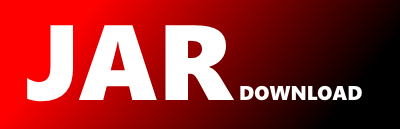
com.pulumi.googlenative.storage.v1.kotlin.Bucket.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.storage.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketAccessControlResponse
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketAutoclassResponse
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketBillingResponse
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketCorsItemResponse
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketCustomPlacementConfigResponse
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketEncryptionResponse
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketIamConfigurationResponse
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketLifecycleResponse
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketLoggingResponse
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketOwnerResponse
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketRetentionPolicyResponse
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketVersioningResponse
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketWebsiteResponse
import com.pulumi.googlenative.storage.v1.kotlin.outputs.ObjectAccessControlResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketAccessControlResponse.Companion.toKotlin as bucketAccessControlResponseToKotlin
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketAutoclassResponse.Companion.toKotlin as bucketAutoclassResponseToKotlin
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketBillingResponse.Companion.toKotlin as bucketBillingResponseToKotlin
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketCorsItemResponse.Companion.toKotlin as bucketCorsItemResponseToKotlin
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketCustomPlacementConfigResponse.Companion.toKotlin as bucketCustomPlacementConfigResponseToKotlin
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketEncryptionResponse.Companion.toKotlin as bucketEncryptionResponseToKotlin
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketIamConfigurationResponse.Companion.toKotlin as bucketIamConfigurationResponseToKotlin
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketLifecycleResponse.Companion.toKotlin as bucketLifecycleResponseToKotlin
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketLoggingResponse.Companion.toKotlin as bucketLoggingResponseToKotlin
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketOwnerResponse.Companion.toKotlin as bucketOwnerResponseToKotlin
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketRetentionPolicyResponse.Companion.toKotlin as bucketRetentionPolicyResponseToKotlin
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketVersioningResponse.Companion.toKotlin as bucketVersioningResponseToKotlin
import com.pulumi.googlenative.storage.v1.kotlin.outputs.BucketWebsiteResponse.Companion.toKotlin as bucketWebsiteResponseToKotlin
import com.pulumi.googlenative.storage.v1.kotlin.outputs.ObjectAccessControlResponse.Companion.toKotlin as objectAccessControlResponseToKotlin
/**
* Builder for [Bucket].
*/
@PulumiTagMarker
public class BucketResourceBuilder internal constructor() {
public var name: String? = null
public var args: BucketArgs = BucketArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend BucketArgsBuilder.() -> Unit) {
val builder = BucketArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Bucket {
val builtJavaResource = com.pulumi.googlenative.storage.v1.Bucket(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Bucket(builtJavaResource)
}
}
/**
* Creates a new bucket.
*/
public class Bucket internal constructor(
override val javaResource: com.pulumi.googlenative.storage.v1.Bucket,
) : KotlinCustomResource(javaResource, BucketMapper) {
/**
* Access controls on the bucket.
*/
public val acl: Output>
get() = javaResource.acl().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
bucketAccessControlResponseToKotlin(args0)
})
})
})
/**
* The bucket's Autoclass configuration.
*/
public val autoclass: Output
get() = javaResource.autoclass().applyValue({ args0 ->
args0.let({ args0 ->
bucketAutoclassResponseToKotlin(args0)
})
})
/**
* The bucket's billing configuration.
*/
public val billing: Output
get() = javaResource.billing().applyValue({ args0 ->
args0.let({ args0 ->
bucketBillingResponseToKotlin(args0)
})
})
/**
* The bucket's Cross-Origin Resource Sharing (CORS) configuration.
*/
public val cors: Output>
get() = javaResource.cors().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
bucketCorsItemResponseToKotlin(args0)
})
})
})
/**
* The bucket's custom placement configuration for Custom Dual Regions.
*/
public val customPlacementConfig: Output
get() = javaResource.customPlacementConfig().applyValue({ args0 ->
args0.let({ args0 ->
bucketCustomPlacementConfigResponseToKotlin(args0)
})
})
/**
* The default value for event-based hold on newly created objects in this bucket. Event-based hold is a way to retain objects indefinitely until an event occurs, signified by the hold's release. After being released, such objects will be subject to bucket-level retention (if any). One sample use case of this flag is for banks to hold loan documents for at least 3 years after loan is paid in full. Here, bucket-level retention is 3 years and the event is loan being paid in full. In this example, these objects will be held intact for any number of years until the event has occurred (event-based hold on the object is released) and then 3 more years after that. That means retention duration of the objects begins from the moment event-based hold transitioned from true to false. Objects under event-based hold cannot be deleted, overwritten or archived until the hold is removed.
*/
public val defaultEventBasedHold: Output
get() = javaResource.defaultEventBasedHold().applyValue({ args0 -> args0 })
/**
* Default access controls to apply to new objects when no ACL is provided.
*/
public val defaultObjectAcl: Output>
get() = javaResource.defaultObjectAcl().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> objectAccessControlResponseToKotlin(args0) })
})
})
/**
* Encryption configuration for a bucket.
*/
public val encryption: Output
get() = javaResource.encryption().applyValue({ args0 ->
args0.let({ args0 ->
bucketEncryptionResponseToKotlin(args0)
})
})
/**
* HTTP 1.1 Entity tag for the bucket.
*/
public val etag: Output
get() = javaResource.etag().applyValue({ args0 -> args0 })
/**
* The bucket's IAM configuration.
*/
public val iamConfiguration: Output
get() = javaResource.iamConfiguration().applyValue({ args0 ->
args0.let({ args0 ->
bucketIamConfigurationResponseToKotlin(args0)
})
})
/**
* The kind of item this is. For buckets, this is always storage#bucket.
*/
public val kind: Output
get() = javaResource.kind().applyValue({ args0 -> args0 })
/**
* User-provided labels, in key/value pairs.
*/
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy