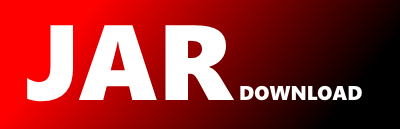
com.pulumi.googlenative.osconfig.v1alpha.kotlin.OsPolicyAssignment.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.osconfig.v1alpha.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.osconfig.v1alpha.kotlin.outputs.OSPolicyAssignmentInstanceFilterResponse
import com.pulumi.googlenative.osconfig.v1alpha.kotlin.outputs.OSPolicyAssignmentRolloutResponse
import com.pulumi.googlenative.osconfig.v1alpha.kotlin.outputs.OSPolicyResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.googlenative.osconfig.v1alpha.kotlin.outputs.OSPolicyAssignmentInstanceFilterResponse.Companion.toKotlin as oSPolicyAssignmentInstanceFilterResponseToKotlin
import com.pulumi.googlenative.osconfig.v1alpha.kotlin.outputs.OSPolicyAssignmentRolloutResponse.Companion.toKotlin as oSPolicyAssignmentRolloutResponseToKotlin
import com.pulumi.googlenative.osconfig.v1alpha.kotlin.outputs.OSPolicyResponse.Companion.toKotlin as oSPolicyResponseToKotlin
/**
* Builder for [OsPolicyAssignment].
*/
@PulumiTagMarker
public class OsPolicyAssignmentResourceBuilder internal constructor() {
public var name: String? = null
public var args: OsPolicyAssignmentArgs = OsPolicyAssignmentArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend OsPolicyAssignmentArgsBuilder.() -> Unit) {
val builder = OsPolicyAssignmentArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): OsPolicyAssignment {
val builtJavaResource =
com.pulumi.googlenative.osconfig.v1alpha.OsPolicyAssignment(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return OsPolicyAssignment(builtJavaResource)
}
}
/**
* Create an OS policy assignment. This method also creates the first revision of the OS policy assignment. This method returns a long running operation (LRO) that contains the rollout details. The rollout can be cancelled by cancelling the LRO. For more information, see [Method: projects.locations.osPolicyAssignments.operations.cancel](https://cloud.google.com/compute/docs/osconfig/rest/v1alpha/projects.locations.osPolicyAssignments.operations/cancel).
*/
public class OsPolicyAssignment internal constructor(
override val javaResource: com.pulumi.googlenative.osconfig.v1alpha.OsPolicyAssignment,
) : KotlinCustomResource(javaResource, OsPolicyAssignmentMapper) {
/**
* Indicates that this revision has been successfully rolled out in this zone and new VMs will be assigned OS policies from this revision. For a given OS policy assignment, there is only one revision with a value of `true` for this field.
*/
public val baseline: Output
get() = javaResource.baseline().applyValue({ args0 -> args0 })
/**
* Indicates that this revision deletes the OS policy assignment.
*/
public val deleted: Output
get() = javaResource.deleted().applyValue({ args0 -> args0 })
/**
* OS policy assignment description. Length of the description is limited to 1024 characters.
*/
public val description: Output
get() = javaResource.description().applyValue({ args0 -> args0 })
/**
* The etag for this OS policy assignment. If this is provided on update, it must match the server's etag.
*/
public val etag: Output
get() = javaResource.etag().applyValue({ args0 -> args0 })
/**
* Filter to select VMs.
*/
public val instanceFilter: Output
get() = javaResource.instanceFilter().applyValue({ args0 ->
args0.let({ args0 ->
oSPolicyAssignmentInstanceFilterResponseToKotlin(args0)
})
})
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* Resource name. Format: `projects/{project_number}/locations/{location}/osPolicyAssignments/{os_policy_assignment_id}` This field is ignored when you create an OS policy assignment.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* List of OS policies to be applied to the VMs.
*/
public val osPolicies: Output>
get() = javaResource.osPolicies().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
oSPolicyResponseToKotlin(args0)
})
})
})
/**
* Required. The logical name of the OS policy assignment in the project with the following restrictions: * Must contain only lowercase letters, numbers, and hyphens. * Must start with a letter. * Must be between 1-63 characters. * Must end with a number or a letter. * Must be unique within the project.
*/
public val osPolicyAssignmentId: Output
get() = javaResource.osPolicyAssignmentId().applyValue({ args0 -> args0 })
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* Indicates that reconciliation is in progress for the revision. This value is `true` when the `rollout_state` is one of: * IN_PROGRESS * CANCELLING
*/
public val reconciling: Output
get() = javaResource.reconciling().applyValue({ args0 -> args0 })
/**
* The timestamp that the revision was created.
*/
public val revisionCreateTime: Output
get() = javaResource.revisionCreateTime().applyValue({ args0 -> args0 })
/**
* The assignment revision ID A new revision is committed whenever a rollout is triggered for a OS policy assignment
*/
public val revisionId: Output
get() = javaResource.revisionId().applyValue({ args0 -> args0 })
/**
* Rollout to deploy the OS policy assignment. A rollout is triggered in the following situations: 1) OSPolicyAssignment is created. 2) OSPolicyAssignment is updated and the update contains changes to one of the following fields: - instance_filter - os_policies 3) OSPolicyAssignment is deleted.
*/
public val rollout: Output
get() = javaResource.rollout().applyValue({ args0 ->
args0.let({ args0 ->
oSPolicyAssignmentRolloutResponseToKotlin(args0)
})
})
/**
* OS policy assignment rollout state
*/
public val rolloutState: Output
get() = javaResource.rolloutState().applyValue({ args0 -> args0 })
/**
* Server generated unique id for the OS policy assignment resource.
*/
public val uid: Output
get() = javaResource.uid().applyValue({ args0 -> args0 })
}
public object OsPolicyAssignmentMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.googlenative.osconfig.v1alpha.OsPolicyAssignment::class == javaResource::class
override fun map(javaResource: Resource): OsPolicyAssignment = OsPolicyAssignment(
javaResource as
com.pulumi.googlenative.osconfig.v1alpha.OsPolicyAssignment,
)
}
/**
* @see [OsPolicyAssignment].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [OsPolicyAssignment].
*/
public suspend fun osPolicyAssignment(
name: String,
block: suspend OsPolicyAssignmentResourceBuilder.() -> Unit,
): OsPolicyAssignment {
val builder = OsPolicyAssignmentResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [OsPolicyAssignment].
* @param name The _unique_ name of the resulting resource.
*/
public fun osPolicyAssignment(name: String): OsPolicyAssignment {
val builder = OsPolicyAssignmentResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy