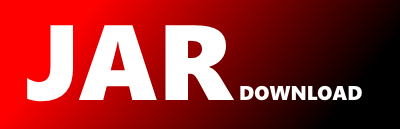
com.pulumi.googlenative.runtimeconfig.v1beta1.kotlin.WaiterArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.runtimeconfig.v1beta1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.runtimeconfig.v1beta1.WaiterArgs.builder
import com.pulumi.googlenative.runtimeconfig.v1beta1.kotlin.inputs.EndConditionArgs
import com.pulumi.googlenative.runtimeconfig.v1beta1.kotlin.inputs.EndConditionArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Creates a Waiter resource. This operation returns a long-running Operation resource which can be polled for completion. However, a waiter with the given name will exist (and can be retrieved) prior to the operation completing. If the operation fails, the failed Waiter resource will still exist and must be deleted prior to subsequent creation attempts.
* @property configId
* @property failure [Optional] The failure condition of this waiter. If this condition is met, `done` will be set to `true` and the `error` code will be set to `ABORTED`. The failure condition takes precedence over the success condition. If both conditions are met, a failure will be indicated. This value is optional; if no failure condition is set, the only failure scenario will be a timeout.
* @property name The name of the Waiter resource, in the format: projects/[PROJECT_ID]/configs/[CONFIG_NAME]/waiters/[WAITER_NAME] The `[PROJECT_ID]` must be a valid Google Cloud project ID, the `[CONFIG_NAME]` must be a valid RuntimeConfig resource, the `[WAITER_NAME]` must match RFC 1035 segment specification, and the length of `[WAITER_NAME]` must be less than 64 bytes. After you create a Waiter resource, you cannot change the resource name.
* @property project
* @property requestId An optional but recommended unique `request_id`. If the server receives two `create()` requests with the same `request_id`, then the second request will be ignored and the first resource created and stored in the backend is returned. Empty `request_id` fields are ignored. It is responsibility of the client to ensure uniqueness of the `request_id` strings. `request_id` strings are limited to 64 characters.
* @property success [Required] The success condition. If this condition is met, `done` will be set to `true` and the `error` value will remain unset. The failure condition takes precedence over the success condition. If both conditions are met, a failure will be indicated.
* @property timeout [Required] Specifies the timeout of the waiter in seconds, beginning from the instant that `waiters().create` method is called. If this time elapses before the success or failure conditions are met, the waiter fails and sets the `error` code to `DEADLINE_EXCEEDED`.
*/
public data class WaiterArgs(
public val configId: Output? = null,
public val failure: Output? = null,
public val name: Output? = null,
public val project: Output? = null,
public val requestId: Output? = null,
public val success: Output? = null,
public val timeout: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.runtimeconfig.v1beta1.WaiterArgs =
com.pulumi.googlenative.runtimeconfig.v1beta1.WaiterArgs.builder()
.configId(configId?.applyValue({ args0 -> args0 }))
.failure(failure?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.requestId(requestId?.applyValue({ args0 -> args0 }))
.success(success?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.timeout(timeout?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [WaiterArgs].
*/
@PulumiTagMarker
public class WaiterArgsBuilder internal constructor() {
private var configId: Output? = null
private var failure: Output? = null
private var name: Output? = null
private var project: Output? = null
private var requestId: Output? = null
private var success: Output? = null
private var timeout: Output? = null
/**
* @param value
*/
@JvmName("nisbremdltgxobjm")
public suspend fun configId(`value`: Output) {
this.configId = value
}
/**
* @param value [Optional] The failure condition of this waiter. If this condition is met, `done` will be set to `true` and the `error` code will be set to `ABORTED`. The failure condition takes precedence over the success condition. If both conditions are met, a failure will be indicated. This value is optional; if no failure condition is set, the only failure scenario will be a timeout.
*/
@JvmName("rifrxfyraoxhiddw")
public suspend fun failure(`value`: Output) {
this.failure = value
}
/**
* @param value The name of the Waiter resource, in the format: projects/[PROJECT_ID]/configs/[CONFIG_NAME]/waiters/[WAITER_NAME] The `[PROJECT_ID]` must be a valid Google Cloud project ID, the `[CONFIG_NAME]` must be a valid RuntimeConfig resource, the `[WAITER_NAME]` must match RFC 1035 segment specification, and the length of `[WAITER_NAME]` must be less than 64 bytes. After you create a Waiter resource, you cannot change the resource name.
*/
@JvmName("xvrjroppcbhqshdj")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value
*/
@JvmName("vvuhevkvpqoawdot")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value An optional but recommended unique `request_id`. If the server receives two `create()` requests with the same `request_id`, then the second request will be ignored and the first resource created and stored in the backend is returned. Empty `request_id` fields are ignored. It is responsibility of the client to ensure uniqueness of the `request_id` strings. `request_id` strings are limited to 64 characters.
*/
@JvmName("xjllmnqvglmlbbxu")
public suspend fun requestId(`value`: Output) {
this.requestId = value
}
/**
* @param value [Required] The success condition. If this condition is met, `done` will be set to `true` and the `error` value will remain unset. The failure condition takes precedence over the success condition. If both conditions are met, a failure will be indicated.
*/
@JvmName("niivspexlfliusre")
public suspend fun success(`value`: Output) {
this.success = value
}
/**
* @param value [Required] Specifies the timeout of the waiter in seconds, beginning from the instant that `waiters().create` method is called. If this time elapses before the success or failure conditions are met, the waiter fails and sets the `error` code to `DEADLINE_EXCEEDED`.
*/
@JvmName("ssfaodayuwltllnd")
public suspend fun timeout(`value`: Output) {
this.timeout = value
}
/**
* @param value
*/
@JvmName("csybeiakfxcmnfll")
public suspend fun configId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.configId = mapped
}
/**
* @param value [Optional] The failure condition of this waiter. If this condition is met, `done` will be set to `true` and the `error` code will be set to `ABORTED`. The failure condition takes precedence over the success condition. If both conditions are met, a failure will be indicated. This value is optional; if no failure condition is set, the only failure scenario will be a timeout.
*/
@JvmName("dheftljajvvvxksg")
public suspend fun failure(`value`: EndConditionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failure = mapped
}
/**
* @param argument [Optional] The failure condition of this waiter. If this condition is met, `done` will be set to `true` and the `error` code will be set to `ABORTED`. The failure condition takes precedence over the success condition. If both conditions are met, a failure will be indicated. This value is optional; if no failure condition is set, the only failure scenario will be a timeout.
*/
@JvmName("qxxuuqmecdllxggg")
public suspend fun failure(argument: suspend EndConditionArgsBuilder.() -> Unit) {
val toBeMapped = EndConditionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.failure = mapped
}
/**
* @param value The name of the Waiter resource, in the format: projects/[PROJECT_ID]/configs/[CONFIG_NAME]/waiters/[WAITER_NAME] The `[PROJECT_ID]` must be a valid Google Cloud project ID, the `[CONFIG_NAME]` must be a valid RuntimeConfig resource, the `[WAITER_NAME]` must match RFC 1035 segment specification, and the length of `[WAITER_NAME]` must be less than 64 bytes. After you create a Waiter resource, you cannot change the resource name.
*/
@JvmName("hiwlhsafempoxeci")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value
*/
@JvmName("qtknfqkxsawiacpn")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value An optional but recommended unique `request_id`. If the server receives two `create()` requests with the same `request_id`, then the second request will be ignored and the first resource created and stored in the backend is returned. Empty `request_id` fields are ignored. It is responsibility of the client to ensure uniqueness of the `request_id` strings. `request_id` strings are limited to 64 characters.
*/
@JvmName("xwrsyvunljwmwusp")
public suspend fun requestId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requestId = mapped
}
/**
* @param value [Required] The success condition. If this condition is met, `done` will be set to `true` and the `error` value will remain unset. The failure condition takes precedence over the success condition. If both conditions are met, a failure will be indicated.
*/
@JvmName("jvytvcylcwovlygn")
public suspend fun success(`value`: EndConditionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.success = mapped
}
/**
* @param argument [Required] The success condition. If this condition is met, `done` will be set to `true` and the `error` value will remain unset. The failure condition takes precedence over the success condition. If both conditions are met, a failure will be indicated.
*/
@JvmName("jnnqmvuwsuathhob")
public suspend fun success(argument: suspend EndConditionArgsBuilder.() -> Unit) {
val toBeMapped = EndConditionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.success = mapped
}
/**
* @param value [Required] Specifies the timeout of the waiter in seconds, beginning from the instant that `waiters().create` method is called. If this time elapses before the success or failure conditions are met, the waiter fails and sets the `error` code to `DEADLINE_EXCEEDED`.
*/
@JvmName("gdtxquawxdmrhijh")
public suspend fun timeout(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeout = mapped
}
internal fun build(): WaiterArgs = WaiterArgs(
configId = configId,
failure = failure,
name = name,
project = project,
requestId = requestId,
success = success,
timeout = timeout,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy