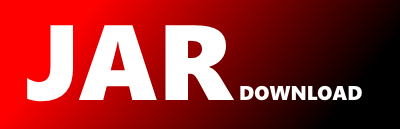
com.pulumi.googlenative.metastore.v1beta.kotlin.BackupArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.metastore.v1beta.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.metastore.v1beta.BackupArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Creates a new backup in a given project and location.
* @property backupId Required. The ID of the backup, which is used as the final component of the backup's name.This value must be between 1 and 64 characters long, begin with a letter, end with a letter or number, and consist of alpha-numeric ASCII characters or hyphens.
* @property description The description of the backup.
* @property location
* @property name Immutable. The relative resource name of the backup, in the following form:projects/{project_number}/locations/{location_id}/services/{service_id}/backups/{backup_id}
* @property project
* @property requestId Optional. A request ID. Specify a unique request ID to allow the server to ignore the request if it has completed. The server will ignore subsequent requests that provide a duplicate request ID for at least 60 minutes after the first request.For example, if an initial request times out, followed by another request with the same request ID, the server ignores the second request to prevent the creation of duplicate commitments.The request ID must be a valid UUID (https://en.wikipedia.org/wiki/Universally_unique_identifier#Format) A zero UUID (00000000-0000-0000-0000-000000000000) is not supported.
* @property serviceId
*/
public data class BackupArgs(
public val backupId: Output? = null,
public val description: Output? = null,
public val location: Output? = null,
public val name: Output? = null,
public val project: Output? = null,
public val requestId: Output? = null,
public val serviceId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.metastore.v1beta.BackupArgs =
com.pulumi.googlenative.metastore.v1beta.BackupArgs.builder()
.backupId(backupId?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.requestId(requestId?.applyValue({ args0 -> args0 }))
.serviceId(serviceId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BackupArgs].
*/
@PulumiTagMarker
public class BackupArgsBuilder internal constructor() {
private var backupId: Output? = null
private var description: Output? = null
private var location: Output? = null
private var name: Output? = null
private var project: Output? = null
private var requestId: Output? = null
private var serviceId: Output? = null
/**
* @param value Required. The ID of the backup, which is used as the final component of the backup's name.This value must be between 1 and 64 characters long, begin with a letter, end with a letter or number, and consist of alpha-numeric ASCII characters or hyphens.
*/
@JvmName("rjsiyljnngrcigit")
public suspend fun backupId(`value`: Output) {
this.backupId = value
}
/**
* @param value The description of the backup.
*/
@JvmName("floeiiokshcxxhld")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value
*/
@JvmName("mcqvauojasaxykqr")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value Immutable. The relative resource name of the backup, in the following form:projects/{project_number}/locations/{location_id}/services/{service_id}/backups/{backup_id}
*/
@JvmName("smheceijmstvtyom")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value
*/
@JvmName("smplygwxwpafbmxf")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value Optional. A request ID. Specify a unique request ID to allow the server to ignore the request if it has completed. The server will ignore subsequent requests that provide a duplicate request ID for at least 60 minutes after the first request.For example, if an initial request times out, followed by another request with the same request ID, the server ignores the second request to prevent the creation of duplicate commitments.The request ID must be a valid UUID (https://en.wikipedia.org/wiki/Universally_unique_identifier#Format) A zero UUID (00000000-0000-0000-0000-000000000000) is not supported.
*/
@JvmName("efbcdqtnautksbdt")
public suspend fun requestId(`value`: Output) {
this.requestId = value
}
/**
* @param value
*/
@JvmName("agpvrgdiodowfcfq")
public suspend fun serviceId(`value`: Output) {
this.serviceId = value
}
/**
* @param value Required. The ID of the backup, which is used as the final component of the backup's name.This value must be between 1 and 64 characters long, begin with a letter, end with a letter or number, and consist of alpha-numeric ASCII characters or hyphens.
*/
@JvmName("eypqssvuaylgmrok")
public suspend fun backupId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backupId = mapped
}
/**
* @param value The description of the backup.
*/
@JvmName("tqnomwrjksabkgsg")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value
*/
@JvmName("msckeufmndurbkhp")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value Immutable. The relative resource name of the backup, in the following form:projects/{project_number}/locations/{location_id}/services/{service_id}/backups/{backup_id}
*/
@JvmName("jtdyimhpnbvbsiwd")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value
*/
@JvmName("pdwypjqulebveesc")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value Optional. A request ID. Specify a unique request ID to allow the server to ignore the request if it has completed. The server will ignore subsequent requests that provide a duplicate request ID for at least 60 minutes after the first request.For example, if an initial request times out, followed by another request with the same request ID, the server ignores the second request to prevent the creation of duplicate commitments.The request ID must be a valid UUID (https://en.wikipedia.org/wiki/Universally_unique_identifier#Format) A zero UUID (00000000-0000-0000-0000-000000000000) is not supported.
*/
@JvmName("ponoylxgukkgoqnv")
public suspend fun requestId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requestId = mapped
}
/**
* @param value
*/
@JvmName("aoralrcesjldpewt")
public suspend fun serviceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceId = mapped
}
internal fun build(): BackupArgs = BackupArgs(
backupId = backupId,
description = description,
location = location,
name = name,
project = project,
requestId = requestId,
serviceId = serviceId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy