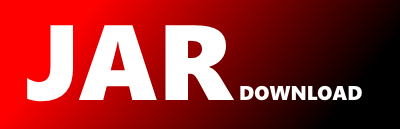
com.pulumi.googlenative.retail.v2beta.kotlin.Retail_v2betaFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.retail.v2beta.kotlin
import com.pulumi.googlenative.retail.v2beta.Retail_v2betaFunctions.getControlPlain
import com.pulumi.googlenative.retail.v2beta.Retail_v2betaFunctions.getModelPlain
import com.pulumi.googlenative.retail.v2beta.Retail_v2betaFunctions.getProductPlain
import com.pulumi.googlenative.retail.v2beta.Retail_v2betaFunctions.getServingConfigPlain
import com.pulumi.googlenative.retail.v2beta.kotlin.inputs.GetControlPlainArgs
import com.pulumi.googlenative.retail.v2beta.kotlin.inputs.GetControlPlainArgsBuilder
import com.pulumi.googlenative.retail.v2beta.kotlin.inputs.GetModelPlainArgs
import com.pulumi.googlenative.retail.v2beta.kotlin.inputs.GetModelPlainArgsBuilder
import com.pulumi.googlenative.retail.v2beta.kotlin.inputs.GetProductPlainArgs
import com.pulumi.googlenative.retail.v2beta.kotlin.inputs.GetProductPlainArgsBuilder
import com.pulumi.googlenative.retail.v2beta.kotlin.inputs.GetServingConfigPlainArgs
import com.pulumi.googlenative.retail.v2beta.kotlin.inputs.GetServingConfigPlainArgsBuilder
import com.pulumi.googlenative.retail.v2beta.kotlin.outputs.GetControlResult
import com.pulumi.googlenative.retail.v2beta.kotlin.outputs.GetModelResult
import com.pulumi.googlenative.retail.v2beta.kotlin.outputs.GetProductResult
import com.pulumi.googlenative.retail.v2beta.kotlin.outputs.GetServingConfigResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.googlenative.retail.v2beta.kotlin.outputs.GetControlResult.Companion.toKotlin as getControlResultToKotlin
import com.pulumi.googlenative.retail.v2beta.kotlin.outputs.GetModelResult.Companion.toKotlin as getModelResultToKotlin
import com.pulumi.googlenative.retail.v2beta.kotlin.outputs.GetProductResult.Companion.toKotlin as getProductResultToKotlin
import com.pulumi.googlenative.retail.v2beta.kotlin.outputs.GetServingConfigResult.Companion.toKotlin as getServingConfigResultToKotlin
public object Retail_v2betaFunctions {
/**
* Gets a Control.
* @param argument null
* @return null
*/
public suspend fun getControl(argument: GetControlPlainArgs): GetControlResult =
getControlResultToKotlin(getControlPlain(argument.toJava()).await())
/**
* @see [getControl].
* @param catalogId
* @param controlId
* @param location
* @param project
* @return null
*/
public suspend fun getControl(
catalogId: String,
controlId: String,
location: String,
project: String? = null,
): GetControlResult {
val argument = GetControlPlainArgs(
catalogId = catalogId,
controlId = controlId,
location = location,
project = project,
)
return getControlResultToKotlin(getControlPlain(argument.toJava()).await())
}
/**
* @see [getControl].
* @param argument Builder for [com.pulumi.googlenative.retail.v2beta.kotlin.inputs.GetControlPlainArgs].
* @return null
*/
public suspend fun getControl(argument: suspend GetControlPlainArgsBuilder.() -> Unit): GetControlResult {
val builder = GetControlPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getControlResultToKotlin(getControlPlain(builtArgument.toJava()).await())
}
/**
* Gets a model.
* @param argument null
* @return null
*/
public suspend fun getModel(argument: GetModelPlainArgs): GetModelResult =
getModelResultToKotlin(getModelPlain(argument.toJava()).await())
/**
* @see [getModel].
* @param catalogId
* @param location
* @param modelId
* @param project
* @return null
*/
public suspend fun getModel(
catalogId: String,
location: String,
modelId: String,
project: String? = null,
): GetModelResult {
val argument = GetModelPlainArgs(
catalogId = catalogId,
location = location,
modelId = modelId,
project = project,
)
return getModelResultToKotlin(getModelPlain(argument.toJava()).await())
}
/**
* @see [getModel].
* @param argument Builder for [com.pulumi.googlenative.retail.v2beta.kotlin.inputs.GetModelPlainArgs].
* @return null
*/
public suspend fun getModel(argument: suspend GetModelPlainArgsBuilder.() -> Unit): GetModelResult {
val builder = GetModelPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getModelResultToKotlin(getModelPlain(builtArgument.toJava()).await())
}
/**
* Gets a Product.
* @param argument null
* @return null
*/
public suspend fun getProduct(argument: GetProductPlainArgs): GetProductResult =
getProductResultToKotlin(getProductPlain(argument.toJava()).await())
/**
* @see [getProduct].
* @param branchId
* @param catalogId
* @param location
* @param productId
* @param project
* @return null
*/
public suspend fun getProduct(
branchId: String,
catalogId: String,
location: String,
productId: String,
project: String? = null,
): GetProductResult {
val argument = GetProductPlainArgs(
branchId = branchId,
catalogId = catalogId,
location = location,
productId = productId,
project = project,
)
return getProductResultToKotlin(getProductPlain(argument.toJava()).await())
}
/**
* @see [getProduct].
* @param argument Builder for [com.pulumi.googlenative.retail.v2beta.kotlin.inputs.GetProductPlainArgs].
* @return null
*/
public suspend fun getProduct(argument: suspend GetProductPlainArgsBuilder.() -> Unit): GetProductResult {
val builder = GetProductPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getProductResultToKotlin(getProductPlain(builtArgument.toJava()).await())
}
/**
* Gets a ServingConfig. Returns a NotFound error if the ServingConfig does not exist.
* @param argument null
* @return null
*/
public suspend fun getServingConfig(argument: GetServingConfigPlainArgs): GetServingConfigResult =
getServingConfigResultToKotlin(getServingConfigPlain(argument.toJava()).await())
/**
* @see [getServingConfig].
* @param catalogId
* @param location
* @param project
* @param servingConfigId
* @return null
*/
public suspend fun getServingConfig(
catalogId: String,
location: String,
project: String? = null,
servingConfigId: String,
): GetServingConfigResult {
val argument = GetServingConfigPlainArgs(
catalogId = catalogId,
location = location,
project = project,
servingConfigId = servingConfigId,
)
return getServingConfigResultToKotlin(getServingConfigPlain(argument.toJava()).await())
}
/**
* @see [getServingConfig].
* @param argument Builder for [com.pulumi.googlenative.retail.v2beta.kotlin.inputs.GetServingConfigPlainArgs].
* @return null
*/
public suspend fun getServingConfig(argument: suspend GetServingConfigPlainArgsBuilder.() -> Unit): GetServingConfigResult {
val builder = GetServingConfigPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getServingConfigResultToKotlin(getServingConfigPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy