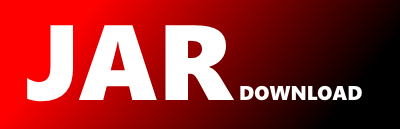
com.pulumi.googlenative.retail.v2alpha.kotlin.ModelArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.retail.v2alpha.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.retail.v2alpha.ModelArgs.builder
import com.pulumi.googlenative.retail.v2alpha.kotlin.enums.ModelFilteringOption
import com.pulumi.googlenative.retail.v2alpha.kotlin.enums.ModelPeriodicTuningState
import com.pulumi.googlenative.retail.v2alpha.kotlin.enums.ModelTrainingState
import com.pulumi.googlenative.retail.v2alpha.kotlin.inputs.GoogleCloudRetailV2alphaModelModelFeaturesConfigArgs
import com.pulumi.googlenative.retail.v2alpha.kotlin.inputs.GoogleCloudRetailV2alphaModelModelFeaturesConfigArgsBuilder
import com.pulumi.googlenative.retail.v2alpha.kotlin.inputs.GoogleCloudRetailV2alphaModelPageOptimizationConfigArgs
import com.pulumi.googlenative.retail.v2alpha.kotlin.inputs.GoogleCloudRetailV2alphaModelPageOptimizationConfigArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Creates a new model.
* @property catalogId
* @property displayName The display name of the model. Should be human readable, used to display Recommendation Models in the Retail Cloud Console Dashboard. UTF-8 encoded string with limit of 1024 characters.
* @property dryRun Optional. Whether to run a dry run to validate the request (without actually creating the model).
* @property filteringOption Optional. If `RECOMMENDATIONS_FILTERING_ENABLED`, recommendation filtering by attributes is enabled for the model.
* @property location
* @property modelFeaturesConfig Optional. Additional model features config.
* @property name The fully qualified resource name of the model. Format: `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}` catalog_id has char limit of 50. recommendation_model_id has char limit of 40.
* @property optimizationObjective Optional. The optimization objective e.g. `cvr`. Currently supported values: `ctr`, `cvr`, `revenue-per-order`. If not specified, we choose default based on model type. Default depends on type of recommendation: `recommended-for-you` => `ctr` `others-you-may-like` => `ctr` `frequently-bought-together` => `revenue_per_order` This field together with optimization_objective describe model metadata to use to control model training and serving. See https://cloud.google.com/retail/docs/models for more details on what the model metadata control and which combination of parameters are valid. For invalid combinations of parameters (e.g. type = `frequently-bought-together` and optimization_objective = `ctr`), you receive an error 400 if you try to create/update a recommendation with this set of knobs.
* @property pageOptimizationConfig Optional. The page optimization config.
* @property periodicTuningState Optional. The state of periodic tuning. The period we use is 3 months - to do a one-off tune earlier use the `TuneModel` method. Default value is `PERIODIC_TUNING_ENABLED`.
* @property project
* @property trainingState Optional. The training state that the model is in (e.g. `TRAINING` or `PAUSED`). Since part of the cost of running the service is frequency of training - this can be used to determine when to train model in order to control cost. If not specified: the default value for `CreateModel` method is `TRAINING`. The default value for `UpdateModel` method is to keep the state the same as before.
* @property type The type of model e.g. `home-page`. Currently supported values: `recommended-for-you`, `others-you-may-like`, `frequently-bought-together`, `page-optimization`, `similar-items`, `buy-it-again`, `on-sale-items`, and `recently-viewed`(readonly value). This field together with optimization_objective describe model metadata to use to control model training and serving. See https://cloud.google.com/retail/docs/models for more details on what the model metadata control and which combination of parameters are valid. For invalid combinations of parameters (e.g. type = `frequently-bought-together` and optimization_objective = `ctr`), you receive an error 400 if you try to create/update a recommendation with this set of knobs.
*/
public data class ModelArgs(
public val catalogId: Output? = null,
public val displayName: Output? = null,
public val dryRun: Output? = null,
public val filteringOption: Output? = null,
public val location: Output? = null,
public val modelFeaturesConfig: Output? =
null,
public val name: Output? = null,
public val optimizationObjective: Output? = null,
public val pageOptimizationConfig: Output? = null,
public val periodicTuningState: Output? = null,
public val project: Output? = null,
public val trainingState: Output? = null,
public val type: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.retail.v2alpha.ModelArgs =
com.pulumi.googlenative.retail.v2alpha.ModelArgs.builder()
.catalogId(catalogId?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.dryRun(dryRun?.applyValue({ args0 -> args0 }))
.filteringOption(filteringOption?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.location(location?.applyValue({ args0 -> args0 }))
.modelFeaturesConfig(
modelFeaturesConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.name(name?.applyValue({ args0 -> args0 }))
.optimizationObjective(optimizationObjective?.applyValue({ args0 -> args0 }))
.pageOptimizationConfig(
pageOptimizationConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.periodicTuningState(
periodicTuningState?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.project(project?.applyValue({ args0 -> args0 }))
.trainingState(trainingState?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.type(type?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ModelArgs].
*/
@PulumiTagMarker
public class ModelArgsBuilder internal constructor() {
private var catalogId: Output? = null
private var displayName: Output? = null
private var dryRun: Output? = null
private var filteringOption: Output? = null
private var location: Output? = null
private var modelFeaturesConfig: Output? =
null
private var name: Output? = null
private var optimizationObjective: Output? = null
private var pageOptimizationConfig:
Output? = null
private var periodicTuningState: Output? = null
private var project: Output? = null
private var trainingState: Output? = null
private var type: Output? = null
/**
* @param value
*/
@JvmName("tckouvwifdyrxkbo")
public suspend fun catalogId(`value`: Output) {
this.catalogId = value
}
/**
* @param value The display name of the model. Should be human readable, used to display Recommendation Models in the Retail Cloud Console Dashboard. UTF-8 encoded string with limit of 1024 characters.
*/
@JvmName("xafywfhyvbnlpwfp")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value Optional. Whether to run a dry run to validate the request (without actually creating the model).
*/
@JvmName("mrppsiwijseryfxm")
public suspend fun dryRun(`value`: Output) {
this.dryRun = value
}
/**
* @param value Optional. If `RECOMMENDATIONS_FILTERING_ENABLED`, recommendation filtering by attributes is enabled for the model.
*/
@JvmName("avaefgnjtupotsam")
public suspend fun filteringOption(`value`: Output) {
this.filteringOption = value
}
/**
* @param value
*/
@JvmName("rdvxcaiaomvvdevm")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value Optional. Additional model features config.
*/
@JvmName("luiqqbrwfomccnqp")
public suspend fun modelFeaturesConfig(`value`: Output) {
this.modelFeaturesConfig = value
}
/**
* @param value The fully qualified resource name of the model. Format: `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}` catalog_id has char limit of 50. recommendation_model_id has char limit of 40.
*/
@JvmName("ckbdwinvkfxsvaiw")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Optional. The optimization objective e.g. `cvr`. Currently supported values: `ctr`, `cvr`, `revenue-per-order`. If not specified, we choose default based on model type. Default depends on type of recommendation: `recommended-for-you` => `ctr` `others-you-may-like` => `ctr` `frequently-bought-together` => `revenue_per_order` This field together with optimization_objective describe model metadata to use to control model training and serving. See https://cloud.google.com/retail/docs/models for more details on what the model metadata control and which combination of parameters are valid. For invalid combinations of parameters (e.g. type = `frequently-bought-together` and optimization_objective = `ctr`), you receive an error 400 if you try to create/update a recommendation with this set of knobs.
*/
@JvmName("thcpwvjdlefvjals")
public suspend fun optimizationObjective(`value`: Output) {
this.optimizationObjective = value
}
/**
* @param value Optional. The page optimization config.
*/
@JvmName("dsvhlfaasrygaypn")
public suspend fun pageOptimizationConfig(`value`: Output) {
this.pageOptimizationConfig = value
}
/**
* @param value Optional. The state of periodic tuning. The period we use is 3 months - to do a one-off tune earlier use the `TuneModel` method. Default value is `PERIODIC_TUNING_ENABLED`.
*/
@JvmName("myndbnosppykfcwy")
public suspend fun periodicTuningState(`value`: Output) {
this.periodicTuningState = value
}
/**
* @param value
*/
@JvmName("unipccbotnjdsxkb")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value Optional. The training state that the model is in (e.g. `TRAINING` or `PAUSED`). Since part of the cost of running the service is frequency of training - this can be used to determine when to train model in order to control cost. If not specified: the default value for `CreateModel` method is `TRAINING`. The default value for `UpdateModel` method is to keep the state the same as before.
*/
@JvmName("xorhfmbgmyolrmbi")
public suspend fun trainingState(`value`: Output) {
this.trainingState = value
}
/**
* @param value The type of model e.g. `home-page`. Currently supported values: `recommended-for-you`, `others-you-may-like`, `frequently-bought-together`, `page-optimization`, `similar-items`, `buy-it-again`, `on-sale-items`, and `recently-viewed`(readonly value). This field together with optimization_objective describe model metadata to use to control model training and serving. See https://cloud.google.com/retail/docs/models for more details on what the model metadata control and which combination of parameters are valid. For invalid combinations of parameters (e.g. type = `frequently-bought-together` and optimization_objective = `ctr`), you receive an error 400 if you try to create/update a recommendation with this set of knobs.
*/
@JvmName("ftbhhadghtucqbit")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value
*/
@JvmName("nyhapdgvnsjyvvcx")
public suspend fun catalogId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.catalogId = mapped
}
/**
* @param value The display name of the model. Should be human readable, used to display Recommendation Models in the Retail Cloud Console Dashboard. UTF-8 encoded string with limit of 1024 characters.
*/
@JvmName("bgayuwmsourfapux")
public suspend fun displayName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.displayName = mapped
}
/**
* @param value Optional. Whether to run a dry run to validate the request (without actually creating the model).
*/
@JvmName("lxnqhurjvpddxcje")
public suspend fun dryRun(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dryRun = mapped
}
/**
* @param value Optional. If `RECOMMENDATIONS_FILTERING_ENABLED`, recommendation filtering by attributes is enabled for the model.
*/
@JvmName("cmfghvitfxiltvuy")
public suspend fun filteringOption(`value`: ModelFilteringOption?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.filteringOption = mapped
}
/**
* @param value
*/
@JvmName("ertakncpheycytqi")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value Optional. Additional model features config.
*/
@JvmName("jxwarvmykitvhile")
public suspend fun modelFeaturesConfig(`value`: GoogleCloudRetailV2alphaModelModelFeaturesConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.modelFeaturesConfig = mapped
}
/**
* @param argument Optional. Additional model features config.
*/
@JvmName("mlxhnobojoxuekkg")
public suspend fun modelFeaturesConfig(argument: suspend GoogleCloudRetailV2alphaModelModelFeaturesConfigArgsBuilder.() -> Unit) {
val toBeMapped = GoogleCloudRetailV2alphaModelModelFeaturesConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.modelFeaturesConfig = mapped
}
/**
* @param value The fully qualified resource name of the model. Format: `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}` catalog_id has char limit of 50. recommendation_model_id has char limit of 40.
*/
@JvmName("sjhnbifyvoyonten")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Optional. The optimization objective e.g. `cvr`. Currently supported values: `ctr`, `cvr`, `revenue-per-order`. If not specified, we choose default based on model type. Default depends on type of recommendation: `recommended-for-you` => `ctr` `others-you-may-like` => `ctr` `frequently-bought-together` => `revenue_per_order` This field together with optimization_objective describe model metadata to use to control model training and serving. See https://cloud.google.com/retail/docs/models for more details on what the model metadata control and which combination of parameters are valid. For invalid combinations of parameters (e.g. type = `frequently-bought-together` and optimization_objective = `ctr`), you receive an error 400 if you try to create/update a recommendation with this set of knobs.
*/
@JvmName("yykupqttawfxgfdh")
public suspend fun optimizationObjective(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.optimizationObjective = mapped
}
/**
* @param value Optional. The page optimization config.
*/
@JvmName("jukohdxbqoxtdnyf")
public suspend fun pageOptimizationConfig(`value`: GoogleCloudRetailV2alphaModelPageOptimizationConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pageOptimizationConfig = mapped
}
/**
* @param argument Optional. The page optimization config.
*/
@JvmName("dbwnjfrxalaqchrt")
public suspend fun pageOptimizationConfig(argument: suspend GoogleCloudRetailV2alphaModelPageOptimizationConfigArgsBuilder.() -> Unit) {
val toBeMapped = GoogleCloudRetailV2alphaModelPageOptimizationConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.pageOptimizationConfig = mapped
}
/**
* @param value Optional. The state of periodic tuning. The period we use is 3 months - to do a one-off tune earlier use the `TuneModel` method. Default value is `PERIODIC_TUNING_ENABLED`.
*/
@JvmName("uremrpjxkhoaffra")
public suspend fun periodicTuningState(`value`: ModelPeriodicTuningState?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.periodicTuningState = mapped
}
/**
* @param value
*/
@JvmName("qftambrnvskxdhkm")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value Optional. The training state that the model is in (e.g. `TRAINING` or `PAUSED`). Since part of the cost of running the service is frequency of training - this can be used to determine when to train model in order to control cost. If not specified: the default value for `CreateModel` method is `TRAINING`. The default value for `UpdateModel` method is to keep the state the same as before.
*/
@JvmName("cdkqojjodqoweurg")
public suspend fun trainingState(`value`: ModelTrainingState?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.trainingState = mapped
}
/**
* @param value The type of model e.g. `home-page`. Currently supported values: `recommended-for-you`, `others-you-may-like`, `frequently-bought-together`, `page-optimization`, `similar-items`, `buy-it-again`, `on-sale-items`, and `recently-viewed`(readonly value). This field together with optimization_objective describe model metadata to use to control model training and serving. See https://cloud.google.com/retail/docs/models for more details on what the model metadata control and which combination of parameters are valid. For invalid combinations of parameters (e.g. type = `frequently-bought-together` and optimization_objective = `ctr`), you receive an error 400 if you try to create/update a recommendation with this set of knobs.
*/
@JvmName("mwwfxtfxdqjiygrg")
public suspend fun type(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): ModelArgs = ModelArgs(
catalogId = catalogId,
displayName = displayName,
dryRun = dryRun,
filteringOption = filteringOption,
location = location,
modelFeaturesConfig = modelFeaturesConfig,
name = name,
optimizationObjective = optimizationObjective,
pageOptimizationConfig = pageOptimizationConfig,
periodicTuningState = periodicTuningState,
project = project,
trainingState = trainingState,
type = type,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy