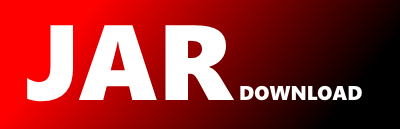
com.pulumi.googlenative.spanner.v1.kotlin.BackupArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.spanner.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.spanner.v1.BackupArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Starts creating a new Cloud Spanner Backup. The returned backup long-running operation will have a name of the format `projects//instances//backups//operations/` and can be used to track creation of the backup. The metadata field type is CreateBackupMetadata. The response field type is Backup, if successful. Cancelling the returned operation will stop the creation and delete the backup. There can be only one pending backup creation per database. Backup creation of different databases can run concurrently.
* @property backupId Required. The id of the backup to be created. The `backup_id` appended to `parent` forms the full backup name of the form `projects//instances//backups/`.
* @property database Required for the CreateBackup operation. Name of the database from which this backup was created. This needs to be in the same instance as the backup. Values are of the form `projects//instances//databases/`.
* @property encryptionConfigEncryptionType Required. The encryption type of the backup.
* @property encryptionConfigKmsKeyName Optional. The Cloud KMS key that will be used to protect the backup. This field should be set only when encryption_type is `CUSTOMER_MANAGED_ENCRYPTION`. Values are of the form `projects//locations//keyRings//cryptoKeys/`.
* @property expireTime Required for the CreateBackup operation. The expiration time of the backup, with microseconds granularity that must be at least 6 hours and at most 366 days from the time the CreateBackup request is processed. Once the `expire_time` has passed, the backup is eligible to be automatically deleted by Cloud Spanner to free the resources used by the backup.
* @property instanceId
* @property name Output only for the CreateBackup operation. Required for the UpdateBackup operation. A globally unique identifier for the backup which cannot be changed. Values are of the form `projects//instances//backups/a-z*[a-z0-9]` The final segment of the name must be between 2 and 60 characters in length. The backup is stored in the location(s) specified in the instance configuration of the instance containing the backup, identified by the prefix of the backup name of the form `projects//instances/`.
* @property project
* @property versionTime The backup will contain an externally consistent copy of the database at the timestamp specified by `version_time`. If `version_time` is not specified, the system will set `version_time` to the `create_time` of the backup.
*/
public data class BackupArgs(
public val backupId: Output? = null,
public val database: Output? = null,
public val encryptionConfigEncryptionType: Output? = null,
public val encryptionConfigKmsKeyName: Output? = null,
public val expireTime: Output? = null,
public val instanceId: Output? = null,
public val name: Output? = null,
public val project: Output? = null,
public val versionTime: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.spanner.v1.BackupArgs =
com.pulumi.googlenative.spanner.v1.BackupArgs.builder()
.backupId(backupId?.applyValue({ args0 -> args0 }))
.database(database?.applyValue({ args0 -> args0 }))
.encryptionConfigEncryptionType(encryptionConfigEncryptionType?.applyValue({ args0 -> args0 }))
.encryptionConfigKmsKeyName(encryptionConfigKmsKeyName?.applyValue({ args0 -> args0 }))
.expireTime(expireTime?.applyValue({ args0 -> args0 }))
.instanceId(instanceId?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.versionTime(versionTime?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BackupArgs].
*/
@PulumiTagMarker
public class BackupArgsBuilder internal constructor() {
private var backupId: Output? = null
private var database: Output? = null
private var encryptionConfigEncryptionType: Output? = null
private var encryptionConfigKmsKeyName: Output? = null
private var expireTime: Output? = null
private var instanceId: Output? = null
private var name: Output? = null
private var project: Output? = null
private var versionTime: Output? = null
/**
* @param value Required. The id of the backup to be created. The `backup_id` appended to `parent` forms the full backup name of the form `projects//instances//backups/`.
*/
@JvmName("wsbwxxwuaefxovje")
public suspend fun backupId(`value`: Output) {
this.backupId = value
}
/**
* @param value Required for the CreateBackup operation. Name of the database from which this backup was created. This needs to be in the same instance as the backup. Values are of the form `projects//instances//databases/`.
*/
@JvmName("teamckyeuyxvsnrl")
public suspend fun database(`value`: Output) {
this.database = value
}
/**
* @param value Required. The encryption type of the backup.
*/
@JvmName("nkkquudnhtqhatph")
public suspend fun encryptionConfigEncryptionType(`value`: Output) {
this.encryptionConfigEncryptionType = value
}
/**
* @param value Optional. The Cloud KMS key that will be used to protect the backup. This field should be set only when encryption_type is `CUSTOMER_MANAGED_ENCRYPTION`. Values are of the form `projects//locations//keyRings//cryptoKeys/`.
*/
@JvmName("htquhxgrhutxacud")
public suspend fun encryptionConfigKmsKeyName(`value`: Output) {
this.encryptionConfigKmsKeyName = value
}
/**
* @param value Required for the CreateBackup operation. The expiration time of the backup, with microseconds granularity that must be at least 6 hours and at most 366 days from the time the CreateBackup request is processed. Once the `expire_time` has passed, the backup is eligible to be automatically deleted by Cloud Spanner to free the resources used by the backup.
*/
@JvmName("epbasbesgbxinltt")
public suspend fun expireTime(`value`: Output) {
this.expireTime = value
}
/**
* @param value
*/
@JvmName("xfkmxensovsxqcnv")
public suspend fun instanceId(`value`: Output) {
this.instanceId = value
}
/**
* @param value Output only for the CreateBackup operation. Required for the UpdateBackup operation. A globally unique identifier for the backup which cannot be changed. Values are of the form `projects//instances//backups/a-z*[a-z0-9]` The final segment of the name must be between 2 and 60 characters in length. The backup is stored in the location(s) specified in the instance configuration of the instance containing the backup, identified by the prefix of the backup name of the form `projects//instances/`.
*/
@JvmName("ykjfyvvcbimunbtf")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value
*/
@JvmName("inocjvciyiirxbsg")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value The backup will contain an externally consistent copy of the database at the timestamp specified by `version_time`. If `version_time` is not specified, the system will set `version_time` to the `create_time` of the backup.
*/
@JvmName("ulqqufqiafhjkcsv")
public suspend fun versionTime(`value`: Output) {
this.versionTime = value
}
/**
* @param value Required. The id of the backup to be created. The `backup_id` appended to `parent` forms the full backup name of the form `projects//instances//backups/`.
*/
@JvmName("nekuxfmmxqxeqgjp")
public suspend fun backupId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backupId = mapped
}
/**
* @param value Required for the CreateBackup operation. Name of the database from which this backup was created. This needs to be in the same instance as the backup. Values are of the form `projects//instances//databases/`.
*/
@JvmName("rflylpaegtcbkrbo")
public suspend fun database(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.database = mapped
}
/**
* @param value Required. The encryption type of the backup.
*/
@JvmName("hlvadexywjfyivaa")
public suspend fun encryptionConfigEncryptionType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryptionConfigEncryptionType = mapped
}
/**
* @param value Optional. The Cloud KMS key that will be used to protect the backup. This field should be set only when encryption_type is `CUSTOMER_MANAGED_ENCRYPTION`. Values are of the form `projects//locations//keyRings//cryptoKeys/`.
*/
@JvmName("vbvqgejhwqcfcdbe")
public suspend fun encryptionConfigKmsKeyName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryptionConfigKmsKeyName = mapped
}
/**
* @param value Required for the CreateBackup operation. The expiration time of the backup, with microseconds granularity that must be at least 6 hours and at most 366 days from the time the CreateBackup request is processed. Once the `expire_time` has passed, the backup is eligible to be automatically deleted by Cloud Spanner to free the resources used by the backup.
*/
@JvmName("potssvjbxxtcpbem")
public suspend fun expireTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.expireTime = mapped
}
/**
* @param value
*/
@JvmName("tnppbjvfglmtfcgn")
public suspend fun instanceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.instanceId = mapped
}
/**
* @param value Output only for the CreateBackup operation. Required for the UpdateBackup operation. A globally unique identifier for the backup which cannot be changed. Values are of the form `projects//instances//backups/a-z*[a-z0-9]` The final segment of the name must be between 2 and 60 characters in length. The backup is stored in the location(s) specified in the instance configuration of the instance containing the backup, identified by the prefix of the backup name of the form `projects//instances/`.
*/
@JvmName("pmpqytoxfxhglylu")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value
*/
@JvmName("pmjjnvcnhsfigaoc")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value The backup will contain an externally consistent copy of the database at the timestamp specified by `version_time`. If `version_time` is not specified, the system will set `version_time` to the `create_time` of the backup.
*/
@JvmName("iopobtabylcxniuq")
public suspend fun versionTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.versionTime = mapped
}
internal fun build(): BackupArgs = BackupArgs(
backupId = backupId,
database = database,
encryptionConfigEncryptionType = encryptionConfigEncryptionType,
encryptionConfigKmsKeyName = encryptionConfigKmsKeyName,
expireTime = expireTime,
instanceId = instanceId,
name = name,
project = project,
versionTime = versionTime,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy