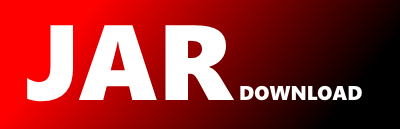
com.pulumi.googlenative.speech.v1.kotlin.CustomClassArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.speech.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.speech.v1.CustomClassArgs.builder
import com.pulumi.googlenative.speech.v1.kotlin.inputs.ClassItemArgs
import com.pulumi.googlenative.speech.v1.kotlin.inputs.ClassItemArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Create a custom class.
* @property customClassId The ID to use for the custom class, which will become the final component of the custom class' resource name. This value should restrict to letters, numbers, and hyphens, with the first character a letter, the last a letter or a number, and be 4-63 characters.
* @property items A collection of class items.
* @property location
* @property name The resource name of the custom class.
* @property project
*/
public data class CustomClassArgs(
public val customClassId: Output? = null,
public val items: Output>? = null,
public val location: Output? = null,
public val name: Output? = null,
public val project: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.speech.v1.CustomClassArgs =
com.pulumi.googlenative.speech.v1.CustomClassArgs.builder()
.customClassId(customClassId?.applyValue({ args0 -> args0 }))
.items(items?.applyValue({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.location(location?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CustomClassArgs].
*/
@PulumiTagMarker
public class CustomClassArgsBuilder internal constructor() {
private var customClassId: Output? = null
private var items: Output>? = null
private var location: Output? = null
private var name: Output? = null
private var project: Output? = null
/**
* @param value The ID to use for the custom class, which will become the final component of the custom class' resource name. This value should restrict to letters, numbers, and hyphens, with the first character a letter, the last a letter or a number, and be 4-63 characters.
*/
@JvmName("hknlscouigotmdlw")
public suspend fun customClassId(`value`: Output) {
this.customClassId = value
}
/**
* @param value A collection of class items.
*/
@JvmName("ddmqmkqbrmbqvmvr")
public suspend fun items(`value`: Output>) {
this.items = value
}
@JvmName("dtiedyokpbrgnuyp")
public suspend fun items(vararg values: Output) {
this.items = Output.all(values.asList())
}
/**
* @param values A collection of class items.
*/
@JvmName("efjcdufdejvdgnvc")
public suspend fun items(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy