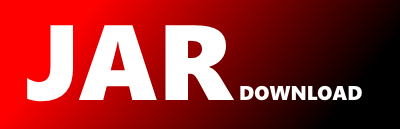
com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs.MethodArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.servicemanagement.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.servicemanagement.v1.inputs.MethodArgs.builder
import com.pulumi.googlenative.servicemanagement.v1.kotlin.enums.MethodSyntax
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Method represents a method of an API interface.
* @property name The simple name of this method.
* @property options Any metadata attached to the method.
* @property requestStreaming If true, the request is streamed.
* @property requestTypeUrl A URL of the input message type.
* @property responseStreaming If true, the response is streamed.
* @property responseTypeUrl The URL of the output message type.
* @property syntax The source syntax of this method.
*/
public data class MethodArgs(
public val name: Output? = null,
public val options: Output>? = null,
public val requestStreaming: Output? = null,
public val requestTypeUrl: Output? = null,
public val responseStreaming: Output? = null,
public val responseTypeUrl: Output? = null,
public val syntax: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.servicemanagement.v1.inputs.MethodArgs =
com.pulumi.googlenative.servicemanagement.v1.inputs.MethodArgs.builder()
.name(name?.applyValue({ args0 -> args0 }))
.options(
options?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.requestStreaming(requestStreaming?.applyValue({ args0 -> args0 }))
.requestTypeUrl(requestTypeUrl?.applyValue({ args0 -> args0 }))
.responseStreaming(responseStreaming?.applyValue({ args0 -> args0 }))
.responseTypeUrl(responseTypeUrl?.applyValue({ args0 -> args0 }))
.syntax(syntax?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [MethodArgs].
*/
@PulumiTagMarker
public class MethodArgsBuilder internal constructor() {
private var name: Output? = null
private var options: Output>? = null
private var requestStreaming: Output? = null
private var requestTypeUrl: Output? = null
private var responseStreaming: Output? = null
private var responseTypeUrl: Output? = null
private var syntax: Output? = null
/**
* @param value The simple name of this method.
*/
@JvmName("kbyyneokyqgnflik")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Any metadata attached to the method.
*/
@JvmName("kapkhfylymlfryjg")
public suspend fun options(`value`: Output>) {
this.options = value
}
@JvmName("khsxgldxjxpimtif")
public suspend fun options(vararg values: Output) {
this.options = Output.all(values.asList())
}
/**
* @param values Any metadata attached to the method.
*/
@JvmName("ibcufunwnlxuobsy")
public suspend fun options(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy