.Federation(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Federation(builtJavaResource)
}
}
/**
* Creates a metastore federation in a project and location.
*/
public class Federation internal constructor(
override val javaResource: com.pulumi.googlenative.metastore.v1alpha.Federation,
) : KotlinCustomResource(javaResource, FederationMapper) {
/**
* A map from BackendMetastore rank to BackendMetastores from which the federation service serves metadata at query time. The map key represents the order in which BackendMetastores should be evaluated to resolve database names at query time and should be greater than or equal to zero. A BackendMetastore with a lower number will be evaluated before a BackendMetastore with a higher number.
*/
public val backendMetastores: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy