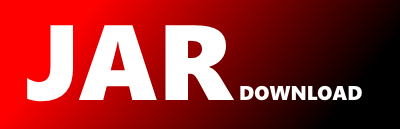
com.pulumi.googlenative.networksecurity.v1beta1.kotlin.TlsInspectionPolicyArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.networksecurity.v1beta1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.networksecurity.v1beta1.TlsInspectionPolicyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Creates a new TlsInspectionPolicy in a given project and location.
* @property caPool A CA pool resource used to issue interception certificates. The CA pool string has a relative resource path following the form "projects/{project}/locations/{location}/caPools/{ca_pool}".
* @property description Optional. Free-text description of the resource.
* @property location
* @property name Name of the resource. Name is of the form projects/{project}/locations/{location}/tlsInspectionPolicies/{tls_inspection_policy} tls_inspection_policy should match the pattern:(^[a-z]([a-z0-9-]{0,61}[a-z0-9])?$).
* @property project
* @property tlsInspectionPolicyId Required. Short name of the TlsInspectionPolicy resource to be created. This value should be 1-63 characters long, containing only letters, numbers, hyphens, and underscores, and should not start with a number. E.g. "tls_inspection_policy1".
*/
public data class TlsInspectionPolicyArgs(
public val caPool: Output? = null,
public val description: Output? = null,
public val location: Output? = null,
public val name: Output? = null,
public val project: Output? = null,
public val tlsInspectionPolicyId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.networksecurity.v1beta1.TlsInspectionPolicyArgs =
com.pulumi.googlenative.networksecurity.v1beta1.TlsInspectionPolicyArgs.builder()
.caPool(caPool?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.tlsInspectionPolicyId(tlsInspectionPolicyId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TlsInspectionPolicyArgs].
*/
@PulumiTagMarker
public class TlsInspectionPolicyArgsBuilder internal constructor() {
private var caPool: Output? = null
private var description: Output? = null
private var location: Output? = null
private var name: Output? = null
private var project: Output? = null
private var tlsInspectionPolicyId: Output? = null
/**
* @param value A CA pool resource used to issue interception certificates. The CA pool string has a relative resource path following the form "projects/{project}/locations/{location}/caPools/{ca_pool}".
*/
@JvmName("uwyhskuabfddprwq")
public suspend fun caPool(`value`: Output) {
this.caPool = value
}
/**
* @param value Optional. Free-text description of the resource.
*/
@JvmName("hpftophdsvvyunmx")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value
*/
@JvmName("wuqskmjlofxbqpct")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value Name of the resource. Name is of the form projects/{project}/locations/{location}/tlsInspectionPolicies/{tls_inspection_policy} tls_inspection_policy should match the pattern:(^[a-z]([a-z0-9-]{0,61}[a-z0-9])?$).
*/
@JvmName("ghgxvyuwwpofqebo")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value
*/
@JvmName("wiirjknatqvncufr")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value Required. Short name of the TlsInspectionPolicy resource to be created. This value should be 1-63 characters long, containing only letters, numbers, hyphens, and underscores, and should not start with a number. E.g. "tls_inspection_policy1".
*/
@JvmName("dwaqkpcwiticnico")
public suspend fun tlsInspectionPolicyId(`value`: Output) {
this.tlsInspectionPolicyId = value
}
/**
* @param value A CA pool resource used to issue interception certificates. The CA pool string has a relative resource path following the form "projects/{project}/locations/{location}/caPools/{ca_pool}".
*/
@JvmName("rodbfspklhjunujt")
public suspend fun caPool(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.caPool = mapped
}
/**
* @param value Optional. Free-text description of the resource.
*/
@JvmName("daftsxymeyykkbho")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value
*/
@JvmName("pvvpqthwlsgjirgm")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value Name of the resource. Name is of the form projects/{project}/locations/{location}/tlsInspectionPolicies/{tls_inspection_policy} tls_inspection_policy should match the pattern:(^[a-z]([a-z0-9-]{0,61}[a-z0-9])?$).
*/
@JvmName("rtxeyhkvhlrmuldt")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value
*/
@JvmName("erynqqssasvpwstn")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value Required. Short name of the TlsInspectionPolicy resource to be created. This value should be 1-63 characters long, containing only letters, numbers, hyphens, and underscores, and should not start with a number. E.g. "tls_inspection_policy1".
*/
@JvmName("tfvwyrddnpfkvkws")
public suspend fun tlsInspectionPolicyId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tlsInspectionPolicyId = mapped
}
internal fun build(): TlsInspectionPolicyArgs = TlsInspectionPolicyArgs(
caPool = caPool,
description = description,
location = location,
name = name,
project = project,
tlsInspectionPolicyId = tlsInspectionPolicyId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy