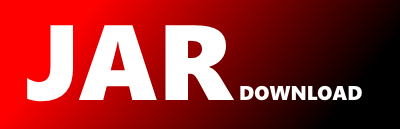
com.pulumi.googlenative.ml.v1.kotlin.Ml_v1Functions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.ml.v1.kotlin
import com.pulumi.googlenative.ml.v1.Ml_v1Functions.getJobIamPolicyPlain
import com.pulumi.googlenative.ml.v1.Ml_v1Functions.getJobPlain
import com.pulumi.googlenative.ml.v1.Ml_v1Functions.getModelIamPolicyPlain
import com.pulumi.googlenative.ml.v1.Ml_v1Functions.getModelPlain
import com.pulumi.googlenative.ml.v1.Ml_v1Functions.getStudyPlain
import com.pulumi.googlenative.ml.v1.Ml_v1Functions.getTrialPlain
import com.pulumi.googlenative.ml.v1.Ml_v1Functions.getVersionPlain
import com.pulumi.googlenative.ml.v1.kotlin.inputs.GetJobIamPolicyPlainArgs
import com.pulumi.googlenative.ml.v1.kotlin.inputs.GetJobIamPolicyPlainArgsBuilder
import com.pulumi.googlenative.ml.v1.kotlin.inputs.GetJobPlainArgs
import com.pulumi.googlenative.ml.v1.kotlin.inputs.GetJobPlainArgsBuilder
import com.pulumi.googlenative.ml.v1.kotlin.inputs.GetModelIamPolicyPlainArgs
import com.pulumi.googlenative.ml.v1.kotlin.inputs.GetModelIamPolicyPlainArgsBuilder
import com.pulumi.googlenative.ml.v1.kotlin.inputs.GetModelPlainArgs
import com.pulumi.googlenative.ml.v1.kotlin.inputs.GetModelPlainArgsBuilder
import com.pulumi.googlenative.ml.v1.kotlin.inputs.GetStudyPlainArgs
import com.pulumi.googlenative.ml.v1.kotlin.inputs.GetStudyPlainArgsBuilder
import com.pulumi.googlenative.ml.v1.kotlin.inputs.GetTrialPlainArgs
import com.pulumi.googlenative.ml.v1.kotlin.inputs.GetTrialPlainArgsBuilder
import com.pulumi.googlenative.ml.v1.kotlin.inputs.GetVersionPlainArgs
import com.pulumi.googlenative.ml.v1.kotlin.inputs.GetVersionPlainArgsBuilder
import com.pulumi.googlenative.ml.v1.kotlin.outputs.GetJobIamPolicyResult
import com.pulumi.googlenative.ml.v1.kotlin.outputs.GetJobResult
import com.pulumi.googlenative.ml.v1.kotlin.outputs.GetModelIamPolicyResult
import com.pulumi.googlenative.ml.v1.kotlin.outputs.GetModelResult
import com.pulumi.googlenative.ml.v1.kotlin.outputs.GetStudyResult
import com.pulumi.googlenative.ml.v1.kotlin.outputs.GetTrialResult
import com.pulumi.googlenative.ml.v1.kotlin.outputs.GetVersionResult
import kotlinx.coroutines.future.await
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.googlenative.ml.v1.kotlin.outputs.GetJobIamPolicyResult.Companion.toKotlin as getJobIamPolicyResultToKotlin
import com.pulumi.googlenative.ml.v1.kotlin.outputs.GetJobResult.Companion.toKotlin as getJobResultToKotlin
import com.pulumi.googlenative.ml.v1.kotlin.outputs.GetModelIamPolicyResult.Companion.toKotlin as getModelIamPolicyResultToKotlin
import com.pulumi.googlenative.ml.v1.kotlin.outputs.GetModelResult.Companion.toKotlin as getModelResultToKotlin
import com.pulumi.googlenative.ml.v1.kotlin.outputs.GetStudyResult.Companion.toKotlin as getStudyResultToKotlin
import com.pulumi.googlenative.ml.v1.kotlin.outputs.GetTrialResult.Companion.toKotlin as getTrialResultToKotlin
import com.pulumi.googlenative.ml.v1.kotlin.outputs.GetVersionResult.Companion.toKotlin as getVersionResultToKotlin
public object Ml_v1Functions {
/**
* Describes a job.
* @param argument null
* @return null
*/
public suspend fun getJob(argument: GetJobPlainArgs): GetJobResult =
getJobResultToKotlin(getJobPlain(argument.toJava()).await())
/**
* @see [getJob].
* @param jobId
* @param project
* @return null
*/
public suspend fun getJob(jobId: String, project: String? = null): GetJobResult {
val argument = GetJobPlainArgs(
jobId = jobId,
project = project,
)
return getJobResultToKotlin(getJobPlain(argument.toJava()).await())
}
/**
* @see [getJob].
* @param argument Builder for [com.pulumi.googlenative.ml.v1.kotlin.inputs.GetJobPlainArgs].
* @return null
*/
public suspend fun getJob(argument: suspend GetJobPlainArgsBuilder.() -> Unit): GetJobResult {
val builder = GetJobPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getJobResultToKotlin(getJobPlain(builtArgument.toJava()).await())
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
* @param argument null
* @return null
*/
public suspend fun getJobIamPolicy(argument: GetJobIamPolicyPlainArgs): GetJobIamPolicyResult =
getJobIamPolicyResultToKotlin(getJobIamPolicyPlain(argument.toJava()).await())
/**
* @see [getJobIamPolicy].
* @param jobId
* @param optionsRequestedPolicyVersion
* @param project
* @return null
*/
public suspend fun getJobIamPolicy(
jobId: String,
optionsRequestedPolicyVersion: Int? = null,
project: String? = null,
): GetJobIamPolicyResult {
val argument = GetJobIamPolicyPlainArgs(
jobId = jobId,
optionsRequestedPolicyVersion = optionsRequestedPolicyVersion,
project = project,
)
return getJobIamPolicyResultToKotlin(getJobIamPolicyPlain(argument.toJava()).await())
}
/**
* @see [getJobIamPolicy].
* @param argument Builder for [com.pulumi.googlenative.ml.v1.kotlin.inputs.GetJobIamPolicyPlainArgs].
* @return null
*/
public suspend fun getJobIamPolicy(argument: suspend GetJobIamPolicyPlainArgsBuilder.() -> Unit): GetJobIamPolicyResult {
val builder = GetJobIamPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getJobIamPolicyResultToKotlin(getJobIamPolicyPlain(builtArgument.toJava()).await())
}
/**
* Gets information about a model, including its name, the description (if set), and the default version (if at least one version of the model has been deployed).
* @param argument null
* @return null
*/
public suspend fun getModel(argument: GetModelPlainArgs): GetModelResult =
getModelResultToKotlin(getModelPlain(argument.toJava()).await())
/**
* @see [getModel].
* @param modelId
* @param project
* @return null
*/
public suspend fun getModel(modelId: String, project: String? = null): GetModelResult {
val argument = GetModelPlainArgs(
modelId = modelId,
project = project,
)
return getModelResultToKotlin(getModelPlain(argument.toJava()).await())
}
/**
* @see [getModel].
* @param argument Builder for [com.pulumi.googlenative.ml.v1.kotlin.inputs.GetModelPlainArgs].
* @return null
*/
public suspend fun getModel(argument: suspend GetModelPlainArgsBuilder.() -> Unit): GetModelResult {
val builder = GetModelPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getModelResultToKotlin(getModelPlain(builtArgument.toJava()).await())
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
* @param argument null
* @return null
*/
public suspend fun getModelIamPolicy(argument: GetModelIamPolicyPlainArgs): GetModelIamPolicyResult =
getModelIamPolicyResultToKotlin(getModelIamPolicyPlain(argument.toJava()).await())
/**
* @see [getModelIamPolicy].
* @param modelId
* @param optionsRequestedPolicyVersion
* @param project
* @return null
*/
public suspend fun getModelIamPolicy(
modelId: String,
optionsRequestedPolicyVersion: Int? = null,
project: String? = null,
): GetModelIamPolicyResult {
val argument = GetModelIamPolicyPlainArgs(
modelId = modelId,
optionsRequestedPolicyVersion = optionsRequestedPolicyVersion,
project = project,
)
return getModelIamPolicyResultToKotlin(getModelIamPolicyPlain(argument.toJava()).await())
}
/**
* @see [getModelIamPolicy].
* @param argument Builder for [com.pulumi.googlenative.ml.v1.kotlin.inputs.GetModelIamPolicyPlainArgs].
* @return null
*/
public suspend fun getModelIamPolicy(argument: suspend GetModelIamPolicyPlainArgsBuilder.() -> Unit): GetModelIamPolicyResult {
val builder = GetModelIamPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getModelIamPolicyResultToKotlin(getModelIamPolicyPlain(builtArgument.toJava()).await())
}
/**
* Gets a study.
* @param argument null
* @return null
*/
public suspend fun getStudy(argument: GetStudyPlainArgs): GetStudyResult =
getStudyResultToKotlin(getStudyPlain(argument.toJava()).await())
/**
* @see [getStudy].
* @param location
* @param project
* @param studyId
* @return null
*/
public suspend fun getStudy(
location: String,
project: String? = null,
studyId: String,
): GetStudyResult {
val argument = GetStudyPlainArgs(
location = location,
project = project,
studyId = studyId,
)
return getStudyResultToKotlin(getStudyPlain(argument.toJava()).await())
}
/**
* @see [getStudy].
* @param argument Builder for [com.pulumi.googlenative.ml.v1.kotlin.inputs.GetStudyPlainArgs].
* @return null
*/
public suspend fun getStudy(argument: suspend GetStudyPlainArgsBuilder.() -> Unit): GetStudyResult {
val builder = GetStudyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getStudyResultToKotlin(getStudyPlain(builtArgument.toJava()).await())
}
/**
* Gets a trial.
* @param argument null
* @return null
*/
public suspend fun getTrial(argument: GetTrialPlainArgs): GetTrialResult =
getTrialResultToKotlin(getTrialPlain(argument.toJava()).await())
/**
* @see [getTrial].
* @param location
* @param project
* @param studyId
* @param trialId
* @return null
*/
public suspend fun getTrial(
location: String,
project: String? = null,
studyId: String,
trialId: String,
): GetTrialResult {
val argument = GetTrialPlainArgs(
location = location,
project = project,
studyId = studyId,
trialId = trialId,
)
return getTrialResultToKotlin(getTrialPlain(argument.toJava()).await())
}
/**
* @see [getTrial].
* @param argument Builder for [com.pulumi.googlenative.ml.v1.kotlin.inputs.GetTrialPlainArgs].
* @return null
*/
public suspend fun getTrial(argument: suspend GetTrialPlainArgsBuilder.() -> Unit): GetTrialResult {
val builder = GetTrialPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getTrialResultToKotlin(getTrialPlain(builtArgument.toJava()).await())
}
/**
* Gets information about a model version. Models can have multiple versions. You can call projects.models.versions.list to get the same information that this method returns for all of the versions of a model.
* @param argument null
* @return null
*/
public suspend fun getVersion(argument: GetVersionPlainArgs): GetVersionResult =
getVersionResultToKotlin(getVersionPlain(argument.toJava()).await())
/**
* @see [getVersion].
* @param modelId
* @param project
* @param versionId
* @return null
*/
public suspend fun getVersion(
modelId: String,
project: String? = null,
versionId: String,
): GetVersionResult {
val argument = GetVersionPlainArgs(
modelId = modelId,
project = project,
versionId = versionId,
)
return getVersionResultToKotlin(getVersionPlain(argument.toJava()).await())
}
/**
* @see [getVersion].
* @param argument Builder for [com.pulumi.googlenative.ml.v1.kotlin.inputs.GetVersionPlainArgs].
* @return null
*/
public suspend fun getVersion(argument: suspend GetVersionPlainArgsBuilder.() -> Unit): GetVersionResult {
val builder = GetVersionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getVersionResultToKotlin(getVersionPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy