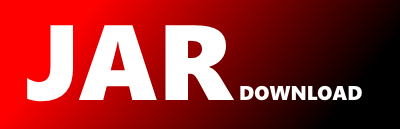
com.pulumi.googlenative.sqladmin.v1beta4.kotlin.UserArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.sqladmin.v1beta4.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.sqladmin.v1beta4.UserArgs.builder
import com.pulumi.googlenative.sqladmin.v1beta4.kotlin.enums.UserDualPasswordType
import com.pulumi.googlenative.sqladmin.v1beta4.kotlin.enums.UserType
import com.pulumi.googlenative.sqladmin.v1beta4.kotlin.inputs.SqlServerUserDetailsArgs
import com.pulumi.googlenative.sqladmin.v1beta4.kotlin.inputs.SqlServerUserDetailsArgsBuilder
import com.pulumi.googlenative.sqladmin.v1beta4.kotlin.inputs.UserPasswordValidationPolicyArgs
import com.pulumi.googlenative.sqladmin.v1beta4.kotlin.inputs.UserPasswordValidationPolicyArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Creates a new user in a Cloud SQL instance.
* @property dualPasswordType Dual password status for the user.
* @property etag This field is deprecated and will be removed from a future version of the API.
* @property host Optional. The host from which the user can connect. For `insert` operations, host defaults to an empty string. For `update` operations, host is specified as part of the request URL. The host name cannot be updated after insertion. For a MySQL instance, it's required; for a PostgreSQL or SQL Server instance, it's optional.
* @property instance The name of the Cloud SQL instance. This does not include the project ID. Can be omitted for *update* because it is already specified on the URL.
* @property kind This is always `sql#user`.
* @property name The name of the user in the Cloud SQL instance. Can be omitted for `update` because it is already specified in the URL.
* @property password The password for the user.
* @property passwordPolicy User level password validation policy.
* @property project The project ID of the project containing the Cloud SQL database. The Google apps domain is prefixed if applicable. Can be omitted for *update* because it is already specified on the URL.
* @property sqlserverUserDetails
* @property type The user type. It determines the method to authenticate the user during login. The default is the database's built-in user type.
*/
public data class UserArgs(
public val dualPasswordType: Output? = null,
@Deprecated(
message = """
This field is deprecated and will be removed from a future version of the API.
""",
)
public val etag: Output? = null,
public val host: Output? = null,
public val instance: Output? = null,
public val kind: Output? = null,
public val name: Output? = null,
public val password: Output? = null,
public val passwordPolicy: Output? = null,
public val project: Output? = null,
public val sqlserverUserDetails: Output? = null,
public val type: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.sqladmin.v1beta4.UserArgs =
com.pulumi.googlenative.sqladmin.v1beta4.UserArgs.builder()
.dualPasswordType(dualPasswordType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.etag(etag?.applyValue({ args0 -> args0 }))
.host(host?.applyValue({ args0 -> args0 }))
.instance(instance?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.password(password?.applyValue({ args0 -> args0 }))
.passwordPolicy(passwordPolicy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.project(project?.applyValue({ args0 -> args0 }))
.sqlserverUserDetails(
sqlserverUserDetails?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.type(type?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [UserArgs].
*/
@PulumiTagMarker
public class UserArgsBuilder internal constructor() {
private var dualPasswordType: Output? = null
private var etag: Output? = null
private var host: Output? = null
private var instance: Output? = null
private var kind: Output? = null
private var name: Output? = null
private var password: Output? = null
private var passwordPolicy: Output? = null
private var project: Output? = null
private var sqlserverUserDetails: Output? = null
private var type: Output? = null
/**
* @param value Dual password status for the user.
*/
@JvmName("vkpnrcksfmfgowsk")
public suspend fun dualPasswordType(`value`: Output) {
this.dualPasswordType = value
}
/**
* @param value This field is deprecated and will be removed from a future version of the API.
*/
@Deprecated(
message = """
This field is deprecated and will be removed from a future version of the API.
""",
)
@JvmName("uswhmikxqqbbvoux")
public suspend fun etag(`value`: Output) {
this.etag = value
}
/**
* @param value Optional. The host from which the user can connect. For `insert` operations, host defaults to an empty string. For `update` operations, host is specified as part of the request URL. The host name cannot be updated after insertion. For a MySQL instance, it's required; for a PostgreSQL or SQL Server instance, it's optional.
*/
@JvmName("gllldapvybixqsxp")
public suspend fun host(`value`: Output) {
this.host = value
}
/**
* @param value The name of the Cloud SQL instance. This does not include the project ID. Can be omitted for *update* because it is already specified on the URL.
*/
@JvmName("vgjndsawenlbqkfx")
public suspend fun instance(`value`: Output) {
this.instance = value
}
/**
* @param value This is always `sql#user`.
*/
@JvmName("fpvytyspmcdexjec")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value The name of the user in the Cloud SQL instance. Can be omitted for `update` because it is already specified in the URL.
*/
@JvmName("bwujjlknrwwltsio")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The password for the user.
*/
@JvmName("kkcljxskgeumyeha")
public suspend fun password(`value`: Output) {
this.password = value
}
/**
* @param value User level password validation policy.
*/
@JvmName("jpwunyonqxednyvx")
public suspend fun passwordPolicy(`value`: Output) {
this.passwordPolicy = value
}
/**
* @param value The project ID of the project containing the Cloud SQL database. The Google apps domain is prefixed if applicable. Can be omitted for *update* because it is already specified on the URL.
*/
@JvmName("dpasdhtayvynxrto")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value
*/
@JvmName("dhodmkgucsiylulq")
public suspend fun sqlserverUserDetails(`value`: Output) {
this.sqlserverUserDetails = value
}
/**
* @param value The user type. It determines the method to authenticate the user during login. The default is the database's built-in user type.
*/
@JvmName("bqxhosstpkhgudmb")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value Dual password status for the user.
*/
@JvmName("minoedisdqrsorhl")
public suspend fun dualPasswordType(`value`: UserDualPasswordType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dualPasswordType = mapped
}
/**
* @param value This field is deprecated and will be removed from a future version of the API.
*/
@Deprecated(
message = """
This field is deprecated and will be removed from a future version of the API.
""",
)
@JvmName("fewdqtwgqukbsvgy")
public suspend fun etag(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.etag = mapped
}
/**
* @param value Optional. The host from which the user can connect. For `insert` operations, host defaults to an empty string. For `update` operations, host is specified as part of the request URL. The host name cannot be updated after insertion. For a MySQL instance, it's required; for a PostgreSQL or SQL Server instance, it's optional.
*/
@JvmName("pujbscwbnwsdbcxy")
public suspend fun host(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.host = mapped
}
/**
* @param value The name of the Cloud SQL instance. This does not include the project ID. Can be omitted for *update* because it is already specified on the URL.
*/
@JvmName("rpiiehemhxgbumua")
public suspend fun instance(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.instance = mapped
}
/**
* @param value This is always `sql#user`.
*/
@JvmName("tiwuwtqjirmaquxr")
public suspend fun kind(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value The name of the user in the Cloud SQL instance. Can be omitted for `update` because it is already specified in the URL.
*/
@JvmName("dctvtttkdeiceycg")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The password for the user.
*/
@JvmName("ondjsrrsqxnpfkew")
public suspend fun password(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.password = mapped
}
/**
* @param value User level password validation policy.
*/
@JvmName("vyslkdvpwaqoatfq")
public suspend fun passwordPolicy(`value`: UserPasswordValidationPolicyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.passwordPolicy = mapped
}
/**
* @param argument User level password validation policy.
*/
@JvmName("nplixecfjuwtxsni")
public suspend fun passwordPolicy(argument: suspend UserPasswordValidationPolicyArgsBuilder.() -> Unit) {
val toBeMapped = UserPasswordValidationPolicyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.passwordPolicy = mapped
}
/**
* @param value The project ID of the project containing the Cloud SQL database. The Google apps domain is prefixed if applicable. Can be omitted for *update* because it is already specified on the URL.
*/
@JvmName("yfvujihdvpvocwuv")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value
*/
@JvmName("fgrlgsuistxkhtam")
public suspend fun sqlserverUserDetails(`value`: SqlServerUserDetailsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sqlserverUserDetails = mapped
}
/**
* @param argument
*/
@JvmName("sppcwfyvlnjqabad")
public suspend fun sqlserverUserDetails(argument: suspend SqlServerUserDetailsArgsBuilder.() -> Unit) {
val toBeMapped = SqlServerUserDetailsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.sqlserverUserDetails = mapped
}
/**
* @param value The user type. It determines the method to authenticate the user during login. The default is the database's built-in user type.
*/
@JvmName("bpyqjuqveuxubcte")
public suspend fun type(`value`: UserType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): UserArgs = UserArgs(
dualPasswordType = dualPasswordType,
etag = etag,
host = host,
instance = instance,
kind = kind,
name = name,
password = password,
passwordPolicy = passwordPolicy,
project = project,
sqlserverUserDetails = sqlserverUserDetails,
type = type,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy