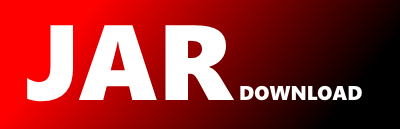
com.pulumi.googlenative.servicemanagement.v1.kotlin.Servicemanagement_v1Functions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.servicemanagement.v1.kotlin
import com.pulumi.googlenative.servicemanagement.v1.Servicemanagement_v1Functions.getConfigPlain
import com.pulumi.googlenative.servicemanagement.v1.Servicemanagement_v1Functions.getRolloutPlain
import com.pulumi.googlenative.servicemanagement.v1.Servicemanagement_v1Functions.getServiceConsumerIamPolicyPlain
import com.pulumi.googlenative.servicemanagement.v1.Servicemanagement_v1Functions.getServiceIamPolicyPlain
import com.pulumi.googlenative.servicemanagement.v1.Servicemanagement_v1Functions.getServicePlain
import com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.GetConfigPlainArgs
import com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.GetConfigPlainArgsBuilder
import com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.GetRolloutPlainArgs
import com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.GetRolloutPlainArgsBuilder
import com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.GetServiceConsumerIamPolicyPlainArgs
import com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.GetServiceConsumerIamPolicyPlainArgsBuilder
import com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.GetServiceIamPolicyPlainArgs
import com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.GetServiceIamPolicyPlainArgsBuilder
import com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.GetServicePlainArgs
import com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.GetServicePlainArgsBuilder
import com.pulumi.googlenative.servicemanagement.v1.kotlin.outputs.GetConfigResult
import com.pulumi.googlenative.servicemanagement.v1.kotlin.outputs.GetRolloutResult
import com.pulumi.googlenative.servicemanagement.v1.kotlin.outputs.GetServiceConsumerIamPolicyResult
import com.pulumi.googlenative.servicemanagement.v1.kotlin.outputs.GetServiceIamPolicyResult
import com.pulumi.googlenative.servicemanagement.v1.kotlin.outputs.GetServiceResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.googlenative.servicemanagement.v1.kotlin.outputs.GetConfigResult.Companion.toKotlin as getConfigResultToKotlin
import com.pulumi.googlenative.servicemanagement.v1.kotlin.outputs.GetRolloutResult.Companion.toKotlin as getRolloutResultToKotlin
import com.pulumi.googlenative.servicemanagement.v1.kotlin.outputs.GetServiceConsumerIamPolicyResult.Companion.toKotlin as getServiceConsumerIamPolicyResultToKotlin
import com.pulumi.googlenative.servicemanagement.v1.kotlin.outputs.GetServiceIamPolicyResult.Companion.toKotlin as getServiceIamPolicyResultToKotlin
import com.pulumi.googlenative.servicemanagement.v1.kotlin.outputs.GetServiceResult.Companion.toKotlin as getServiceResultToKotlin
public object Servicemanagement_v1Functions {
/**
* Gets a service configuration (version) for a managed service.
* @param argument null
* @return null
*/
public suspend fun getConfig(argument: GetConfigPlainArgs): GetConfigResult =
getConfigResultToKotlin(getConfigPlain(argument.toJava()).await())
/**
* @see [getConfig].
* @param configId
* @param serviceName
* @param view
* @return null
*/
public suspend fun getConfig(
configId: String,
serviceName: String,
view: String? = null,
): GetConfigResult {
val argument = GetConfigPlainArgs(
configId = configId,
serviceName = serviceName,
view = view,
)
return getConfigResultToKotlin(getConfigPlain(argument.toJava()).await())
}
/**
* @see [getConfig].
* @param argument Builder for [com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.GetConfigPlainArgs].
* @return null
*/
public suspend fun getConfig(argument: suspend GetConfigPlainArgsBuilder.() -> Unit): GetConfigResult {
val builder = GetConfigPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getConfigResultToKotlin(getConfigPlain(builtArgument.toJava()).await())
}
/**
* Gets a service configuration rollout.
* @param argument null
* @return null
*/
public suspend fun getRollout(argument: GetRolloutPlainArgs): GetRolloutResult =
getRolloutResultToKotlin(getRolloutPlain(argument.toJava()).await())
/**
* @see [getRollout].
* @param rolloutId
* @param serviceName
* @return null
*/
public suspend fun getRollout(rolloutId: String, serviceName: String): GetRolloutResult {
val argument = GetRolloutPlainArgs(
rolloutId = rolloutId,
serviceName = serviceName,
)
return getRolloutResultToKotlin(getRolloutPlain(argument.toJava()).await())
}
/**
* @see [getRollout].
* @param argument Builder for [com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.GetRolloutPlainArgs].
* @return null
*/
public suspend fun getRollout(argument: suspend GetRolloutPlainArgsBuilder.() -> Unit): GetRolloutResult {
val builder = GetRolloutPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getRolloutResultToKotlin(getRolloutPlain(builtArgument.toJava()).await())
}
/**
* Gets a managed service. Authentication is required unless the service is public.
* @param argument null
* @return null
*/
public suspend fun getService(argument: GetServicePlainArgs): GetServiceResult =
getServiceResultToKotlin(getServicePlain(argument.toJava()).await())
/**
* @see [getService].
* @param serviceName
* @return null
*/
public suspend fun getService(serviceName: String): GetServiceResult {
val argument = GetServicePlainArgs(
serviceName = serviceName,
)
return getServiceResultToKotlin(getServicePlain(argument.toJava()).await())
}
/**
* @see [getService].
* @param argument Builder for [com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.GetServicePlainArgs].
* @return null
*/
public suspend fun getService(argument: suspend GetServicePlainArgsBuilder.() -> Unit): GetServiceResult {
val builder = GetServicePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getServiceResultToKotlin(getServicePlain(builtArgument.toJava()).await())
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
* @param argument null
* @return null
*/
public suspend fun getServiceConsumerIamPolicy(argument: GetServiceConsumerIamPolicyPlainArgs): GetServiceConsumerIamPolicyResult =
getServiceConsumerIamPolicyResultToKotlin(getServiceConsumerIamPolicyPlain(argument.toJava()).await())
/**
* @see [getServiceConsumerIamPolicy].
* @param consumerId
* @param serviceId
* @return null
*/
public suspend fun getServiceConsumerIamPolicy(consumerId: String, serviceId: String): GetServiceConsumerIamPolicyResult {
val argument = GetServiceConsumerIamPolicyPlainArgs(
consumerId = consumerId,
serviceId = serviceId,
)
return getServiceConsumerIamPolicyResultToKotlin(getServiceConsumerIamPolicyPlain(argument.toJava()).await())
}
/**
* @see [getServiceConsumerIamPolicy].
* @param argument Builder for [com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.GetServiceConsumerIamPolicyPlainArgs].
* @return null
*/
public suspend fun getServiceConsumerIamPolicy(argument: suspend GetServiceConsumerIamPolicyPlainArgsBuilder.() -> Unit): GetServiceConsumerIamPolicyResult {
val builder = GetServiceConsumerIamPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getServiceConsumerIamPolicyResultToKotlin(getServiceConsumerIamPolicyPlain(builtArgument.toJava()).await())
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
* @param argument null
* @return null
*/
public suspend fun getServiceIamPolicy(argument: GetServiceIamPolicyPlainArgs): GetServiceIamPolicyResult =
getServiceIamPolicyResultToKotlin(getServiceIamPolicyPlain(argument.toJava()).await())
/**
* @see [getServiceIamPolicy].
* @param serviceId
* @return null
*/
public suspend fun getServiceIamPolicy(serviceId: String): GetServiceIamPolicyResult {
val argument = GetServiceIamPolicyPlainArgs(
serviceId = serviceId,
)
return getServiceIamPolicyResultToKotlin(getServiceIamPolicyPlain(argument.toJava()).await())
}
/**
* @see [getServiceIamPolicy].
* @param argument Builder for [com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.GetServiceIamPolicyPlainArgs].
* @return null
*/
public suspend fun getServiceIamPolicy(argument: suspend GetServiceIamPolicyPlainArgsBuilder.() -> Unit): GetServiceIamPolicyResult {
val builder = GetServiceIamPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getServiceIamPolicyResultToKotlin(getServiceIamPolicyPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy