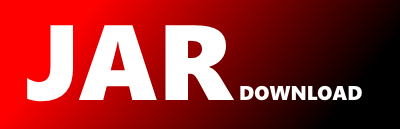
com.pulumi.googlenative.runtimeconfig.v1beta1.kotlin.ConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.runtimeconfig.v1beta1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.runtimeconfig.v1beta1.ConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Creates a new RuntimeConfig resource. The configuration name must be unique within project.
* @property description An optional description of the RuntimeConfig object.
* @property name The resource name of a runtime config. The name must have the format: projects/[PROJECT_ID]/configs/[CONFIG_NAME] The `[PROJECT_ID]` must be a valid project ID, and `[CONFIG_NAME]` is an arbitrary name that matches the `[0-9A-Za-z](?:[_.A-Za-z0-9-]{0,62}[_.A-Za-z0-9])?` regular expression. The length of `[CONFIG_NAME]` must be less than 64 characters. You pick the RuntimeConfig resource name, but the server will validate that the name adheres to this format. After you create the resource, you cannot change the resource's name.
* @property project
* @property requestId An optional but recommended unique `request_id`. If the server receives two `create()` requests with the same `request_id`, then the second request will be ignored and the first resource created and stored in the backend is returned. Empty `request_id` fields are ignored. It is responsibility of the client to ensure uniqueness of the `request_id` strings. `request_id` strings are limited to 64 characters.
*/
public data class ConfigArgs(
public val description: Output? = null,
public val name: Output? = null,
public val project: Output? = null,
public val requestId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.runtimeconfig.v1beta1.ConfigArgs =
com.pulumi.googlenative.runtimeconfig.v1beta1.ConfigArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.requestId(requestId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ConfigArgs].
*/
@PulumiTagMarker
public class ConfigArgsBuilder internal constructor() {
private var description: Output? = null
private var name: Output? = null
private var project: Output? = null
private var requestId: Output? = null
/**
* @param value An optional description of the RuntimeConfig object.
*/
@JvmName("lrxebxqhichpnbfu")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The resource name of a runtime config. The name must have the format: projects/[PROJECT_ID]/configs/[CONFIG_NAME] The `[PROJECT_ID]` must be a valid project ID, and `[CONFIG_NAME]` is an arbitrary name that matches the `[0-9A-Za-z](?:[_.A-Za-z0-9-]{0,62}[_.A-Za-z0-9])?` regular expression. The length of `[CONFIG_NAME]` must be less than 64 characters. You pick the RuntimeConfig resource name, but the server will validate that the name adheres to this format. After you create the resource, you cannot change the resource's name.
*/
@JvmName("tojyjqrfiljqpoqo")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value
*/
@JvmName("oqrobuuknnqsiwkc")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value An optional but recommended unique `request_id`. If the server receives two `create()` requests with the same `request_id`, then the second request will be ignored and the first resource created and stored in the backend is returned. Empty `request_id` fields are ignored. It is responsibility of the client to ensure uniqueness of the `request_id` strings. `request_id` strings are limited to 64 characters.
*/
@JvmName("vslonapnncxbkair")
public suspend fun requestId(`value`: Output) {
this.requestId = value
}
/**
* @param value An optional description of the RuntimeConfig object.
*/
@JvmName("gocpnocxjttsuocm")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value The resource name of a runtime config. The name must have the format: projects/[PROJECT_ID]/configs/[CONFIG_NAME] The `[PROJECT_ID]` must be a valid project ID, and `[CONFIG_NAME]` is an arbitrary name that matches the `[0-9A-Za-z](?:[_.A-Za-z0-9-]{0,62}[_.A-Za-z0-9])?` regular expression. The length of `[CONFIG_NAME]` must be less than 64 characters. You pick the RuntimeConfig resource name, but the server will validate that the name adheres to this format. After you create the resource, you cannot change the resource's name.
*/
@JvmName("soshcsjaxmpttifo")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value
*/
@JvmName("btdwcpqldcoectlo")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value An optional but recommended unique `request_id`. If the server receives two `create()` requests with the same `request_id`, then the second request will be ignored and the first resource created and stored in the backend is returned. Empty `request_id` fields are ignored. It is responsibility of the client to ensure uniqueness of the `request_id` strings. `request_id` strings are limited to 64 characters.
*/
@JvmName("rccrgufrtjgyobxg")
public suspend fun requestId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requestId = mapped
}
internal fun build(): ConfigArgs = ConfigArgs(
description = description,
name = name,
project = project,
requestId = requestId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy