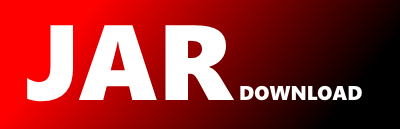
com.pulumi.googlenative.servicemanagement.v1.kotlin.RolloutArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.servicemanagement.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.servicemanagement.v1.RolloutArgs.builder
import com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.DeleteServiceStrategyArgs
import com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.DeleteServiceStrategyArgsBuilder
import com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.TrafficPercentStrategyArgs
import com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.TrafficPercentStrategyArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Creates a new service configuration rollout. Based on rollout, the Google Service Management will roll out the service configurations to different backend services. For example, the logging configuration will be pushed to Google Cloud Logging. Please note that any previous pending and running Rollouts and associated Operations will be automatically cancelled so that the latest Rollout will not be blocked by previous Rollouts. Only the 100 most recent (in any state) and the last 10 successful (if not already part of the set of 100 most recent) rollouts are kept for each service. The rest will be deleted eventually. Operation
* Auto-naming is currently not supported for this resource.
* Note - this resource's API doesn't support deletion. When deleted, the resource will persist
* on Google Cloud even though it will be deleted from Pulumi state.
* @property createTime Creation time of the rollout. Readonly.
* @property createdBy The user who created the Rollout. Readonly.
* @property deleteServiceStrategy The strategy associated with a rollout to delete a `ManagedService`. Readonly.
* @property rolloutId Optional. Unique identifier of this Rollout. Must be no longer than 63 characters and only lower case letters, digits, '.', '_' and '-' are allowed. If not specified by client, the server will generate one. The generated id will have the form of , where "date" is the create date in ISO 8601 format. "revision number" is a monotonically increasing positive number that is reset every day for each service. An example of the generated rollout_id is '2016-02-16r1'
* @property serviceName The name of the service associated with this Rollout.
* @property trafficPercentStrategy Google Service Control selects service configurations based on traffic percentage.
*/
public data class RolloutArgs(
public val createTime: Output? = null,
public val createdBy: Output? = null,
public val deleteServiceStrategy: Output? = null,
public val rolloutId: Output? = null,
public val serviceName: Output? = null,
public val trafficPercentStrategy: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.servicemanagement.v1.RolloutArgs =
com.pulumi.googlenative.servicemanagement.v1.RolloutArgs.builder()
.createTime(createTime?.applyValue({ args0 -> args0 }))
.createdBy(createdBy?.applyValue({ args0 -> args0 }))
.deleteServiceStrategy(
deleteServiceStrategy?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.rolloutId(rolloutId?.applyValue({ args0 -> args0 }))
.serviceName(serviceName?.applyValue({ args0 -> args0 }))
.trafficPercentStrategy(
trafficPercentStrategy?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [RolloutArgs].
*/
@PulumiTagMarker
public class RolloutArgsBuilder internal constructor() {
private var createTime: Output? = null
private var createdBy: Output? = null
private var deleteServiceStrategy: Output? = null
private var rolloutId: Output? = null
private var serviceName: Output? = null
private var trafficPercentStrategy: Output? = null
/**
* @param value Creation time of the rollout. Readonly.
*/
@JvmName("wucaikcvaxnrwtos")
public suspend fun createTime(`value`: Output) {
this.createTime = value
}
/**
* @param value The user who created the Rollout. Readonly.
*/
@JvmName("sxgwboxlbrayrxjs")
public suspend fun createdBy(`value`: Output) {
this.createdBy = value
}
/**
* @param value The strategy associated with a rollout to delete a `ManagedService`. Readonly.
*/
@JvmName("xhxthajulkbxldvw")
public suspend fun deleteServiceStrategy(`value`: Output) {
this.deleteServiceStrategy = value
}
/**
* @param value Optional. Unique identifier of this Rollout. Must be no longer than 63 characters and only lower case letters, digits, '.', '_' and '-' are allowed. If not specified by client, the server will generate one. The generated id will have the form of , where "date" is the create date in ISO 8601 format. "revision number" is a monotonically increasing positive number that is reset every day for each service. An example of the generated rollout_id is '2016-02-16r1'
*/
@JvmName("sxdpkwgugockqkqv")
public suspend fun rolloutId(`value`: Output) {
this.rolloutId = value
}
/**
* @param value The name of the service associated with this Rollout.
*/
@JvmName("buifbrhpogaxmohi")
public suspend fun serviceName(`value`: Output) {
this.serviceName = value
}
/**
* @param value Google Service Control selects service configurations based on traffic percentage.
*/
@JvmName("hhrbnoalklmgtopl")
public suspend fun trafficPercentStrategy(`value`: Output) {
this.trafficPercentStrategy = value
}
/**
* @param value Creation time of the rollout. Readonly.
*/
@JvmName("ormucjrwhoxonhne")
public suspend fun createTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.createTime = mapped
}
/**
* @param value The user who created the Rollout. Readonly.
*/
@JvmName("jellyhqaxjebipgw")
public suspend fun createdBy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.createdBy = mapped
}
/**
* @param value The strategy associated with a rollout to delete a `ManagedService`. Readonly.
*/
@JvmName("wcwcugpcdrhrtqpp")
public suspend fun deleteServiceStrategy(`value`: DeleteServiceStrategyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deleteServiceStrategy = mapped
}
/**
* @param argument The strategy associated with a rollout to delete a `ManagedService`. Readonly.
*/
@JvmName("efpnrvoafgagqwfl")
public suspend fun deleteServiceStrategy(argument: suspend DeleteServiceStrategyArgsBuilder.() -> Unit) {
val toBeMapped = DeleteServiceStrategyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.deleteServiceStrategy = mapped
}
/**
* @param value Optional. Unique identifier of this Rollout. Must be no longer than 63 characters and only lower case letters, digits, '.', '_' and '-' are allowed. If not specified by client, the server will generate one. The generated id will have the form of , where "date" is the create date in ISO 8601 format. "revision number" is a monotonically increasing positive number that is reset every day for each service. An example of the generated rollout_id is '2016-02-16r1'
*/
@JvmName("xhsitoxlbrujuywr")
public suspend fun rolloutId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rolloutId = mapped
}
/**
* @param value The name of the service associated with this Rollout.
*/
@JvmName("isukixqexxsbmors")
public suspend fun serviceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceName = mapped
}
/**
* @param value Google Service Control selects service configurations based on traffic percentage.
*/
@JvmName("efqlcjjjoicyxfeu")
public suspend fun trafficPercentStrategy(`value`: TrafficPercentStrategyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.trafficPercentStrategy = mapped
}
/**
* @param argument Google Service Control selects service configurations based on traffic percentage.
*/
@JvmName("rlexglojluiqskkg")
public suspend fun trafficPercentStrategy(argument: suspend TrafficPercentStrategyArgsBuilder.() -> Unit) {
val toBeMapped = TrafficPercentStrategyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.trafficPercentStrategy = mapped
}
internal fun build(): RolloutArgs = RolloutArgs(
createTime = createTime,
createdBy = createdBy,
deleteServiceStrategy = deleteServiceStrategy,
rolloutId = rolloutId,
serviceName = serviceName,
trafficPercentStrategy = trafficPercentStrategy,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy