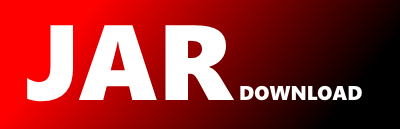
com.pulumi.googlenative.pubsub.v1beta2.kotlin.Subscription.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.pubsub.v1beta2.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.pubsub.v1beta2.kotlin.outputs.PushConfigResponse
import com.pulumi.googlenative.pubsub.v1beta2.kotlin.outputs.PushConfigResponse.Companion.toKotlin
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [Subscription].
*/
@PulumiTagMarker
public class SubscriptionResourceBuilder internal constructor() {
public var name: String? = null
public var args: SubscriptionArgs = SubscriptionArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend SubscriptionArgsBuilder.() -> Unit) {
val builder = SubscriptionArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Subscription {
val builtJavaResource =
com.pulumi.googlenative.pubsub.v1beta2.Subscription(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Subscription(builtJavaResource)
}
}
/**
* Creates a subscription to a given topic. If the subscription already exists, returns `ALREADY_EXISTS`. If the corresponding topic doesn't exist, returns `NOT_FOUND`. If the name is not provided in the request, the server will assign a random name for this subscription on the same project as the topic. Note that for REST API requests, you must specify a name.
*/
public class Subscription internal constructor(
override val javaResource: com.pulumi.googlenative.pubsub.v1beta2.Subscription,
) : KotlinCustomResource(javaResource, SubscriptionMapper) {
/**
* This value is the maximum time after a subscriber receives a message before the subscriber should acknowledge the message. After message delivery but before the ack deadline expires and before the message is acknowledged, it is an outstanding message and will not be delivered again during that time (on a best-effort basis). For pull subscriptions, this value is used as the initial value for the ack deadline. To override this value for a given message, call `ModifyAckDeadline` with the corresponding `ack_id` if using pull. The maximum custom deadline you can specify is 600 seconds (10 minutes). For push delivery, this value is also used to set the request timeout for the call to the push endpoint. If the subscriber never acknowledges the message, the Pub/Sub system will eventually redeliver the message. If this parameter is 0, a default value of 10 seconds is used.
*/
public val ackDeadlineSeconds: Output
get() = javaResource.ackDeadlineSeconds().applyValue({ args0 -> args0 })
/**
* The name of the subscription. It must have the format `"projects/{project}/subscriptions/{subscription}"`. `{subscription}` must start with a letter, and contain only letters (`[A-Za-z]`), numbers (`[0-9]`), dashes (`-`), underscores (`_`), periods (`.`), tildes (`~`), plus (`+`) or percent signs (`%`). It must be between 3 and 255 characters in length, and it must not start with `"goog"`.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* If push delivery is used with this subscription, this field is used to configure it. An empty `pushConfig` signifies that the subscriber will pull and ack messages using API methods.
*/
public val pushConfig: Output
get() = javaResource.pushConfig().applyValue({ args0 -> args0.let({ args0 -> toKotlin(args0) }) })
public val subscriptionId: Output
get() = javaResource.subscriptionId().applyValue({ args0 -> args0 })
/**
* The name of the topic from which this subscription is receiving messages. The value of this field will be `_deleted-topic_` if the topic has been deleted.
*/
public val topic: Output
get() = javaResource.topic().applyValue({ args0 -> args0 })
}
public object SubscriptionMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.googlenative.pubsub.v1beta2.Subscription::class == javaResource::class
override fun map(javaResource: Resource): Subscription = Subscription(
javaResource as
com.pulumi.googlenative.pubsub.v1beta2.Subscription,
)
}
/**
* @see [Subscription].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Subscription].
*/
public suspend fun subscription(
name: String,
block: suspend SubscriptionResourceBuilder.() -> Unit,
): Subscription {
val builder = SubscriptionResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Subscription].
* @param name The _unique_ name of the resulting resource.
*/
public fun subscription(name: String): Subscription {
val builder = SubscriptionResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy