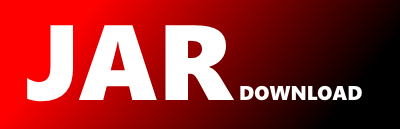
com.pulumi.googlenative.sqladmin.v1.kotlin.Instance.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.sqladmin.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.DiskEncryptionConfigurationResponse
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.DiskEncryptionStatusResponse
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.InstanceFailoverReplicaResponse
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.IpMappingResponse
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.OnPremisesConfigurationResponse
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.ReplicaConfigurationResponse
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.SettingsResponse
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.SqlOutOfDiskReportResponse
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.SqlScheduledMaintenanceResponse
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.SslCertResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.DiskEncryptionConfigurationResponse.Companion.toKotlin as diskEncryptionConfigurationResponseToKotlin
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.DiskEncryptionStatusResponse.Companion.toKotlin as diskEncryptionStatusResponseToKotlin
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.InstanceFailoverReplicaResponse.Companion.toKotlin as instanceFailoverReplicaResponseToKotlin
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.IpMappingResponse.Companion.toKotlin as ipMappingResponseToKotlin
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.OnPremisesConfigurationResponse.Companion.toKotlin as onPremisesConfigurationResponseToKotlin
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.ReplicaConfigurationResponse.Companion.toKotlin as replicaConfigurationResponseToKotlin
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.SettingsResponse.Companion.toKotlin as settingsResponseToKotlin
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.SqlOutOfDiskReportResponse.Companion.toKotlin as sqlOutOfDiskReportResponseToKotlin
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.SqlScheduledMaintenanceResponse.Companion.toKotlin as sqlScheduledMaintenanceResponseToKotlin
import com.pulumi.googlenative.sqladmin.v1.kotlin.outputs.SslCertResponse.Companion.toKotlin as sslCertResponseToKotlin
/**
* Builder for [Instance].
*/
@PulumiTagMarker
public class InstanceResourceBuilder internal constructor() {
public var name: String? = null
public var args: InstanceArgs = InstanceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend InstanceArgsBuilder.() -> Unit) {
val builder = InstanceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Instance {
val builtJavaResource = com.pulumi.googlenative.sqladmin.v1.Instance(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Instance(builtJavaResource)
}
}
/**
* Creates a new Cloud SQL instance.
*/
public class Instance internal constructor(
override val javaResource: com.pulumi.googlenative.sqladmin.v1.Instance,
) : KotlinCustomResource(javaResource, InstanceMapper) {
/**
* List all maintenance versions applicable on the instance
*/
public val availableMaintenanceVersions: Output>
get() = javaResource.availableMaintenanceVersions().applyValue({ args0 ->
args0.map({ args0 ->
args0
})
})
/**
* The backend type. `SECOND_GEN`: Cloud SQL database instance. `EXTERNAL`: A database server that is not managed by Google. This property is read-only; use the `tier` property in the `settings` object to determine the database type.
*/
public val backendType: Output
get() = javaResource.backendType().applyValue({ args0 -> args0 })
/**
* Connection name of the Cloud SQL instance used in connection strings.
*/
public val connectionName: Output
get() = javaResource.connectionName().applyValue({ args0 -> args0 })
/**
* The time when the instance was created in [RFC 3339](https://tools.ietf.org/html/rfc3339) format, for example `2012-11-15T16:19:00.094Z`.
*/
public val createTime: Output
get() = javaResource.createTime().applyValue({ args0 -> args0 })
/**
* The current disk usage of the instance in bytes. This property has been deprecated. Use the "cloudsql.googleapis.com/database/disk/bytes_used" metric in Cloud Monitoring API instead. Please see [this announcement](https://groups.google.com/d/msg/google-cloud-sql-announce/I_7-F9EBhT0/BtvFtdFeAgAJ) for details.
*/
public val currentDiskSize: Output
get() = javaResource.currentDiskSize().applyValue({ args0 -> args0 })
/**
* Stores the current database version running on the instance including minor version such as `MYSQL_8_0_18`.
*/
public val databaseInstalledVersion: Output
get() = javaResource.databaseInstalledVersion().applyValue({ args0 -> args0 })
/**
* The database engine type and version. The `databaseVersion` field cannot be changed after instance creation.
*/
public val databaseVersion: Output
get() = javaResource.databaseVersion().applyValue({ args0 -> args0 })
/**
* Disk encryption configuration specific to an instance.
*/
public val diskEncryptionConfiguration: Output
get() = javaResource.diskEncryptionConfiguration().applyValue({ args0 ->
args0.let({ args0 ->
diskEncryptionConfigurationResponseToKotlin(args0)
})
})
/**
* Disk encryption status specific to an instance.
*/
public val diskEncryptionStatus: Output
get() = javaResource.diskEncryptionStatus().applyValue({ args0 ->
args0.let({ args0 ->
diskEncryptionStatusResponseToKotlin(args0)
})
})
/**
* This field is deprecated and will be removed from a future version of the API. Use the `settings.settingsVersion` field instead.
*/
@Deprecated(
message = """
This field is deprecated and will be removed from a future version of the API. Use the
`settings.settingsVersion` field instead.
""",
)
public val etag: Output
get() = javaResource.etag().applyValue({ args0 -> args0 })
/**
* The name and status of the failover replica.
*/
public val failoverReplica: Output
get() = javaResource.failoverReplica().applyValue({ args0 ->
args0.let({ args0 ->
instanceFailoverReplicaResponseToKotlin(args0)
})
})
/**
* The Compute Engine zone that the instance is currently serving from. This value could be different from the zone that was specified when the instance was created if the instance has failed over to its secondary zone. WARNING: Changing this might restart the instance.
*/
public val gceZone: Output
get() = javaResource.gceZone().applyValue({ args0 -> args0 })
/**
* The instance type.
*/
public val instanceType: Output
get() = javaResource.instanceType().applyValue({ args0 -> args0 })
/**
* The assigned IP addresses for the instance.
*/
public val ipAddresses: Output>
get() = javaResource.ipAddresses().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
ipMappingResponseToKotlin(args0)
})
})
})
/**
* The IPv6 address assigned to the instance. (Deprecated) This property was applicable only to First Generation instances.
*/
@Deprecated(
message = """
The IPv6 address assigned to the instance. (Deprecated) This property was applicable only to First
Generation instances.
""",
)
public val ipv6Address: Output
get() = javaResource.ipv6Address().applyValue({ args0 -> args0 })
/**
* This is always `sql#instance`.
*/
public val kind: Output
get() = javaResource.kind().applyValue({ args0 -> args0 })
/**
* The current software version on the instance.
*/
public val maintenanceVersion: Output
get() = javaResource.maintenanceVersion().applyValue({ args0 -> args0 })
/**
* The name of the instance which will act as primary in the replication setup.
*/
public val masterInstanceName: Output
get() = javaResource.masterInstanceName().applyValue({ args0 -> args0 })
/**
* The maximum disk size of the instance in bytes.
*/
public val maxDiskSize: Output
get() = javaResource.maxDiskSize().applyValue({ args0 -> args0 })
/**
* Name of the Cloud SQL instance. This does not include the project ID.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Configuration specific to on-premises instances.
*/
public val onPremisesConfiguration: Output
get() = javaResource.onPremisesConfiguration().applyValue({ args0 ->
args0.let({ args0 ->
onPremisesConfigurationResponseToKotlin(args0)
})
})
/**
* This field represents the report generated by the proactive database wellness job for OutOfDisk issues. * Writers: * the proactive database wellness job for OOD. * Readers: * the proactive database wellness job
*/
public val outOfDiskReport: Output
get() = javaResource.outOfDiskReport().applyValue({ args0 ->
args0.let({ args0 ->
sqlOutOfDiskReportResponseToKotlin(args0)
})
})
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* The geographical region. Can be: * `us-central` (`FIRST_GEN` instances only) * `us-central1` (`SECOND_GEN` instances only) * `asia-east1` or `europe-west1`. Defaults to `us-central` or `us-central1` depending on the instance type. The region cannot be changed after instance creation.
*/
public val region: Output
get() = javaResource.region().applyValue({ args0 -> args0 })
/**
* Configuration specific to failover replicas and read replicas.
*/
public val replicaConfiguration: Output
get() = javaResource.replicaConfiguration().applyValue({ args0 ->
args0.let({ args0 ->
replicaConfigurationResponseToKotlin(args0)
})
})
/**
* The replicas of the instance.
*/
public val replicaNames: Output>
get() = javaResource.replicaNames().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* Initial root password. Use only on creation. You must set root passwords before you can connect to PostgreSQL instances.
*/
public val rootPassword: Output
get() = javaResource.rootPassword().applyValue({ args0 -> args0 })
/**
* The status indicating if instance satisfiesPzs. Reserved for future use.
*/
public val satisfiesPzs: Output
get() = javaResource.satisfiesPzs().applyValue({ args0 -> args0 })
/**
* The start time of any upcoming scheduled maintenance for this instance.
*/
public val scheduledMaintenance: Output
get() = javaResource.scheduledMaintenance().applyValue({ args0 ->
args0.let({ args0 ->
sqlScheduledMaintenanceResponseToKotlin(args0)
})
})
/**
* The Compute Engine zone that the failover instance is currently serving from for a regional instance. This value could be different from the zone that was specified when the instance was created if the instance has failed over to its secondary/failover zone.
*/
public val secondaryGceZone: Output
get() = javaResource.secondaryGceZone().applyValue({ args0 -> args0 })
/**
* The URI of this resource.
*/
public val selfLink: Output
get() = javaResource.selfLink().applyValue({ args0 -> args0 })
/**
* SSL configuration.
*/
public val serverCaCert: Output
get() = javaResource.serverCaCert().applyValue({ args0 ->
args0.let({ args0 ->
sslCertResponseToKotlin(args0)
})
})
/**
* The service account email address assigned to the instance.\This property is read-only.
*/
public val serviceAccountEmailAddress: Output
get() = javaResource.serviceAccountEmailAddress().applyValue({ args0 -> args0 })
/**
* The user settings.
*/
public val settings: Output
get() = javaResource.settings().applyValue({ args0 ->
args0.let({ args0 ->
settingsResponseToKotlin(args0)
})
})
/**
* The current serving state of the Cloud SQL instance.
*/
public val state: Output
get() = javaResource.state().applyValue({ args0 -> args0 })
/**
* If the instance state is SUSPENDED, the reason for the suspension.
*/
public val suspensionReason: Output>
get() = javaResource.suspensionReason().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
}
public object InstanceMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.googlenative.sqladmin.v1.Instance::class == javaResource::class
override fun map(javaResource: Resource): Instance = Instance(
javaResource as
com.pulumi.googlenative.sqladmin.v1.Instance,
)
}
/**
* @see [Instance].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Instance].
*/
public suspend fun instance(name: String, block: suspend InstanceResourceBuilder.() -> Unit): Instance {
val builder = InstanceResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Instance].
* @param name The _unique_ name of the resulting resource.
*/
public fun instance(name: String): Instance {
val builder = InstanceResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy