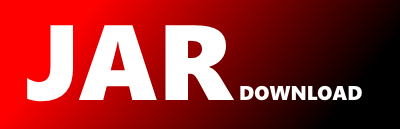
com.pulumi.googlenative.networkservices.v1beta1.kotlin.TcpRoute.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.networkservices.v1beta1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.networkservices.v1beta1.kotlin.outputs.TcpRouteRouteRuleResponse
import com.pulumi.googlenative.networkservices.v1beta1.kotlin.outputs.TcpRouteRouteRuleResponse.Companion.toKotlin
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
/**
* Builder for [TcpRoute].
*/
@PulumiTagMarker
public class TcpRouteResourceBuilder internal constructor() {
public var name: String? = null
public var args: TcpRouteArgs = TcpRouteArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend TcpRouteArgsBuilder.() -> Unit) {
val builder = TcpRouteArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): TcpRoute {
val builtJavaResource =
com.pulumi.googlenative.networkservices.v1beta1.TcpRoute(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return TcpRoute(builtJavaResource)
}
}
/**
* Creates a new TcpRoute in a given project and location.
*/
public class TcpRoute internal constructor(
override val javaResource: com.pulumi.googlenative.networkservices.v1beta1.TcpRoute,
) : KotlinCustomResource(javaResource, TcpRouteMapper) {
/**
* The timestamp when the resource was created.
*/
public val createTime: Output
get() = javaResource.createTime().applyValue({ args0 -> args0 })
/**
* Optional. A free-text description of the resource. Max length 1024 characters.
*/
public val description: Output
get() = javaResource.description().applyValue({ args0 -> args0 })
/**
* Optional. Gateways defines a list of gateways this TcpRoute is attached to, as one of the routing rules to route the requests served by the gateway. Each gateway reference should match the pattern: `projects/*/locations/global/gateways/`
* */
*/
public val gateways: Output>
get() = javaResource.gateways().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* Optional. Set of label tags associated with the TcpRoute resource.
*/
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy