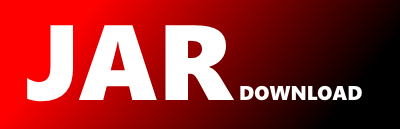
com.pulumi.googlenative.sqladmin.v1.kotlin.inputs.ReplicaConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.sqladmin.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.sqladmin.v1.inputs.ReplicaConfigurationArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Read-replica configuration for connecting to the primary instance.
* @property failoverTarget Specifies if the replica is the failover target. If the field is set to `true`, the replica will be designated as a failover replica. In case the primary instance fails, the replica instance will be promoted as the new primary instance. Only one replica can be specified as failover target, and the replica has to be in different zone with the primary instance.
* @property kind This is always `sql#replicaConfiguration`.
* @property mysqlReplicaConfiguration MySQL specific configuration when replicating from a MySQL on-premises primary instance. Replication configuration information such as the username, password, certificates, and keys are not stored in the instance metadata. The configuration information is used only to set up the replication connection and is stored by MySQL in a file named `master.info` in the data directory.
*/
public data class ReplicaConfigurationArgs(
public val failoverTarget: Output? = null,
public val kind: Output? = null,
public val mysqlReplicaConfiguration: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.sqladmin.v1.inputs.ReplicaConfigurationArgs =
com.pulumi.googlenative.sqladmin.v1.inputs.ReplicaConfigurationArgs.builder()
.failoverTarget(failoverTarget?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 }))
.mysqlReplicaConfiguration(
mysqlReplicaConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [ReplicaConfigurationArgs].
*/
@PulumiTagMarker
public class ReplicaConfigurationArgsBuilder internal constructor() {
private var failoverTarget: Output? = null
private var kind: Output? = null
private var mysqlReplicaConfiguration: Output? = null
/**
* @param value Specifies if the replica is the failover target. If the field is set to `true`, the replica will be designated as a failover replica. In case the primary instance fails, the replica instance will be promoted as the new primary instance. Only one replica can be specified as failover target, and the replica has to be in different zone with the primary instance.
*/
@JvmName("nfpevqftqynfynoj")
public suspend fun failoverTarget(`value`: Output) {
this.failoverTarget = value
}
/**
* @param value This is always `sql#replicaConfiguration`.
*/
@JvmName("dhiumscuidtbmmoe")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value MySQL specific configuration when replicating from a MySQL on-premises primary instance. Replication configuration information such as the username, password, certificates, and keys are not stored in the instance metadata. The configuration information is used only to set up the replication connection and is stored by MySQL in a file named `master.info` in the data directory.
*/
@JvmName("vibjaalwjvkwnlka")
public suspend fun mysqlReplicaConfiguration(`value`: Output) {
this.mysqlReplicaConfiguration = value
}
/**
* @param value Specifies if the replica is the failover target. If the field is set to `true`, the replica will be designated as a failover replica. In case the primary instance fails, the replica instance will be promoted as the new primary instance. Only one replica can be specified as failover target, and the replica has to be in different zone with the primary instance.
*/
@JvmName("eenqtbupuljaebmw")
public suspend fun failoverTarget(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failoverTarget = mapped
}
/**
* @param value This is always `sql#replicaConfiguration`.
*/
@JvmName("lvilswrswrpoxlhw")
public suspend fun kind(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value MySQL specific configuration when replicating from a MySQL on-premises primary instance. Replication configuration information such as the username, password, certificates, and keys are not stored in the instance metadata. The configuration information is used only to set up the replication connection and is stored by MySQL in a file named `master.info` in the data directory.
*/
@JvmName("kukmdrvfroncinen")
public suspend fun mysqlReplicaConfiguration(`value`: MySqlReplicaConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mysqlReplicaConfiguration = mapped
}
/**
* @param argument MySQL specific configuration when replicating from a MySQL on-premises primary instance. Replication configuration information such as the username, password, certificates, and keys are not stored in the instance metadata. The configuration information is used only to set up the replication connection and is stored by MySQL in a file named `master.info` in the data directory.
*/
@JvmName("qtlvqonqixfsjisf")
public suspend fun mysqlReplicaConfiguration(argument: suspend MySqlReplicaConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = MySqlReplicaConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.mysqlReplicaConfiguration = mapped
}
internal fun build(): ReplicaConfigurationArgs = ReplicaConfigurationArgs(
failoverTarget = failoverTarget,
kind = kind,
mysqlReplicaConfiguration = mysqlReplicaConfiguration,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy