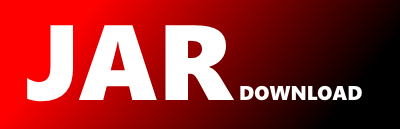
com.pulumi.googlenative.networkservices.v1beta1.kotlin.MeshArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.networkservices.v1beta1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.networkservices.v1beta1.MeshArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Creates a new Mesh in a given project and location.
* @property description Optional. A free-text description of the resource. Max length 1024 characters.
* @property interceptionPort Optional. If set to a valid TCP port (1-65535), instructs the SIDECAR proxy to listen on the specified port of localhost (127.0.0.1) address. The SIDECAR proxy will expect all traffic to be redirected to this port regardless of its actual ip:port destination. If unset, a port '15001' is used as the interception port. This will is applicable only for sidecar proxy deployments.
* @property labels Optional. Set of label tags associated with the Mesh resource.
* @property location
* @property meshId Required. Short name of the Mesh resource to be created.
* @property name Name of the Mesh resource. It matches pattern `projects/*/locations/global/meshes/`.
* @property project
* */
*/
public data class MeshArgs(
public val description: Output? = null,
public val interceptionPort: Output? = null,
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy