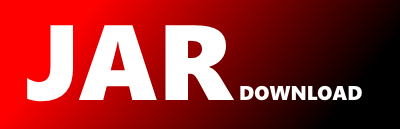
com.pulumi.googlenative.securitycenter.v1.kotlin.FolderCustomModuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.securitycenter.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.securitycenter.v1.FolderCustomModuleArgs.builder
import com.pulumi.googlenative.securitycenter.v1.kotlin.enums.FolderCustomModuleEnablementState
import com.pulumi.googlenative.securitycenter.v1.kotlin.inputs.GoogleCloudSecuritycenterV1CustomConfigArgs
import com.pulumi.googlenative.securitycenter.v1.kotlin.inputs.GoogleCloudSecuritycenterV1CustomConfigArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Creates a resident SecurityHealthAnalyticsCustomModule at the scope of the given CRM parent, and also creates inherited SecurityHealthAnalyticsCustomModules for all CRM descendants of the given parent. These modules are enabled by default.
* Auto-naming is currently not supported for this resource.
* @property customConfig The user specified custom configuration for the module.
* @property displayName The display name of the Security Health Analytics custom module. This display name becomes the finding category for all findings that are returned by this custom module. The display name must be between 1 and 128 characters, start with a lowercase letter, and contain alphanumeric characters or underscores only.
* @property enablementState The enablement state of the custom module.
* @property folderId
* @property name Immutable. The resource name of the custom module. Its format is "organizations/{organization}/securityHealthAnalyticsSettings/customModules/{customModule}", or "folders/{folder}/securityHealthAnalyticsSettings/customModules/{customModule}", or "projects/{project}/securityHealthAnalyticsSettings/customModules/{customModule}" The id {customModule} is server-generated and is not user settable. It will be a numeric id containing 1-20 digits.
*/
public data class FolderCustomModuleArgs(
public val customConfig: Output? = null,
public val displayName: Output? = null,
public val enablementState: Output? = null,
public val folderId: Output? = null,
public val name: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.securitycenter.v1.FolderCustomModuleArgs =
com.pulumi.googlenative.securitycenter.v1.FolderCustomModuleArgs.builder()
.customConfig(customConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.enablementState(enablementState?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.folderId(folderId?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FolderCustomModuleArgs].
*/
@PulumiTagMarker
public class FolderCustomModuleArgsBuilder internal constructor() {
private var customConfig: Output? = null
private var displayName: Output? = null
private var enablementState: Output? = null
private var folderId: Output? = null
private var name: Output? = null
/**
* @param value The user specified custom configuration for the module.
*/
@JvmName("jwoadcpspdbpkewh")
public suspend fun customConfig(`value`: Output) {
this.customConfig = value
}
/**
* @param value The display name of the Security Health Analytics custom module. This display name becomes the finding category for all findings that are returned by this custom module. The display name must be between 1 and 128 characters, start with a lowercase letter, and contain alphanumeric characters or underscores only.
*/
@JvmName("sdgcviloefmcpoyj")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value The enablement state of the custom module.
*/
@JvmName("huexhnyngaqxtsod")
public suspend fun enablementState(`value`: Output) {
this.enablementState = value
}
/**
* @param value
*/
@JvmName("ekrnnbhjuhqwqpae")
public suspend fun folderId(`value`: Output) {
this.folderId = value
}
/**
* @param value Immutable. The resource name of the custom module. Its format is "organizations/{organization}/securityHealthAnalyticsSettings/customModules/{customModule}", or "folders/{folder}/securityHealthAnalyticsSettings/customModules/{customModule}", or "projects/{project}/securityHealthAnalyticsSettings/customModules/{customModule}" The id {customModule} is server-generated and is not user settable. It will be a numeric id containing 1-20 digits.
*/
@JvmName("jgtosxaffjltlsmq")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The user specified custom configuration for the module.
*/
@JvmName("gafjxgsivngxwaac")
public suspend fun customConfig(`value`: GoogleCloudSecuritycenterV1CustomConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customConfig = mapped
}
/**
* @param argument The user specified custom configuration for the module.
*/
@JvmName("jhixpciypagjfitp")
public suspend fun customConfig(argument: suspend GoogleCloudSecuritycenterV1CustomConfigArgsBuilder.() -> Unit) {
val toBeMapped = GoogleCloudSecuritycenterV1CustomConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.customConfig = mapped
}
/**
* @param value The display name of the Security Health Analytics custom module. This display name becomes the finding category for all findings that are returned by this custom module. The display name must be between 1 and 128 characters, start with a lowercase letter, and contain alphanumeric characters or underscores only.
*/
@JvmName("mgjidvaelgsusubd")
public suspend fun displayName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.displayName = mapped
}
/**
* @param value The enablement state of the custom module.
*/
@JvmName("anywrttiwrnqogwr")
public suspend fun enablementState(`value`: FolderCustomModuleEnablementState?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enablementState = mapped
}
/**
* @param value
*/
@JvmName("ihpgewceumkqjkdj")
public suspend fun folderId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.folderId = mapped
}
/**
* @param value Immutable. The resource name of the custom module. Its format is "organizations/{organization}/securityHealthAnalyticsSettings/customModules/{customModule}", or "folders/{folder}/securityHealthAnalyticsSettings/customModules/{customModule}", or "projects/{project}/securityHealthAnalyticsSettings/customModules/{customModule}" The id {customModule} is server-generated and is not user settable. It will be a numeric id containing 1-20 digits.
*/
@JvmName("hndvvoqssfgwbnvp")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
internal fun build(): FolderCustomModuleArgs = FolderCustomModuleArgs(
customConfig = customConfig,
displayName = displayName,
enablementState = enablementState,
folderId = folderId,
name = name,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy